get Text and link (if exists) when users highlight text on screen.
Hi guys, I wonder that how can I get text and the link when users highlight it on screen? I got the text by this func but do not know how to get the link. Can anyone help me?
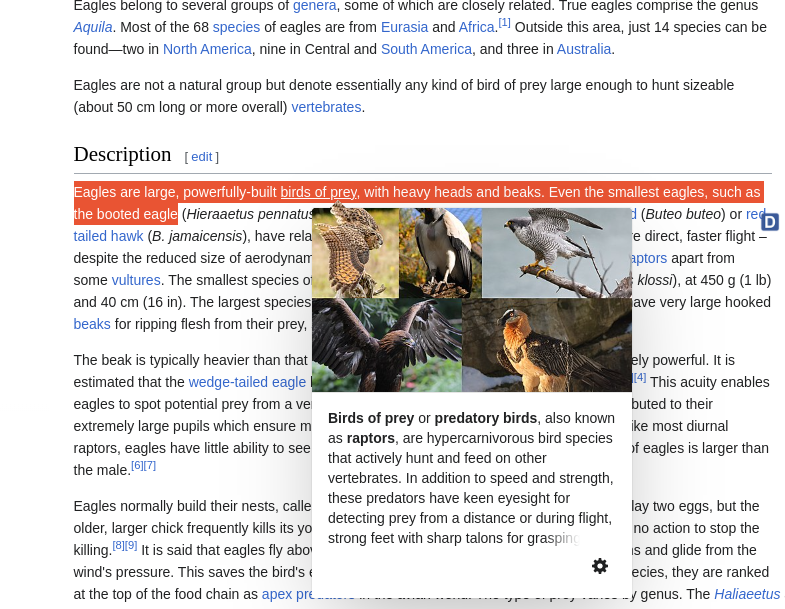
11 Replies
Try this:
(note - I swapped the "var" for "let" and "const" but that is just me being pedantic)
I face an issue "selectedRange.commonAncestorContainer.querySelectorAll is not a function"
That would seem to indicate that selectedRange isn't defined.
Can you share the complete function code?
Here is my test incase you want to compare: https://codepen.io/cbolson/pen/NWmeRBO
ah, OK, the code also needs to check that "something" is selected on mouseup
So I need to check selection and selectedRange are exist before I access commonAncestorContainer right ?
I'm using your code 😅
possibly not the best idea - as you have discovered, if the selected text does not contain an href it throws an error.
Ok. But I wonder that when the else if case oucurs? I have tried but never faced that case.
The "else" is for (old) browsers that don't support window.getSelection(). You could probably remove it unless you need to support IE or similar.
Ok thanks
I got a version of this working, sorry, I forgot to post it here. Work got in the way as usual 😛
How can u check if users are selecting something or not?
Can I check base on "isCollapsed" property?
I'm using your code now. But I face a case that it can not get the link
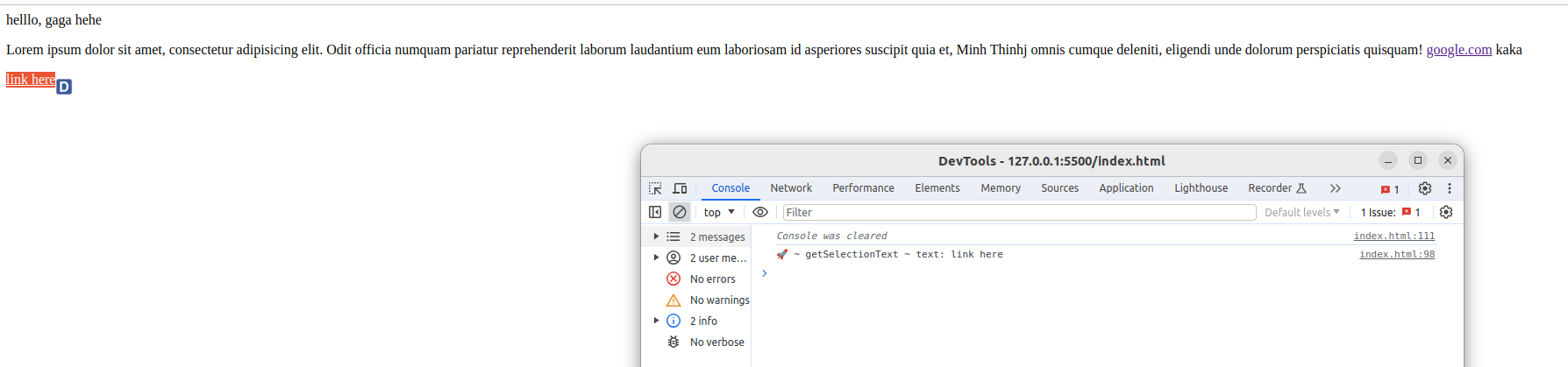