You can use `flex-row` and `flex-wrap`.
You can use
flex-row
and flex-wrap
. Some example code:
import { Dom } from "OneJS/Dom"
import { Tab } from "onejs/comps"
import { h, render } from "preact"
import { Style } from "preact/jsx"
import { Vector2 } from "UnityEngine"
import { useRef } from "preact/hooks"
import { classNames } from "classnames"
import { parseColor as c } from "onejs/utils/color-parser"
import { ScrollViewMode, WheelEvent } from "UnityEngine/UIElements"
interface ExampleTabsProps {
class?: string
style?: Style
}
const exampleTabs = [
{ label: "Tab 1", nums: randInts(200) },
{ label: "Tab 2", nums: randInts(200) },
{ label: "Tab 3", nums: randInts(200) },
{ label: "Tab 4", nums: randInts(200) },
{ label: "Tab 5", nums: randInts(200) },
{ label: "Tab 6", nums: randInts(200) },
{ label: "Tab 7", nums: randInts(200) },
{ label: "Tab 8", nums: randInts(200) },
]
const ExampleTabs = ({ class: classProp, style }: ExampleTabsProps) => {
const refs = Array.from(Array(exampleTabs.length)).map(() => useRef<Dom>())
function onChange(index: number) {
log(`Tabs index changed to ${index}`)
}
// Fixing the UI Toolkit Scrollview speed bug
// https://forum.unity.com/threads/listview-mousewheel-scrolling-speed.1167404/#post-8258421
function onWheel(index: number, evt: WheelEvent) {
let sv = refs[index].current.ve as any
let scroller = sv.verticalScroller
if (scroller.highValue > 0) {
sv.scrollOffset = new Vector2(0, sv.scrollOffset.y + 1000 * evt.delta.y)
evt.StopPropagation()
}
}
return <Tab.Group class={`flex-col w-[700px] ${classProp}`} onChange={onChange} style={style}>
<Tab.List class={`flex-row justify-between rounded-md bg-black/50 p-1 mb-2`}>
{exampleTabs.map((tab, index) =>
<Tab name={tab.label} index={index} class={({ selected }) => (classNames(`flex-row rounded-md text-white/80 items-center bold justify-center p-3 transition-[background-color] duration-200`, selected ? `bg-white active-text-color` : `hover:bg-white/10`))} style={{ width: `${98 / exampleTabs.length}%` }}>{tab.label}</Tab>
)}
</Tab.List>
<Tab.Panels>
{exampleTabs.map((tab, index) =>
<Tab.Panel class={`bg-white rounded-md p-5 flex-row flex-wrap`}>
<scrollview key={`${index}`} class="w-full max-h-[200px]" mode={ScrollViewMode.Vertical}
ref={refs[index]} onWheel={(e) => onWheel(index, e)}>
<div class="w-full flex-row flex-wrap">
{tab.nums.map(num => <div class="m-2 justify-center items-center w-10 h-10 bg-orange-200">{num}</div>)}
</div>
</scrollview>
</Tab.Panel>
)}
</Tab.Panels>
</Tab.Group>
}
const App = () => {
return <gradientrect class="w-full h-full justify-center items-center" colors={[c("#42c873"), c("#06a0bb")]}>
<ExampleTabs class="mb-4" />
</gradientrect>
}
render(<App />, document.body)
function randInts(n, min = 0, max = 999) {
let array: number[] = [];
for (let i = 0; i < n; i++) {
array.push(Math.floor(Math.random() * (max - min + 1)) + min);
}
return array;
}
import { Dom } from "OneJS/Dom"
import { Tab } from "onejs/comps"
import { h, render } from "preact"
import { Style } from "preact/jsx"
import { Vector2 } from "UnityEngine"
import { useRef } from "preact/hooks"
import { classNames } from "classnames"
import { parseColor as c } from "onejs/utils/color-parser"
import { ScrollViewMode, WheelEvent } from "UnityEngine/UIElements"
interface ExampleTabsProps {
class?: string
style?: Style
}
const exampleTabs = [
{ label: "Tab 1", nums: randInts(200) },
{ label: "Tab 2", nums: randInts(200) },
{ label: "Tab 3", nums: randInts(200) },
{ label: "Tab 4", nums: randInts(200) },
{ label: "Tab 5", nums: randInts(200) },
{ label: "Tab 6", nums: randInts(200) },
{ label: "Tab 7", nums: randInts(200) },
{ label: "Tab 8", nums: randInts(200) },
]
const ExampleTabs = ({ class: classProp, style }: ExampleTabsProps) => {
const refs = Array.from(Array(exampleTabs.length)).map(() => useRef<Dom>())
function onChange(index: number) {
log(`Tabs index changed to ${index}`)
}
// Fixing the UI Toolkit Scrollview speed bug
// https://forum.unity.com/threads/listview-mousewheel-scrolling-speed.1167404/#post-8258421
function onWheel(index: number, evt: WheelEvent) {
let sv = refs[index].current.ve as any
let scroller = sv.verticalScroller
if (scroller.highValue > 0) {
sv.scrollOffset = new Vector2(0, sv.scrollOffset.y + 1000 * evt.delta.y)
evt.StopPropagation()
}
}
return <Tab.Group class={`flex-col w-[700px] ${classProp}`} onChange={onChange} style={style}>
<Tab.List class={`flex-row justify-between rounded-md bg-black/50 p-1 mb-2`}>
{exampleTabs.map((tab, index) =>
<Tab name={tab.label} index={index} class={({ selected }) => (classNames(`flex-row rounded-md text-white/80 items-center bold justify-center p-3 transition-[background-color] duration-200`, selected ? `bg-white active-text-color` : `hover:bg-white/10`))} style={{ width: `${98 / exampleTabs.length}%` }}>{tab.label}</Tab>
)}
</Tab.List>
<Tab.Panels>
{exampleTabs.map((tab, index) =>
<Tab.Panel class={`bg-white rounded-md p-5 flex-row flex-wrap`}>
<scrollview key={`${index}`} class="w-full max-h-[200px]" mode={ScrollViewMode.Vertical}
ref={refs[index]} onWheel={(e) => onWheel(index, e)}>
<div class="w-full flex-row flex-wrap">
{tab.nums.map(num => <div class="m-2 justify-center items-center w-10 h-10 bg-orange-200">{num}</div>)}
</div>
</scrollview>
</Tab.Panel>
)}
</Tab.Panels>
</Tab.Group>
}
const App = () => {
return <gradientrect class="w-full h-full justify-center items-center" colors={[c("#42c873"), c("#06a0bb")]}>
<ExampleTabs class="mb-4" />
</gradientrect>
}
render(<App />, document.body)
function randInts(n, min = 0, max = 999) {
let array: number[] = [];
for (let i = 0; i < n; i++) {
array.push(Math.floor(Math.random() * (max - min + 1)) + min);
}
return array;
}
1 Reply
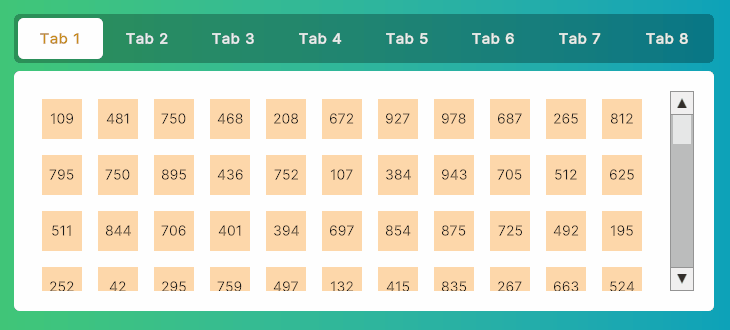