Is conditional rendering possible?
I have the following code:
getProgram
and postProgram
are wrappers around solid-query
.
When accessing the page with an id
in the query string (eg. ?id=123
) the first time, initialValues
equals to undefined
as the query is not yet finished and program.data
is undefined.
Then the query resolved and now program.data
is populated, but the form is already rendered so the form does not have any default values.
Hitting a second time the same page with the same id will lead to a populated form: the cache is already present so this time program.data
is defined.
Is it possiple to trigger a re-rendering à la React - (don't shoot me 🙈 ) - to rerun createForm with the actual data?
Or should I extract the createForm
+ <Form />
is a separate component and wrap it with a conditional <Show />
?14 Replies
Personally I’d do the latter - don’t create the form until initial data is ready, unless you want the form to still render while the data loads, in which case id reset the form once the data loads with the new data
the trick is I would like to reuse the form for both insert and update
id reset the formmeaning having a formRef.reset()? not sure if modular forms allow to reset a form
I think the form state should have a reset function?
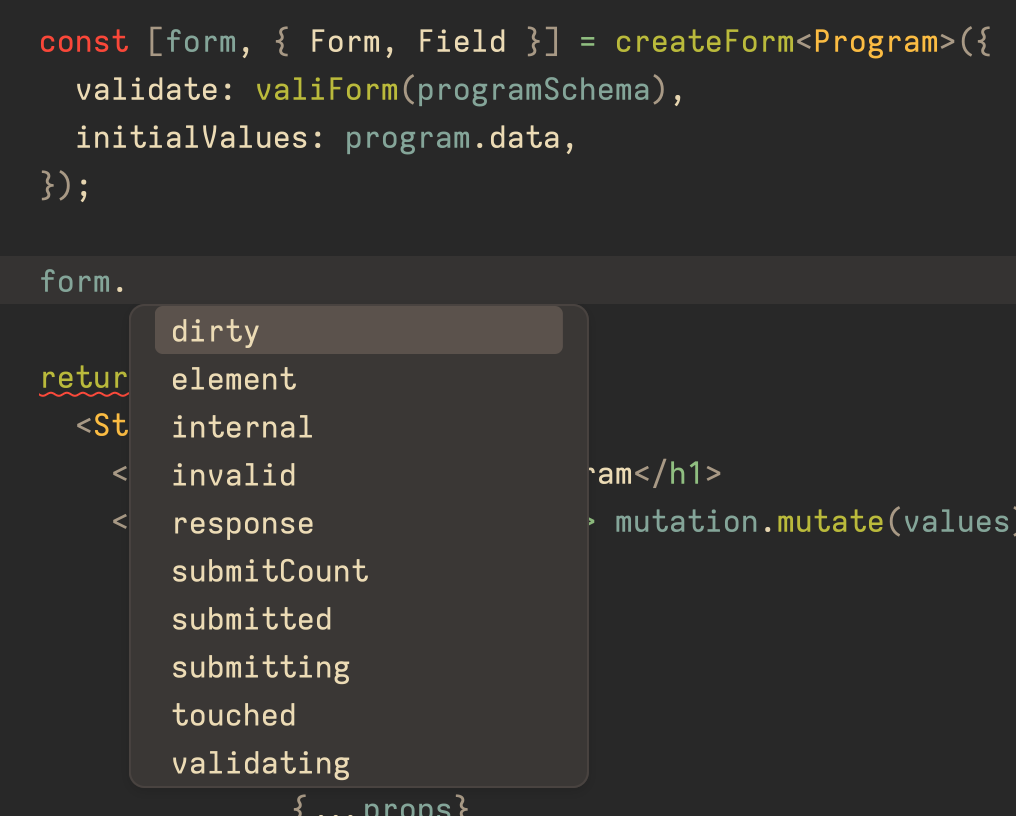
nope 😦
I think I'll have to do the latter
or separate the views
create
and update
Hmm rip
haha, rest in peace?
here's the docs https://modularforms.dev/
I swear I read it 4 times already
yet...
there is indeed a way to reset the form (https://modularforms.dev/solid/api/reset)
ty both for the brainstorming and the pointers 🙏
Ahhh right it’s a separate function
Is this VSCode?
If so, what theme?
Zed editor
"theme": "Gruvbox Dark",
"font_family": "JetBrainsMono Nerd Font Mono"
Okayy. I’ll check out the editor.
perhaps the theme exist for vscode too