Entity framework exception while creating a many to many relation
Hello everyone,
I've been trying to make a many to many relation between two classes
Voyage
and Transporteur
.
these are the Three classes: 23 Replies
This is my dbContext:
This is the exception:
I'd try configuring the join table with
.UsingEntity()
how?
I've tried this to be more precise
but got another exception
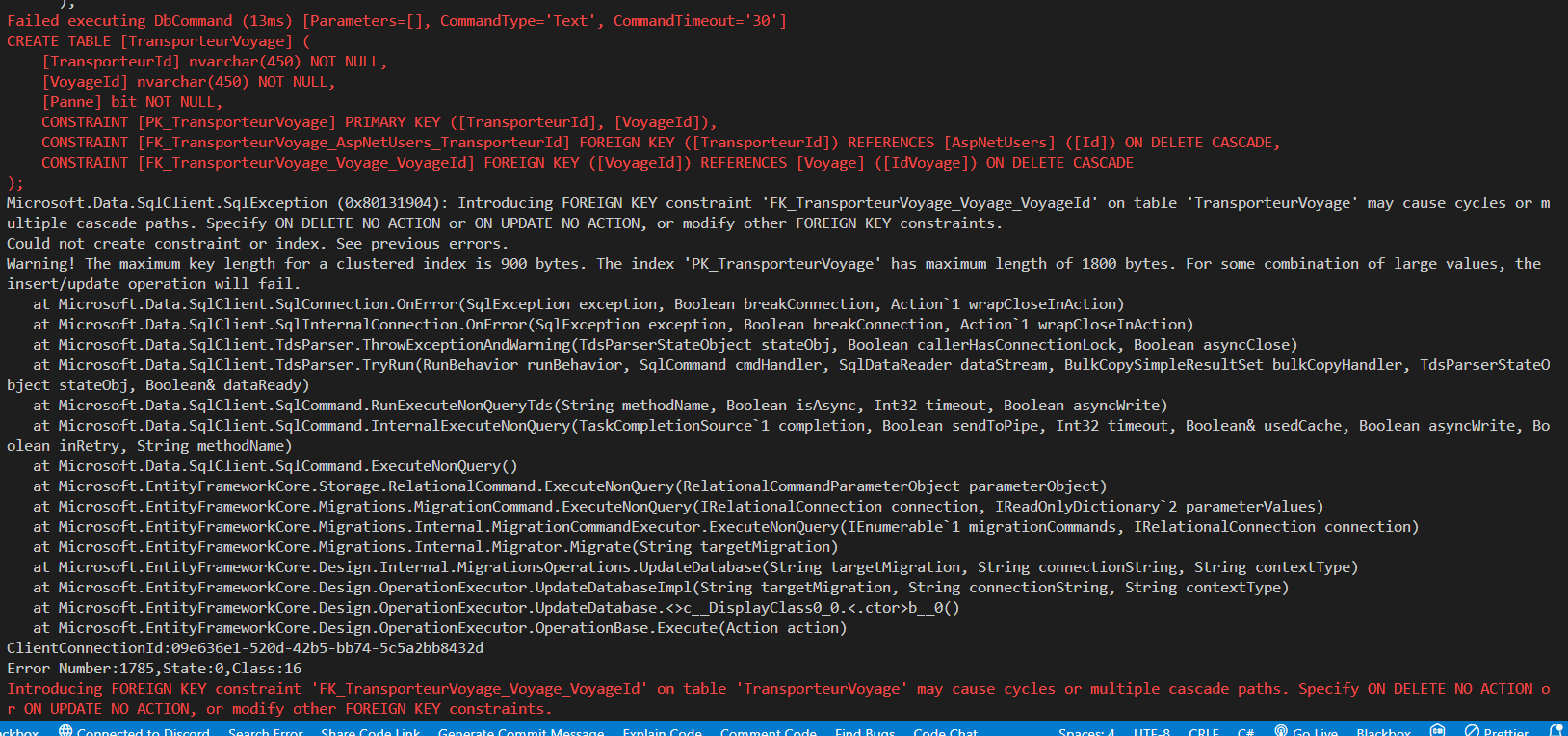
Why are the IDs 450-character long varchars
The Transporteur inherits from IdentityUser
I'd change the type of the key it uses
To a GUID or something
How can I change it?
Because varchar IDs this long are a bad idea
Transporteur : IdentityUser<TKey>
TKey
being the type of the keyow
You'll need to recreate the migrations, mind you
Or, well, at least create a new one
Actually... you will need to drop the database, delete migrations, and create them from zero
I'm still creating my database
so np
Since PK change is not backwards compatible
Just one more question
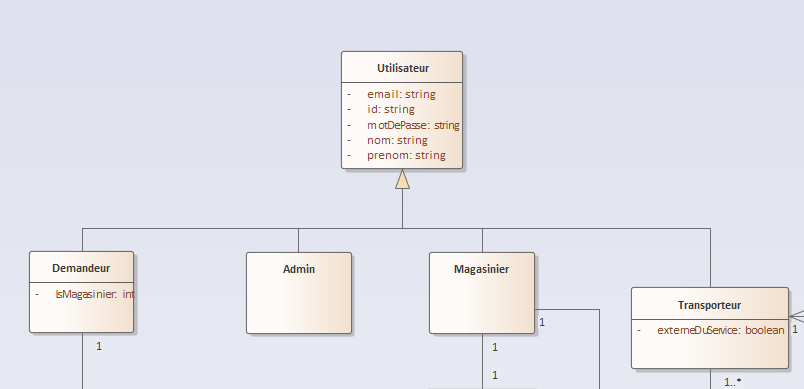
I have these 4 classes
that extends Utilisateur
what I've done
I created the Demandeur, Admin Magasinier and the transporteur classes
all of them extends the IdentityUser
is it a good approach?
or there is a better one
IMHO the best approach would be to use roles, not inheritance in this case
But, sure, inheritance should work as well
my business logic imposes inheritance
Then you do what you must
Tysm for your time
no problem