39 Replies
Yes and no.
your
MembershipManager
doesn't actually do any meaningful work, it just manipulates a value on the Person
model itself, which is already publicly mutable.
But if the methods on that manager did something more interesting, like talk to a database, or emit messages to a broker or something, then you might have a more appropriate case for this structureYes in a real world scenario I would write to the database instead of just
person.Membership = true;
.
Still not sure why I wouldn't use an abstract class for this?I don't follow, what would be abstract here?
I feel like I'm not yet fully understanding why I should use an interface here and not just something easier
I mean what is the useful thing about an interface here
Nothing at all
its entirely dead weight
What would be a case where I definitely should use an interface?
Two classes that share the same functionality
Interfaces are useful in two capacities: guiding an implementation (oh I must have this method to fulfill the interface...) and providing an abstraction over multiple implementations
lets say I have a
IOutputHandler
interface
we might have a ConsoleOutputHandler
and a FileOutputHandler
or maybe even a DatabaseOutputHandler
as long as they all implement the same interface, the rest of your codebase doesnt need to care about where the data actually ends up, or how its written there
you just know that IOutputHandler
has a Write(Guid id, string data)
methodIs this what you mean
yeah
Okay so I'm using the same function over multiple classes
And that's not what I'm doing
so in this case, you can have another method take a
IOutputHandler outputHandler
as an argument
and you dont need to know what specific implementation that isI don't understand what you mean with
and you dont need to know what specific implementation that is
one sec
something like this, for one
we can refer to all the three outputhandlers as the interface
and why is that useful
why is it more useful than not using an inteface
You just know that it has a
Write
method with a given signature. That's all you're concerned with, not where it's written. That's the concern of something else.
if we dont have an interface, how would you put these three things in one list?
Lets you set which writer you use once
thats another great example
Ah okay I think I get it
But it will become fully clear once I have to implement this myself
the core idea here is "abstraction", the ability to refer to something as something else (something less specific)
Also, if you were to distribute your output handlers as a package, I — the user of the package — could define my own
DiscordMessageOutput : IOutputHandler
and also use that, to use some webhook to send messages to my Discord server
That's the, uh, open-closed principle from SOLID?yeah
the O in solid 🙂
So I could also do this
Instead of using
IOutputHandler
as a interface, I can just call each handler from Main()
like this.
But an interface would be more practical because of abstraction, I dont have to create a tightly coupled instance with each specific handler classes to access it. So it's better to just call the interface instead, like this:
that, but also the extensibility
if your entire system uses the interface instead of a concrete class, someone else can make their own implementation (
DiscordMessageOutputHandler
) and it will just work
Have you heard of dependency injection yet?
Interfaces play a big role there, both for multiple implementations, swapping what concrete implementation is used based on a setting, etcI've heard of it before. I'm just walking through a C# .Net roadmap make sure I understand everything before I apply for a job. But I'll look into dependency injection. I think it will help understanding interfaces as well. I do get why it's useful but I just need to see some real life examples
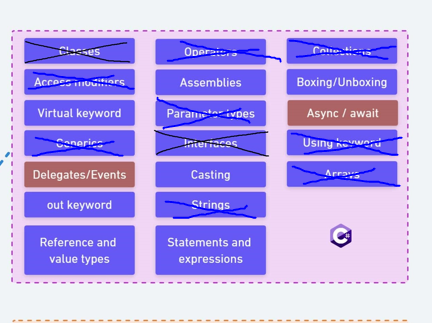
I made a video on DI a few years ago. Its not perfect, but its fairly short and no bullshit: https://youtu.be/USBoZtGt0QU
Thx I'll check itg out
I really should re-record that some day 😄
:catpog:
Heya leo
good video, I like how u explain a bit into the singleton vs transient
u should make more videos u explain things very well and have a clear voice
I have made more videos 🙂
https://www.youtube.com/playlist?list=PLNrLTXgfP7pOOIMcHghOgUOBtrPxrCqcA
DI, delegates + events, async/await and tasks, unittests, git, builder pattern, reflection
I saw those I was saying in general these small 10 minutes explaining 1 thing and doing it well
ah yeah
im a fan of those and u do it very well 😉
sorry hijacked the thread lol
I'd like to do more. But I need ideas on what to do and talk about
also yeah, sorry Falco
I be gone sorry falco 😛
That's okay no worries:)