Moving Element with Javascript
I want ginger cat image to move 50px in the direction of the button click
https://codepen.io/JimKi8/pen/YzMOQVp
51 Replies
and because its a maze i want the cat not to be able to go through the walls
use can use position property
use position absolute, position the cat on top left either with css or js
everytime a button is clicked, for example if right button is clicked, get the current x position of the cat, this will return you the x position of the top left corner of the ing so u need to offset the value by half the image width to get the horizontal central point. then add 50 to the value set that as the
left: ;
property
u would need to use some sort of box collision detection
tbh, i would just use grid
and set the cat image and bg image to whatever tile it's going to go to instead of ACTUALLY moving the img
u won't have that transition but it's gonna be less painful
and won't look too bad
if u use grid, then u can also use bitmap to create mazecan you give an example because I've done similar stuff but I can figure it out
idk what bitmap is
but how would i move the cat when it is as a background img?
that's the neat part, u don't
say the cat is on the 1st tile, u want to move it to the 2nd tile
u remove the bg image of the cat from the 1st tile and add to the 2nd tile
u get what m saying?
yea but how do i do that?
it's a 2d array of 1 and 0 or even other numbers but mostly 1 and 0
where 1 might mean wall
0 might mean empty tile
you use that array to represent the board
i just told u
well
first of all, don't worry about walls rn
just make the cat move
but i want to know where to move the cat
whichever method u want to use
the cat will move based on what btn has been clicked xD wdym
so for example
if the right button is clicked, u check which box is on the right of the current box
is there any way i can see the near boxes to my current position?
then u basically remove the inage from the current box and add to the box on the right
now how to figure out what box in on the right left top bottom, that's a tricky part
but u should be able to solve it
I personally would use matrix method
like your whole board is a 2d matrix
here u can use indexes to uniquly identify each box like we do in matrix
where 1st digit mean the row
2nd digit mean the col
so when say your current box is (1,1) and u wanna know what box is on the right, u just increase the col digit --> (1,2)
for left u decrease --> (1,0)
for top u decrease row digit --> (0,1)
for bottom --> (2,1)
ya what I just explained
so i should make a "map" of the maze with the matrix method?
well, that would be a more flexible method instead of hardcoding with html and css
cause then you can just make an array of such maps with just 1 and 0 / bitmap
turn your static game into dynamic by adding different level
or heck, u can even procedurally generate bitmap but that's a bit too hard xD
my brain is going to explode right now
the "map" would be like this? ...?
the bitmap for your current maze would be as follows
where 0 = orange and 1 = brown box
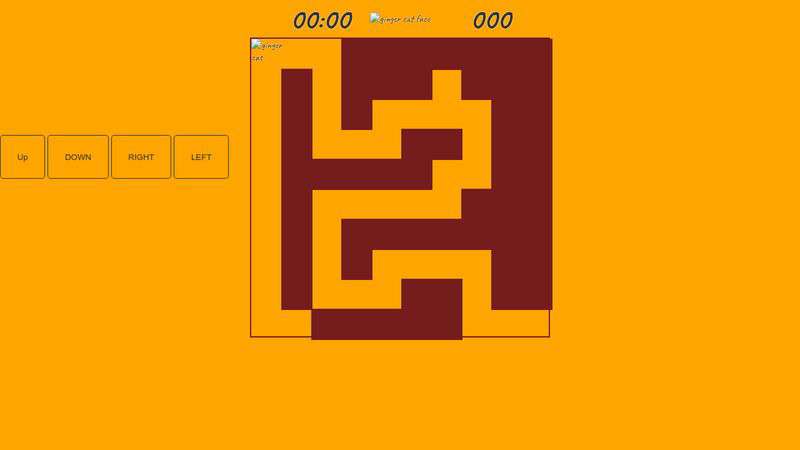
☝️
oh wow thank you
now here for example u can use a nested loop to loop through the whole board
for each bit u make a box and give it the defined color
u can use the bitmap to keep track of current box and boxs near using the same index method
and u can use the index method to check if a box next to the current box is a wall or not by checking if the value is 1 or not since 1 represents wall
welcome
I'll try it in like an hour and if I run into a problem I'll send a message here
try to isolate each section of the code into a different function
for example,
your first function might be
createBoard()
the sole purpose of it is to loop through the bitmap and set up the boxs nothing elsehttps://codepen.io/JimKi8/pen/YzMOQVp
@glutonium can you check now? the cat moves perfectly but when i move it another cat appears in the starting position
the buttosn don't work
i realise your html doesnt even have a start button
Ohh its because the image is as background you cant see it in code pen
The timer starts when a button is clicked
Csn I upload images in code pen? Idk
upload the image on discord
open the image from discord in browser
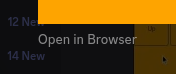
then copy the link and use that as src=""
https://cdn.discordapp.com/attachments/1229727540948504576/1229809464371187772/ball-of-yarn.png?ex=66310851&is=661e9351&hm=e932939c1b9e7099de93b5c3a457b1eb2c6af4a31bd8d24aa8db2a7f7e1375f5&
https://cdn.discordapp.com/attachments/1229727540948504576/1229809464635293726/cat-food.png?ex=66310851&is=661e9351&hm=0e1fd884b95d199b176bdc7c9e47ca0307c3d6b36d46458142f825db5668a23c&
https://cdn.discordapp.com/attachments/1229727540948504576/1229809464874635374/dead-fish.png?ex=66310851&is=661e9351&hm=b86c1a29406289dcf7248ed5c3c4ebb14cce8dd2dd846c07d1df7daea5a8bc6d&
https://cdn.discordapp.com/attachments/1229727540948504576/1229809465205854328/ginger-cat.png?ex=66310851&is=661e9351&hm=5087d115807e9c6b52669bd8b3b3c0066e53b95b41f18fb2342f5cd3b3740a21&
https://cdn.discordapp.com/attachments/1229727540948504576/1229809465432211456/ginger-cat-face.png?ex=66310851&is=661e9351&hm=3814a642051eb1852383e0368b0758a867a29a6bbe9933d191c1d0852f46b891&
https://codepen.io/JimKi8/pen/YzMOQVp
ok you can check now
some of the imgs are not being showed but that's not a problem
it's still the same
Yeah idk I'll just describe it
So when I move the cat in a box a new cat appears at the 0,0 position
u need to make sure to remove the background image from the previous position as well
Yea I've done that but the image only stays on the 0,0
Every other box removes the image except this one
you never set previousCatBox to anything before clearing
you should initialize it to the start position instead of
null
once u r done with the project lemme know
i also made something similar to yours, I'll share my code so u can check it out
yea go for it
i finished the project and delivered it, i made 3 difficulties
thank you for the answer, I delivered the project
Thanks you guys
k I'll share the code here
I can't log in to codepen for spme reason
I'll just send the code here
your code is much more readable than mine, maybe it would be better to change the j with x and i with y to indicate the axis
I don't understand this part of the code
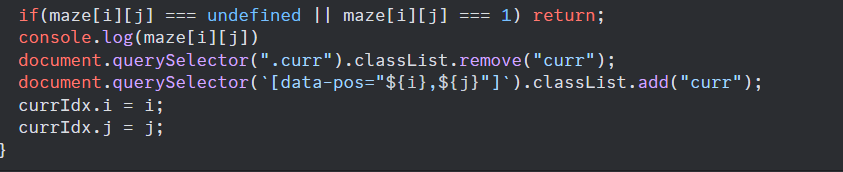
line by line,
as for x/y vs i/j, that's probably what I'd do too, but there's an argument to be made that i and j are more often used as array accessors. They're also used in createBoard in the for loops, so it's consistent with those (though that has no programatical reason for being consistent)
ya what jochem said
document.querySelector(".curr") fetches the element that is currently where the cat is, before it is moved
the next line fetches the box where the cat will be after we move it
but in order to move, we remove the cat from the element with curr class by removing the class itself and we add it to the element where the cat will be
good practice would probably to use an optional chaining operator on the
querySelector(".curr")
, just in caseagree
we can use the same index method to get the curr element, since our bitmap corresponds to the actual board as well
thanks for the explanation
ok now i understand exactly how this works but I would never do it by myself
at least for now
well dw, these r nothing pro level coding 😂
you just need a bit of practice. once you understand how to modularize and isolate parts of your code independent from one and another you'll start to write clean code as well
but yah, all u need is practice
the more i code the more i realize there are so much to learn...
it's not just learn, but also practice. People often think coding is just something you study and then you're great at it, but there's so much of it that is just repetition and training, just like any other creative work people do
yea that so true, you need to think in code, i think thats the tough part
This is the tough part, the breaking down and modularizing
that's true
you need that problem solving mindset
which is hard to achieve
once you have that though, there's very few programming challenges you can't solve