✅ Entity Framework One To Many: Adding to the dbContext and getting value '{Id: -2147482644}'
54 Replies
Error is: System.InvalidOperationException: 'The instance of entity type 'CheckGroupModel' cannot be tracked because another instance with the key value '{Id: -2147482644}' is already being tracked. When attaching existing entities, ensure that only one entity instance with a given key value is attached.'
error occurs on "await context.Patients.AddAsync(model);"
Don't use
AddAsync()
first of all
Or AddRangeAsync()
Patient model:
Second, what's that weird...
QueryOnContext
method?Why
the tutorial i followed used it and it did well for me. I was going to review it once i knew more about what im doing
all the calls in the service use it.
It's just a completely useless method
But aight
lol, that doesnt surprise me
Replace your
.AddAsync()
with .Add()
the guy also used AddAsync everywhere
And
.AddRangeAsync()
with .AddRange()
You should just throw away this tutorial, then
It shoes the guy has absolutely zero clue about EF
Just a "monke see async monke use async"should I need to add the range? I added it part of troubleshooting
Idk, do you want to add multiple records to the database at once?
the PatientModel has another model within it and I'm not sure if I can add it that way or if i have to make the nested model seperately
PatientModel has a list of these
Two ways you can add a new
CheckGroup
to a Patient
:
the top on would require a SaveASync too?
SaveChangesAsync*
Yes, good catch
great, thank you
I've covered the documentation, but do you have a good reference or guide for EF?
The documentation ¯\_(ツ)_/¯
it's easy to find something out there that works, but I'm finding it hard to find something that doesn't make other recoil when you mention it's your guide
https://www.learnentityframeworkcore.com/ is sometimes useful as well
ok great, that's been one of my resources
awesome thank you for the help!
Anytime :Ok:
quick followup: if i have a new patient and want an id, I must: add patient to context and save it to generate that Id? Is that the standard approach?
Yes, the database generates IDs
But you can create a whole hierarchy first, and all the IDs will be set up
For example
will create the blogpost, create both of the tags, and will set the ID in those tags properly
sweet, thanks!
wierd, still getting that error
happens on "context.Patients.Add(newPatient);"
Why are you setting IDs manually?
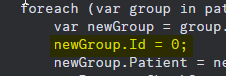
crap, part of my troubleshooting snuck in there
issue remains without it
Okay, another thought is that the issue might be elsewhere
Something also deals with those groups, patients, whatever, and doesn't release them properly
Immediate suspects:
* any
async void
method
* any unawaited async
method callhrm, I know enough to know to completely avoid async voids
would those things cause a "Id: -2147482644"? That feels like a int 0 goign back once
Angius
REPL Result: Success
Result: int
Compile: 174.163ms | Execution: 15.770ms | React with ❌ to remove this embed.
Close, but not quite
this is being called from a seeding service in the main program. the main program is async task. It appears to be async/await the whole path
took it out of the QueryOnExecute thing and same result as well
ok, I think you're right about that async thing, something weird's happening. My breakpoints for this whole method aren't being triggered anymore, but the patient is being created.
and this si the only place that could be happening
ok, it's been refactored to save changes after each entity is made. barbaric, but it seemed like the right choice to see whats going on
the error is occurring when the second CheckGroupModel is added. Maybe something is screwed up with the EF modelBuilder?
that, or calling await in the main Program.cs causes some funky things
It's not this. I tossed the Seeding to be called after done with the startup and it still happens
banging my head against this issue, the best i can see is that it's got to do with generating the PK for CheckGroupModel. I can make PatientModel entities just fine. I tried adding the patient id directly, doesn't work. It doesn't generate the foriegn key properly then or something
I've moved where this code cocurs, issue still remains
occurs*
what db you're using
Sqlite
Wait, could the dB cause issues with creating pk and fk?
well i've seen an issue before that was similar to yours where the db was filled with invalid data like null columns etc including in the primary key field
causing it to not respect anything being inserted
u could use dbeaver or dbrowser and check the sqlite file and see if its fine or not
There's no data yet. This error occurs when trying to use the service to insert
if possible can you create a github of the project? I will take a look when im free
Ya, it's currently private atm. I'll share it in a bit
GitHub
GitHub - nsmela/BlazorPatients
Contribute to nsmela/BlazorPatients development by creating an account on GitHub.
mmm
Shouldn't the fk be on PatientModelId?
ill check
no i think it's right. PatientModel is using the ChckGroupModel.Id as the foerign key
if i change it, i get foriegn key constraint issues
wait, let me double-check it
I think that was it! I completely removed that model config and everything is workign fine now
so far anyways
so .HasForeignKey is setting the fk for the child and not the parent? I noticed the generated code for migration refused to house the foriegn key on the parent
nvm, hasFK is to set the key on the current item. That's why i got confused
ok, triple-checked and that seemed to be the fix! Thank you so much!
but looking at your code I dont think u want one to many there
otherwise u will have duplicated checkgroups
and for that you would need to change
and then the process of adding seeds would need to change a bit.
u want to ensure you attribute the id if a check group exist as to not create another redundency
and what happens in all this is that you a many to many with a intermediate table and navigation
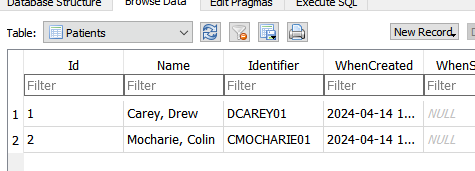
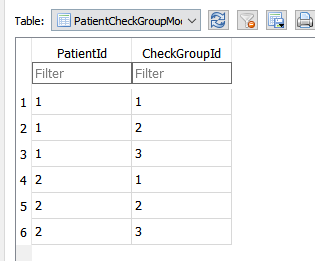
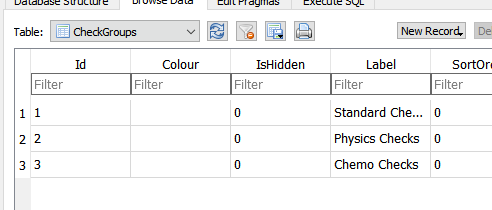
https://learn.microsoft.com/en-us/ef/core/modeling/relationships/many-to-many#many-to-many-with-navigations-to-join-entity
@ZZZZZZZZZZZZZZZZZZZZZZZZZ mind reviewing?
Fetching labels in a loop feels iffy, but if it's just for seeding then it should be fine
yeah its just for the seeding I couldn't see a way around it as groups are not attached and if I just create a new checkgroupmodel they collide
I appreciate the feedback, but I think I want the groups seperate/duplicated. I don't want patients sharing the because the groups are just collections of checks/tasks for each patient
Basically, each patient is like a Kan an task board
Kanban*
Groups and checks can dynamically be added and removed and have no link to what other patients have
I debated on even having groups, but it felt better than having a couple of extra fields in the patient or checks
Unless I'm misunderstanding what you're recommending
For the seeding, I'm considering just incorporating it into the migrations. I've been using the patient service to test run it
they are separated
but what I meant is
everytime u would be adding a new item
but if u have many to many
all the checks above only exists once
but in the joined table
u have the correlation of patient to groupcheck
so you can have a table with all kind of checks that exist
and easily link it to patients
this would also facilitate if u have a dropdown with checks u want to attribute to a patient
but yeah either u do your thing and u have both examples 😉
Hrm, I'll have to think about it. The request was that you could have two "Standard Checks" groups that could have entirely different checks and colored background. There will be a template version (reason why I had the navigation value as nullable)
yeah I dont know your requirements etc, but at least u have both examples and know how to do either now
Use the /close command to mark a forum thread as answered
Ya, but nonetheless it's appreciated.