✅ Help with Data Bindings
So, now I'm trying to create a Task Manager clone, just the graphs part for now. So, I have a UserControl called Graph.xaml which is basically a graph and a text box. Then I have a Home.xaml which is my View and then HomeVM which is obviously my ViewModel. Now, I have an issue. In my Graph.xaml I've created a Binding called GraphData and then through Home.xaml I'm trying to define that GraphData so that my User Control remains re-usable and I use it for other graphs too.
Home.xaml
and in my Graph.xaml, I've put it like this
but when I debug the program, it says that Graph.xaml is looking for "GraphData" in HomeVM and it's unable to find it, but instead it should look for "GraphDataCPU" as defined in Home.xaml, why is it not working?
28 Replies
Graph.xaml.cs
Home.xaml.cs
It would not accept the GraphData from Home.xaml but if I put Series="{Binding GraphDataCPU}" in Graph.xaml it works and I don't want to hardcode it.
Error from debugger
you dont need to overcomplicate things
the DataContext propagates to the child
so if u just have
<local:Graph/>
in your MainWIndow.xaml for example
and in your UserControl called Graph.xaml u have
and in your MainWindow vm u have a Series property
it will by default reach out to it
This is just an example to illustrate it:
XAML
Graph.xaml
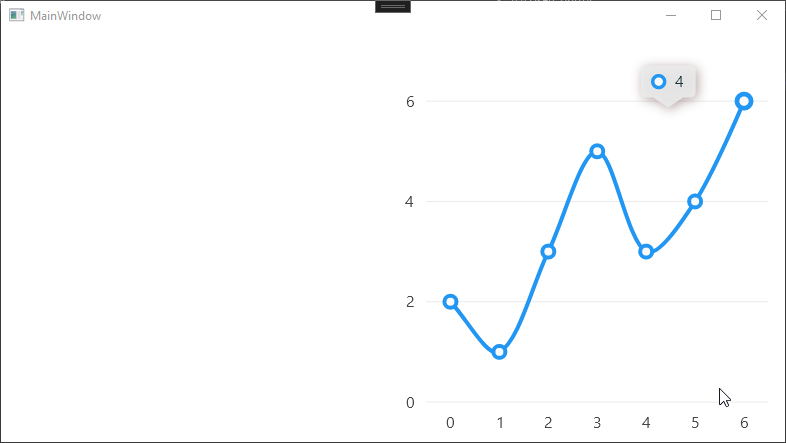
Yes but what if I want to re-use it, how would I change the DataBinding in that case?
I see you want to have multiple Graphs
Yes
one way would be exposing the binding property
the other would be just setting its own datacontext
let me see what u did above moment
alright
and add to your UserControl
x:Name="self"
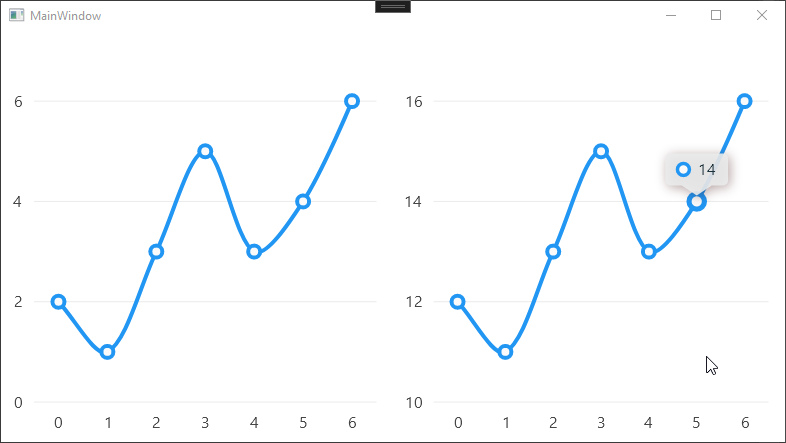
u were nearly there 😉
so close yet so far
wait hold on I don't understand one thing
without self it tries to look for GraphData in the parent
you said
add to your UserControl x:Name="self"sooo ?
that is not inside the graph.xaml
and its not using self
unless I missed something
uhmm... can you please pin point me sometimes I'm just so dumb
ahhhh
ok
like just doing x:Name means nothing if you're not using it in the scope
it works but
so if u did it on the MainWindow
a lot of errors and warnings in the debugger
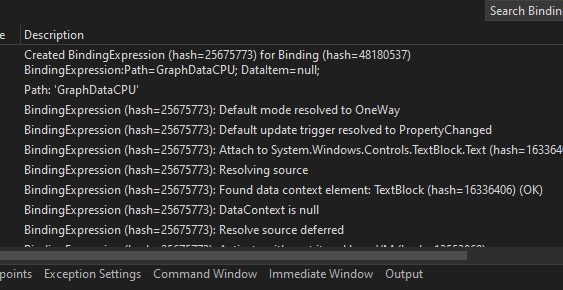
it wont propagate to the child
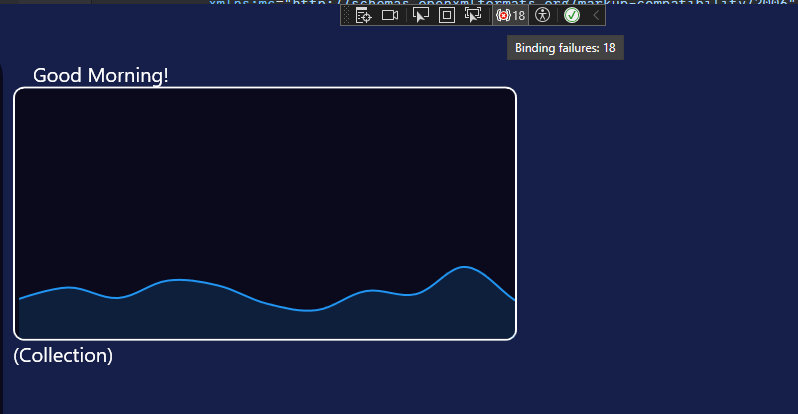
I mean I dont know what else u have there... I only replied from what u provided me
probably me then, I'll have a look at my code because it's a mess rn but at least I got it all figured out
thanks again
👍
Use the /close command to mark a forum thread as answered