Issue Querying Table via Drizzle ORM with SQLite
Hello Drizzle Community,
I'm currently facing an issue with querying the
tbl_workshops
table in a SQLite database using Drizzle ORM. Despite confirming that the table exists and is properly structured (I can query it directly using SQLite tools), attempts to query it through Drizzle result in an error indicating that the table doesn't exist.
Error Message:
Environment:
- ORM Version: Drizzle ORM v0.29.5
- Database: SQLite
- Bun Version: Bun v1.1.0
- Operating System: win11
Code Snippet:
Here's a simplified version of how I'm setting up Drizzle and attempting to query the tbl_workshops
table:
I've already verified the following:
- The DATABASE_URL
environment variable correctly points to the SQLite database file.
- The table name in the schema matches the actual table name in the database.
- I can manually query the tbl_workshops
table using SQLite command-line tools or GUIs.
I'm puzzled about what could be causing this issue and would greatly appreciate any insights or suggestions from the community. Has anyone encountered a similar problem, or does anyone have ideas on what might be going wrong or how to troubleshoot this further?
Thank you in advance for your time and help!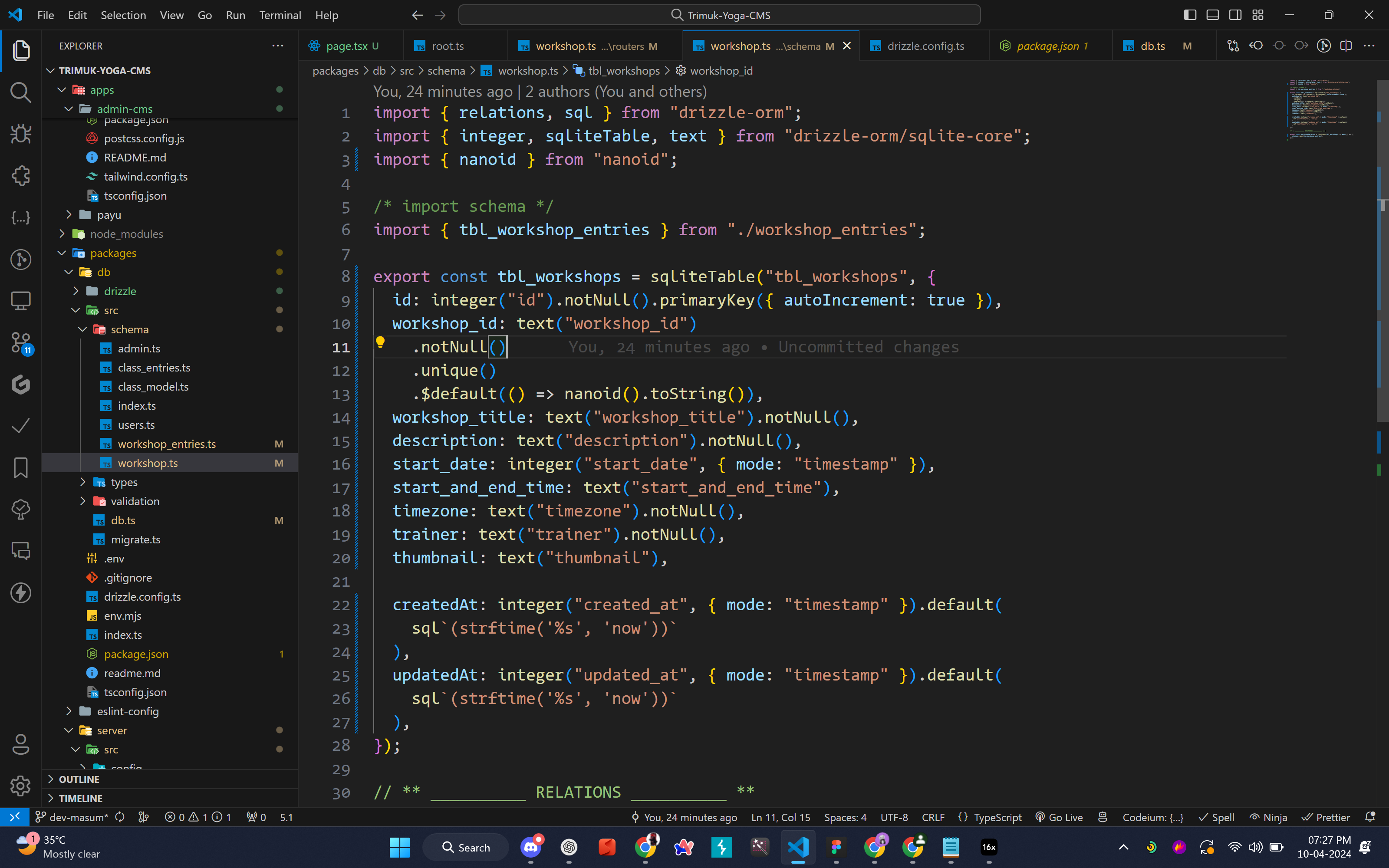
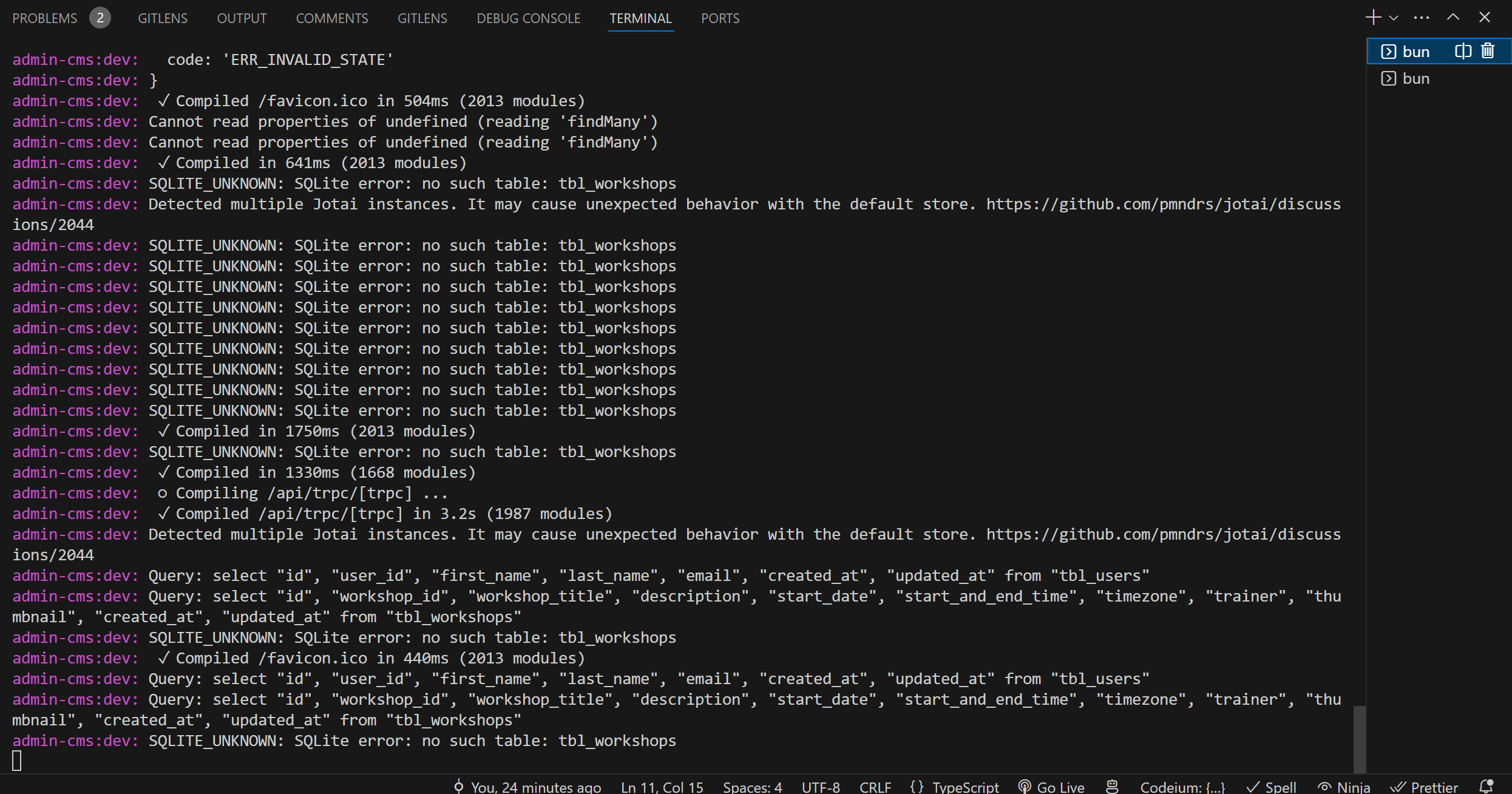
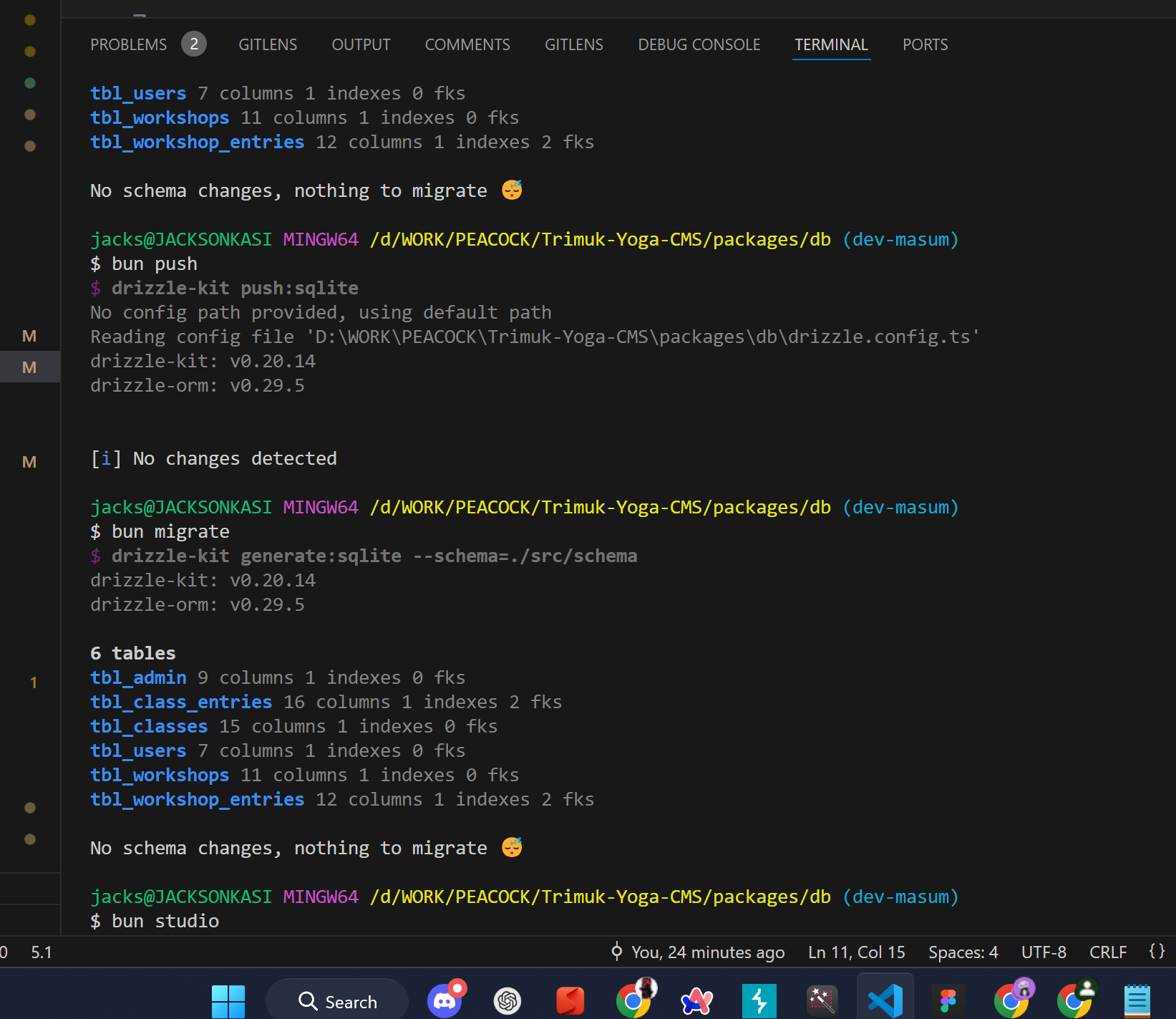
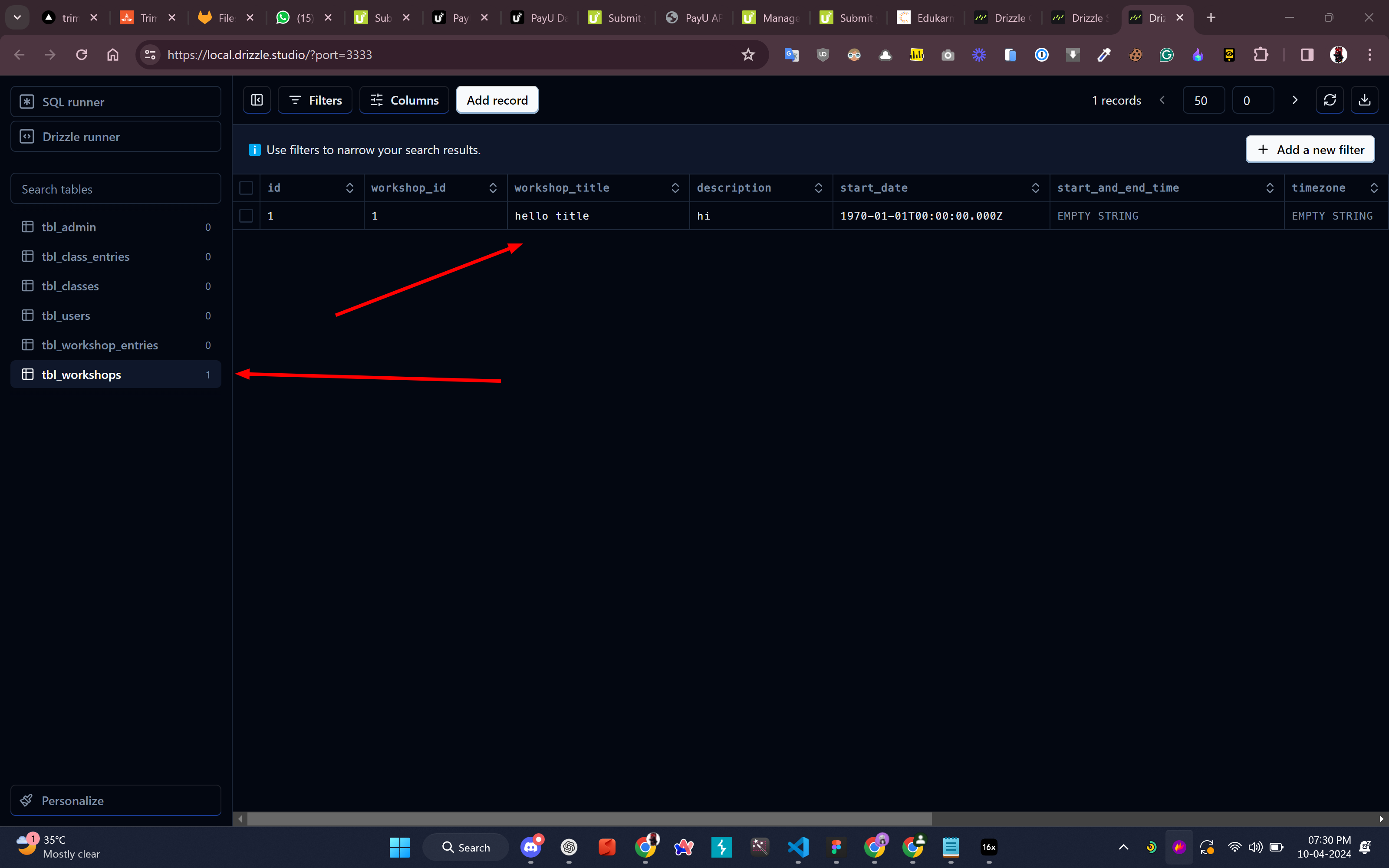
9 Replies
Hello, @Jackson Kasi! Thank you very much for detailed description of your issue!
Did it work with
select()
syntax? Could you please provide your raw sql query that you execute against SQLite database with cli and get sql query from your actual query?
Hello, @Mykhailo and thank you for the response!
I've tried querying the
tbl_workshops
table in two different ways using Drizzle ORM, but unfortunately, I'm still facing the same issue.
1. Using a more direct select
method provided by Drizzle ORM:
2. Using the findMany
method with limit
and offset
options:
Both methods result in an error message indicating that the tbl_workshops
table doesn't exist
Here's how I'm calling the .toSQL()
to inspect the generated SQL query
I've inspected the SQL query generated by Drizzle ORM, and here are the details:
Hi @Mykhailo, Can I set up the base code, make it, and then publish it in a public repository? I will share it with you to help me with this issue.Would be great! Do you use turso db?
yes
Even the database key, I will add it in the code! Later, I will remove the Turso DB from my account.
Are these the correct steps to reproduce the issue, or did I miss something?
1. Create turso db
2. Create drizzle schema
3. Push changes
4. Connect to turso db with drizzle
5. Execute query
no worries, I can use my own turso db
Yes, those are the correct steps to reproduce the issue.
okay then i will share the code soon!
Hi @Mykhailo
Just wanted to let you know that in the base version, I didn't encounter any issues. However, in my full version of the code, I'm facing an issue. I may have made an error in the TRPC config or somewhere else. I will try to fix the issue. Thanks for your support.
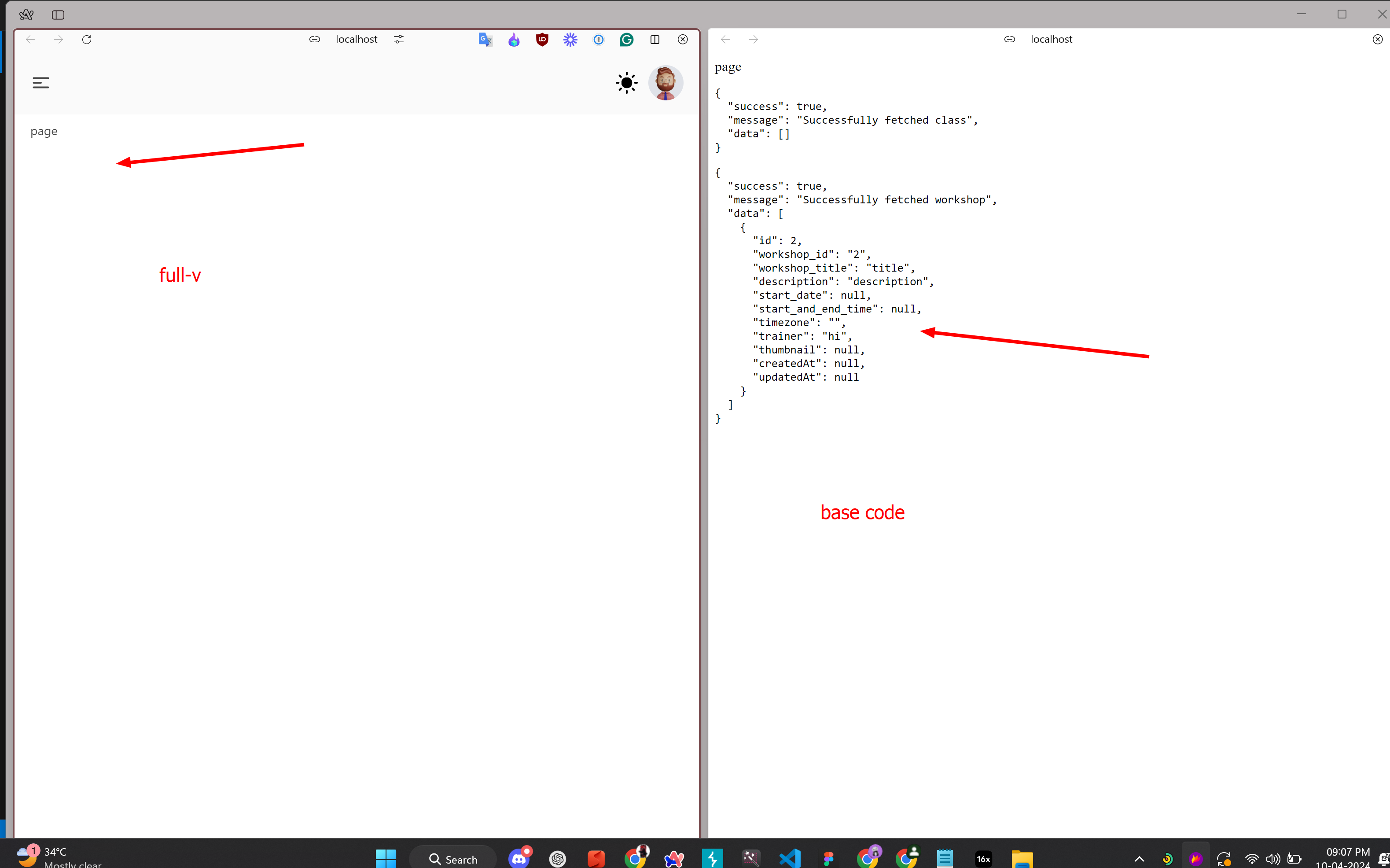
Super!
and this is the base code: https://github.com/jacksonkasi1/next-trpc-drizzle-turso
GitHub
GitHub - jacksonkasi1/next-trpc-drizzle-turso
Contribute to jacksonkasi1/next-trpc-drizzle-turso development by creating an account on GitHub.