Error: Can't resolve 'net' when importing schemas created with drizzle-zod to client components
I have created and exported a zod schema from the drizzle orm schema file with the createInsertSchema function from the drizzle-zod package. But I get the following error when importing the zod schema in a client file and passing it to react-hook-form. Most probably this error is because I am importing something in a client component from a server component and it has nothing to do with drizzle. I'm using next js 14 btw.
Here is the code for drizzle schema:
Here is the frontend code.
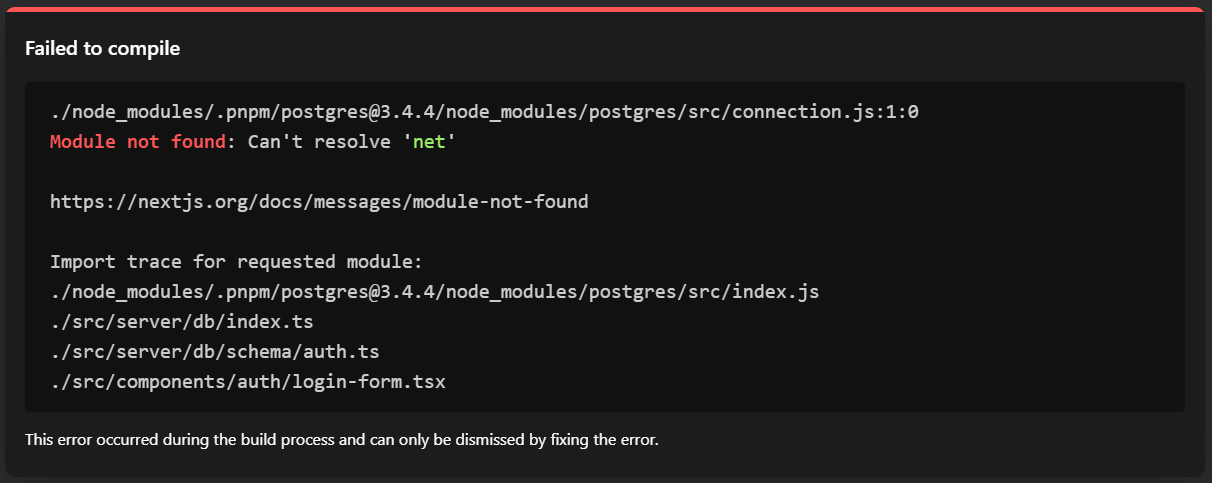
0 Replies