how to connect mysql to .net program as 2nd db
I'm using mongodb in my program and I want to add second db and I decided to MySQL. I created the mysql container but don'tknow how to connect to program.
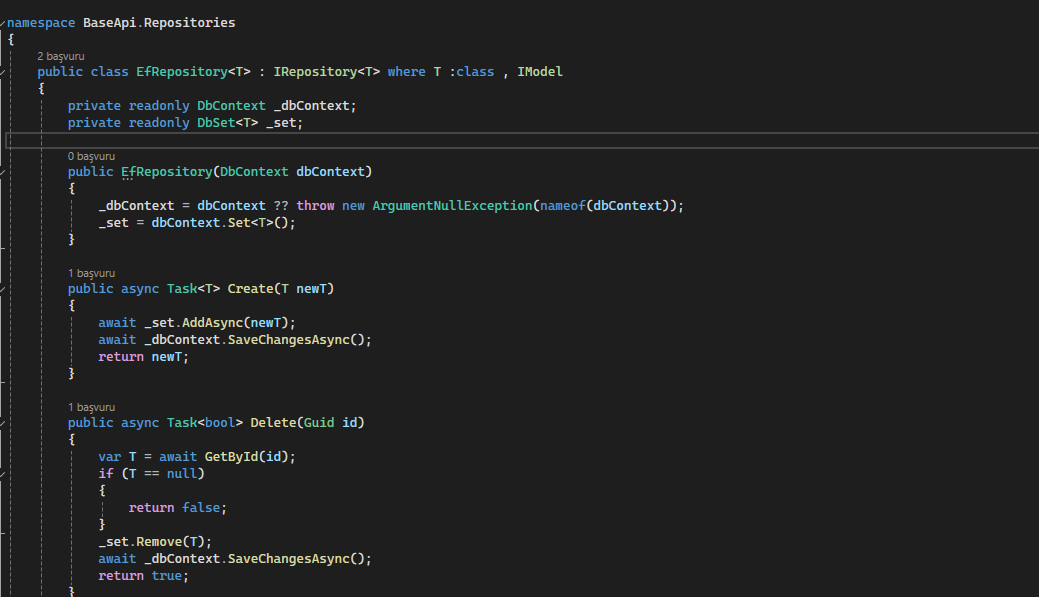
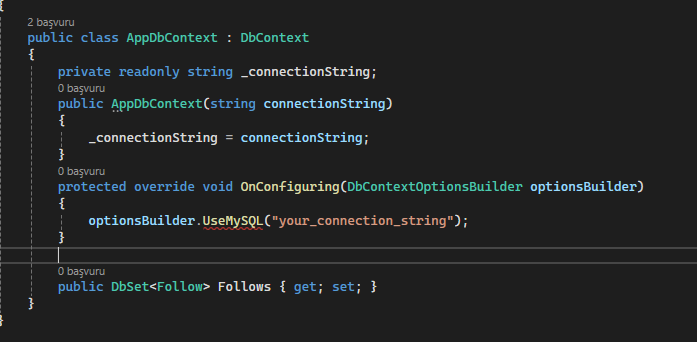
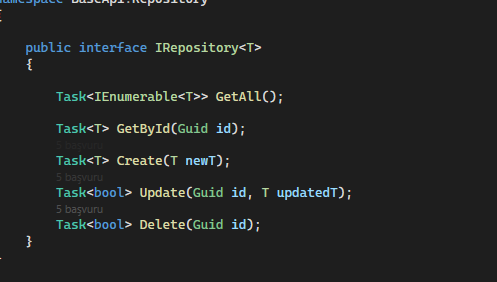
93 Replies
what do you mean by "second database"?
what are you going to store in it?
would you be running one or the other, or both at the same time?
I'll use both at the same time
okay, then make a second DbContext
oh right, its you again. you dont use EF for the mongo DB at all right?
Repository over EF is considered a huge anti-pattern FYI
yeah but for mysql I didn't want to write all queries
then what can I do except EF
the trick isnt to replace EF
its to not use a repository over EF
just use the context directly
can I use mysql like i used mongodb?
you can, using something like Dapper, or maybe even Linq2Db
EF is however the "default" way to integrate with relational DBs in modern C#
but it's against my structure?
then change your structure
its really simple, just dont use a repository over EF
in your service, inject the context itself, and use it directly
keep the mongo repositories if you like, thats fine
you know I didn't want to use methods of mongo in my services directly
yes
so you introduced a repo to abstract mongo away
but EF is that abstraction over mysql
you could change mysql for postgres, and probably all you'd need to do was re-generate your migrations and DB Snapshot
Em if u using ef coore and dependency injection u can just make another db context and then add it to injector
and also I can use EF for other dbs right? so make sense that i don't need a repo. so no need for making it generic its already
Pretty easy
yes, EF supports a whole range of relational DBs
If u talking about postgresql and others there are a lot of extensions for them
To connect ef core to them
MSSQL (SQL Server), Postgres, MySQL, Sqlite, Cosmos, Oracle, even Mongo (but I dont recommend it)
Mongo is non sql)
so for services that uses mongo I'll keep repos and for services that uses relational dbs no need for repos
Im aware, thats why I dont recommend it.
yh im planning to use mysql
specifically with EF Core, yes
because EFCore itself already implements the repository pattern
a Db Context contains one or more
DbSet<T>
s
DbSet is a repository abstraction
If you want shared, re-usable queries, like with a normal repository, you can implement that with extension methods on top of DbSet<T>
for a given TI'd like to cuz I don't want to define common crud methods over and over
you dont
EF already has all the CRUD
_context.Posts.Add(new Post(...));
exists.so I just define my custom methods
same with Remove,
Find(int id)
etc
yes, as extensionsgot it but how do I connect it to mysql?
make sure you use
Pomelo.EntityFrameworkCore.MySql
and not the other MySQL connector
this one doesnt suck. the other one sucks.which one is useless here
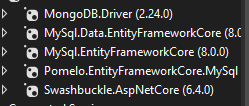
also what am I missing is it about my dbcontext?

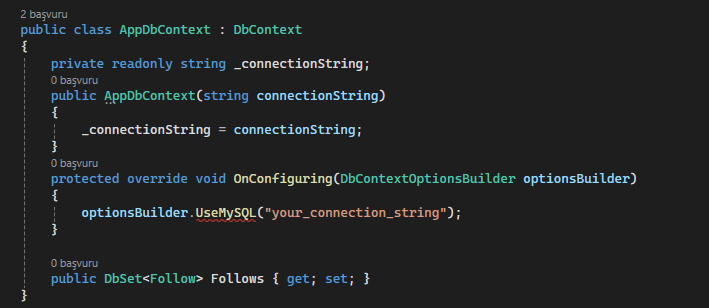
The ones starting with
MySql
what about my dbcontext?
what about it?
remove your
OnConfiguring
method, and change your constructor to inject the context options instead
literally follow any sensible EF tutorial
thats how your ctor should look
ofc you can rename the class itself, but you need that constructor argumentdo i have to inject this to appcontext or I can use it from program.cs?

you would resolve that from program.cs and pass it into
.UseMySql
how does it look

thats about right. just gotta fix your server version 😛
pls work🙏

ups looks like I did all of this for nothing...
my senior wants me to use sql without orm
what path should I follow @Pobiega can you help me please?
?
Ask your senior, lol
is Dapper fine? or does he explicitly want you to use ADO.NET?
ask them, get an answer, use what they say
or challenge the "no ERM" rule, but deal with them, not us
I asked him and he said "no orm at first
write some sql query
we'll see after"
that means Ado
dapper could also fit the bill
but since its consider orm u can't
the point of writing some sql is so you understand what it means to perform actions in the database
probably. I asked him is ado okay and he said it got many names 😄
SELECT, UPDATE, INSERT, CREATE, using joins subqueries are all important aspects to understand before u go into ORMs
sounds like a big yes to me
how do I connect to db while using ado
mysql.client is okay?
https://learn.microsoft.com/en-us/dotnet/framework/data/adonet/ado-net-code-examples#sqlclient
for mysql u would need something else
Be prepared for a lot of hard work when using ADO
if not mistaken the counter part of ado for mysql is MySql.Data
you need to manually map the results to the proper types, and line up your queries fields, order of select, etc
yeah
and the mysql docs are awful to follow
@only you know https://dev.mysql.com/doc/connector-net/en/connector-net-tutorials.html
this one looks ok for a start
uhh looks scary
guess why ORMs exist?
yh but rn not for me🥲
is it okay to construct crud methods in repo
yes
how does it look
SELECT id, name
reader.GetOrdinal("username")
one of these things is not like the otherfixed sorry except this any problem?
I dont really like using getordinal I dont see much point in it
and I imagine it might have a small overhead
but aside from that looks like a start
thank you
how do I use my connection string? its in appsetting.json and I tried to register but couldn't do it.

also here is the general look of my repo. does constructor look right
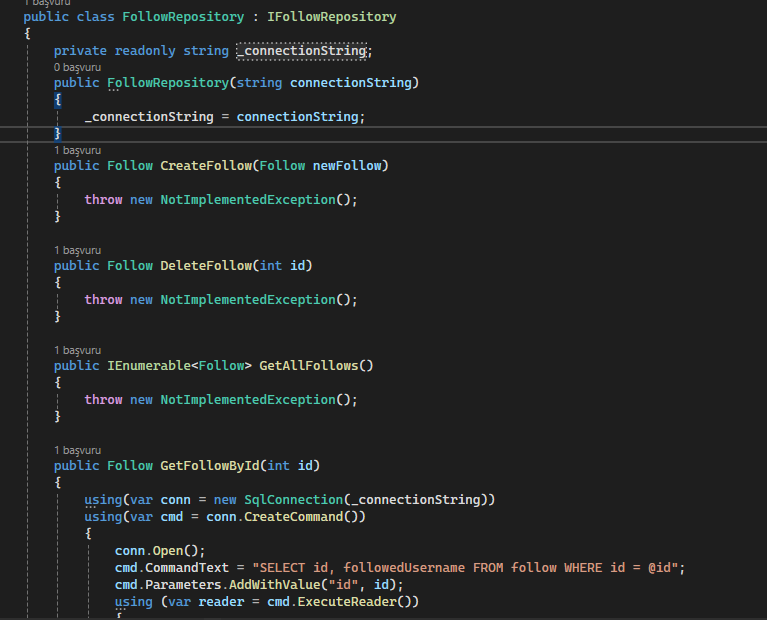
oh I did like this

@leowest @Pobiega when I register my IFollowRepo and Follow repo there is a problem because of IConfig
how do I register it?
program.cs is like this atm
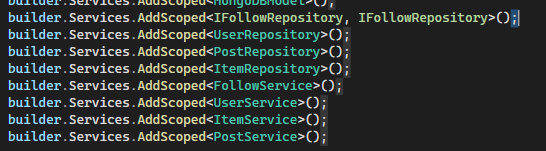
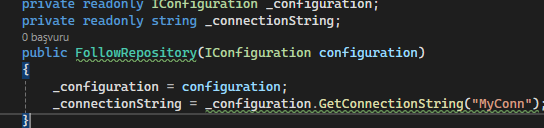
you dont
move it to its own config object
IOptions<T>
@Pobiega am I connecting to mysql db wrong cuz when I run service there is error says that "System.Data.SqlClient.SqlException: 'A network-related or instance-specific error occurred while establishing a connection to SQL Server. The server was not found or was not accessible. Verify that the instance name is correct and that SQL Server is configured to allow remote connections. (provider: Named Pipes Provider, error: 40 - Could not open a connection to SQL Server)'"
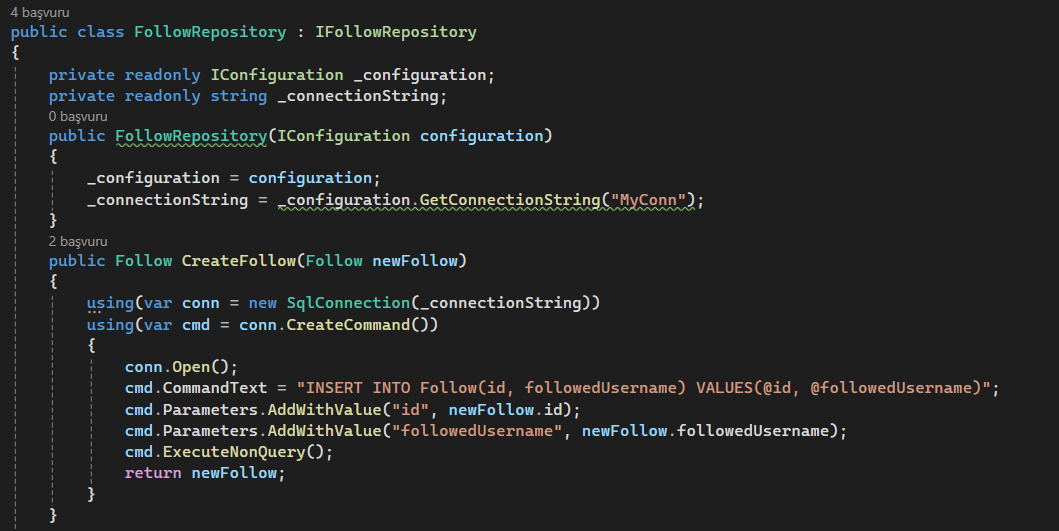

I created the container with this command
uuhh yeah
you are using
new SqlConnection
thats not for MySQL
thats for SqlServer
so you need to use their connection classoh
what connector did you install?

thats not for mysql >_>
🥺
but also, why oh why are you using mysql
its not a very good database to begin with
I have no idea just don't try to do as I'm told
how about this

yep
that sounds good
I changed sql connection as mysqlconnection
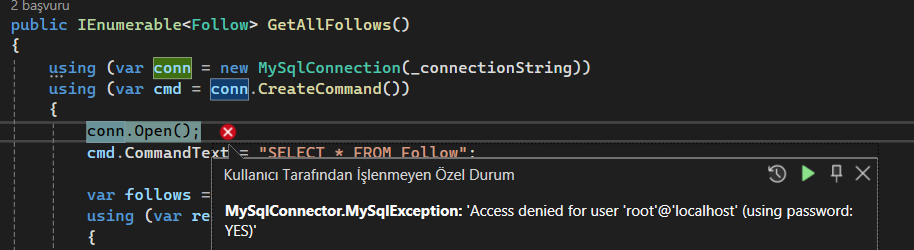
yep
thats a lot better
also I'm sure that mypw is true
well, thats an actual response from your mysql server
and it says that you did use a password, and you tried to connect as
root
and it failed. it almost has to be the passwordif we look here we can't see the root user. does root default right so no need to be defined
mhm
can you connect to it with some client?
HeidiSql, PhpMyAdmin etc?
I can connect from cmd
and I created the db and table
ok that should be enough
set a breakpoint in your code, verify that its using the right password
and that the encoding is correct
make sense let me try
make sure your settingsfile is utf8
I can see that connection string comes as it should be

okay, dunno then
ohh thanks anyway