db.query throwing undefined is not an object (evaluating 'relation.referencedTable')
Hi, so i have this schema called
product.ts
and it's related to category with one-to-many relationship
But when i try to do query findMany, it throws an error
The error like in the image.
Thank you!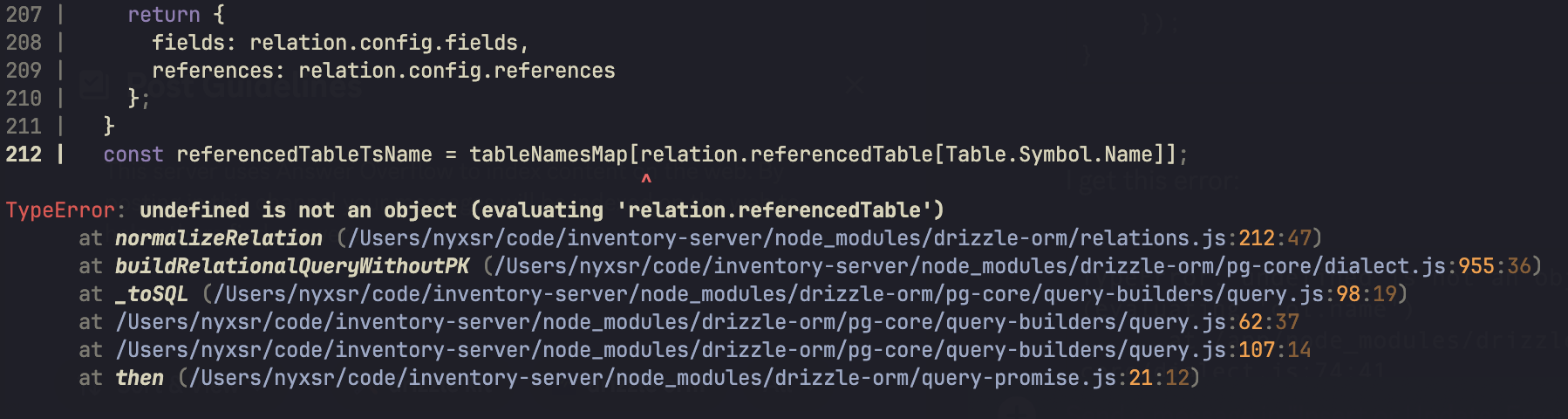
5 Replies
Hello, @NyxSR! Could you please show how do you initialize db? You might forget to pass
schema
parameter.
https://orm.drizzle.team/docs/rqbDrizzle ORM - Query
Drizzle ORM is a lightweight and performant TypeScript ORM with developer experience in mind.
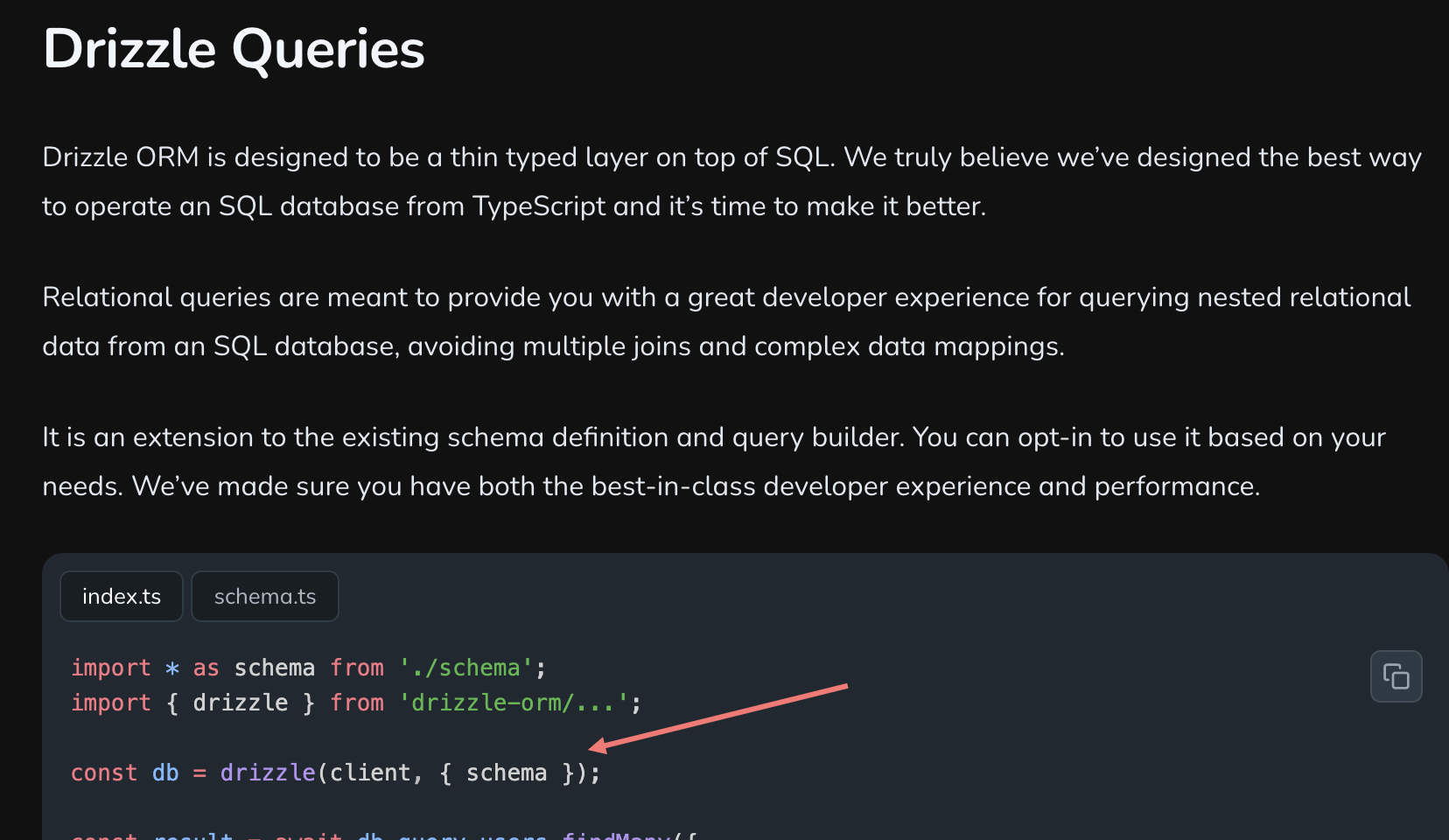
So i've created a file called
db.ts
and for the schema value inside db variables is from this. what inside
index.ts
from db/schema is just a simple file
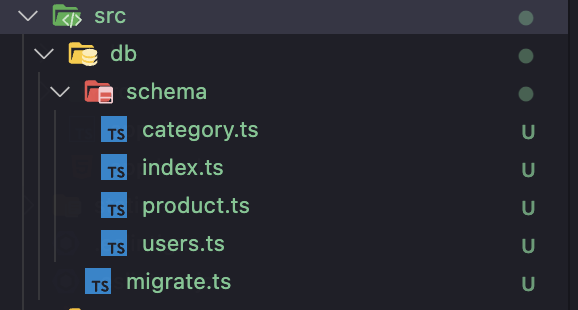
@NyxSR you have to update your exports because you need relations too and then you can import from your schema/index
Ahh i see, let me try that
Thanks a lot @Mykhailo , that works !