Why scope().compile().infer resolves to any?
I tried to follow the example here: https://arktype.io/docs/scopes
Not sure if this is a bug or if I'm doing it wrong
Stackblitz: https://stackblitz.com/edit/rzkceh-eodur9?file=demo.ts%3AL63,index.ts
9 Replies
It seems the intersection
account: "clientDocument&accountData"
is responsible for the fact, that the type popup was not appearing.
I moved account
from 2nd position after clientDocument
and accountData
and the type popup reappeared. But .infer() returns still any 🤔
// Not showing types
popup
// Showing types
popup
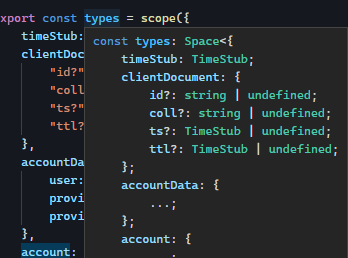
Hmm, that way
.infer
resolves the type, but why? 🤔
It seems, I can narrow it down to these two problems:
1. It seems the property order inside scope()
matters. If I define a property after it's being used, no popup appears for baseTypes
/ clientTypes
. But then how do I define types that reference each other?
2. How can a union be created where one of both types comes from another scope()
?
Unknown User•13mo ago
Message Not Public
Sign In & Join Server To View
Arktype 1.0.29
Can't see which TS version stackblitz is using (I've forked David's version)
https://stackblitz.com/edit/rzkceh-eodur9?file=demo.ts%3AL63,index.ts
Hey sorry for the delayed response, I didn't realize until just now that my notifications for questions had been turned off. I'm taking a look at some of these I missed now and should be quicker to respond in the future!
This is so weird I've never seen anything like this
(where the ordering of object keys in TS affects inference, even outside ArkType)
That might just be some artifact of StackBlitz though as locally I'm at least able to see the root types (though that intersection should be reduced)
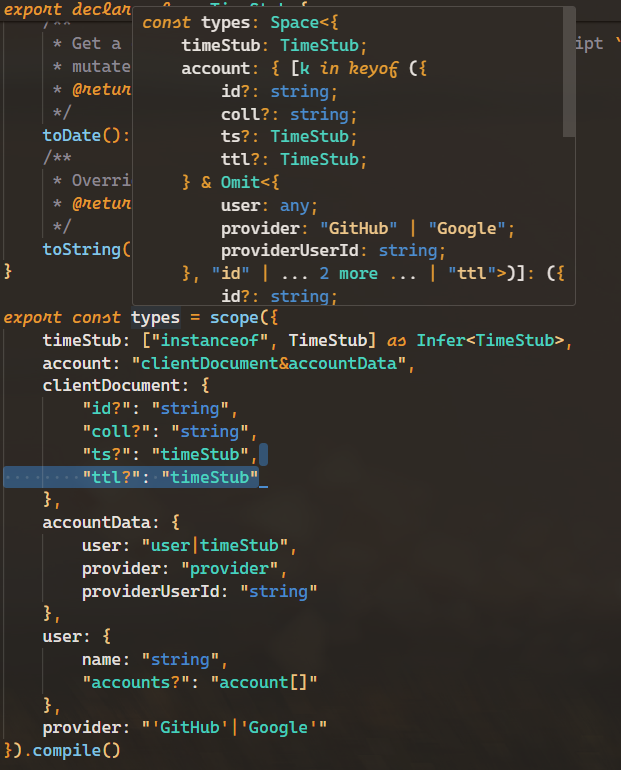
I've seen some problems with inference for cyclic intersections/unions in the past but I thought I had resolved those on alpha, I'm investigating this now as clearly alpha is not a fan of these types in general.
Well it works fine on beta which is a relief
It's also very fast on beta where something going on in alpha is really slowing down the language server for that scope
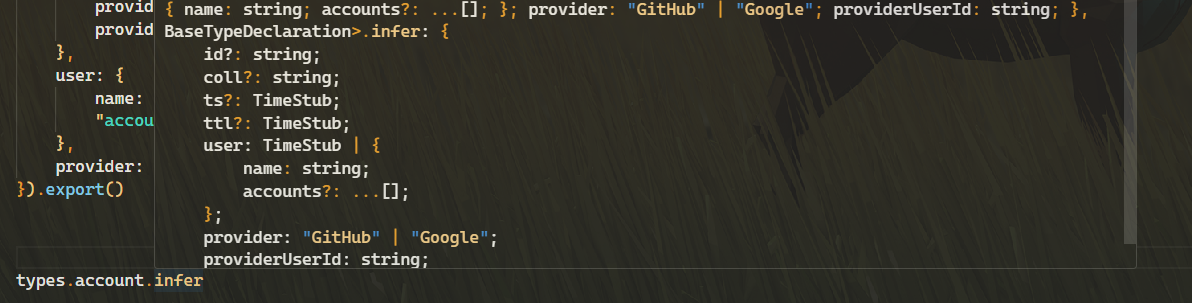
Honestly I'd love to figure out where in alpha things are going awry but given I'll be releasing a 2.0 prerelease that is mostly working in the next few days it might be better to just add your example as a test case and ensure it continues to work well there