I'm getting the following error when trying to fetch a data with relations.
I have invoice table with a id of type PgSerial, and invoiceAmenities table has invoiceId as foreign key which is of type integer.
The select query from invoice table with invoiceRelations fail stating "operator does not exist: text = integer" at the following point of the query: "invoiceId" = "invoice"."id".
The generated query with the error log is attached.
The drizzle query that I'm running is as follows:
The relevant schemas:
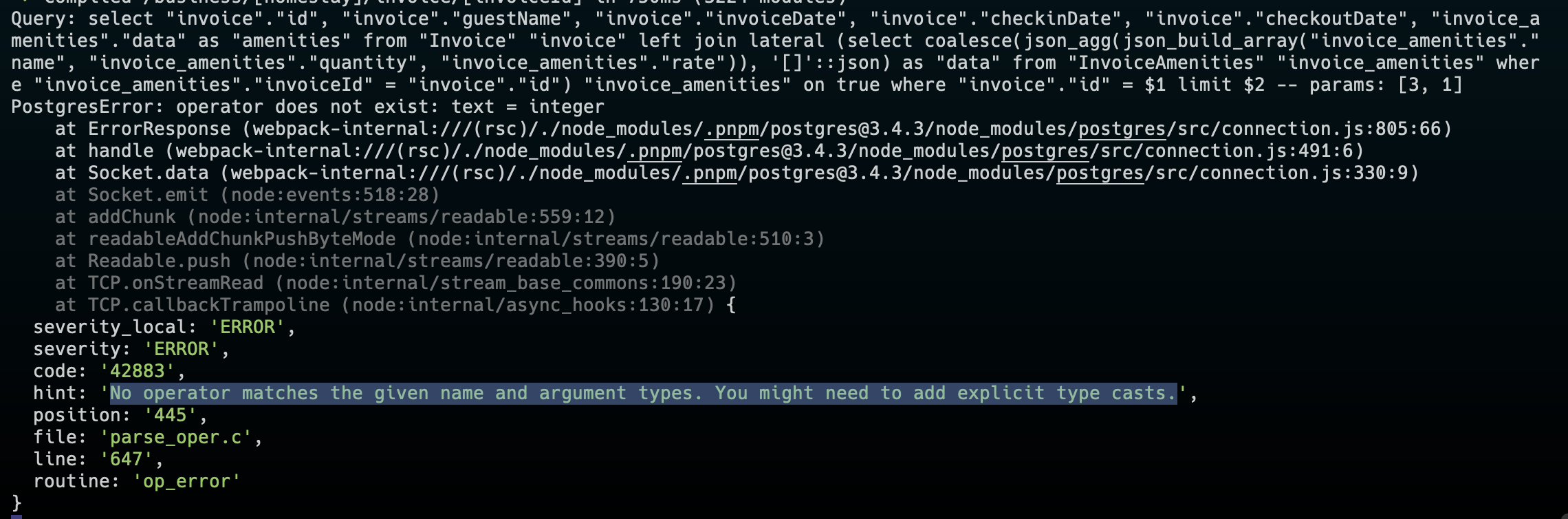
5 Replies
I tried deleting the tables and recreating them with same schemas and this is what I get(attached).
My guess is that Postgres isn't able to convert serial to integer automatically. What should I do to fix this?
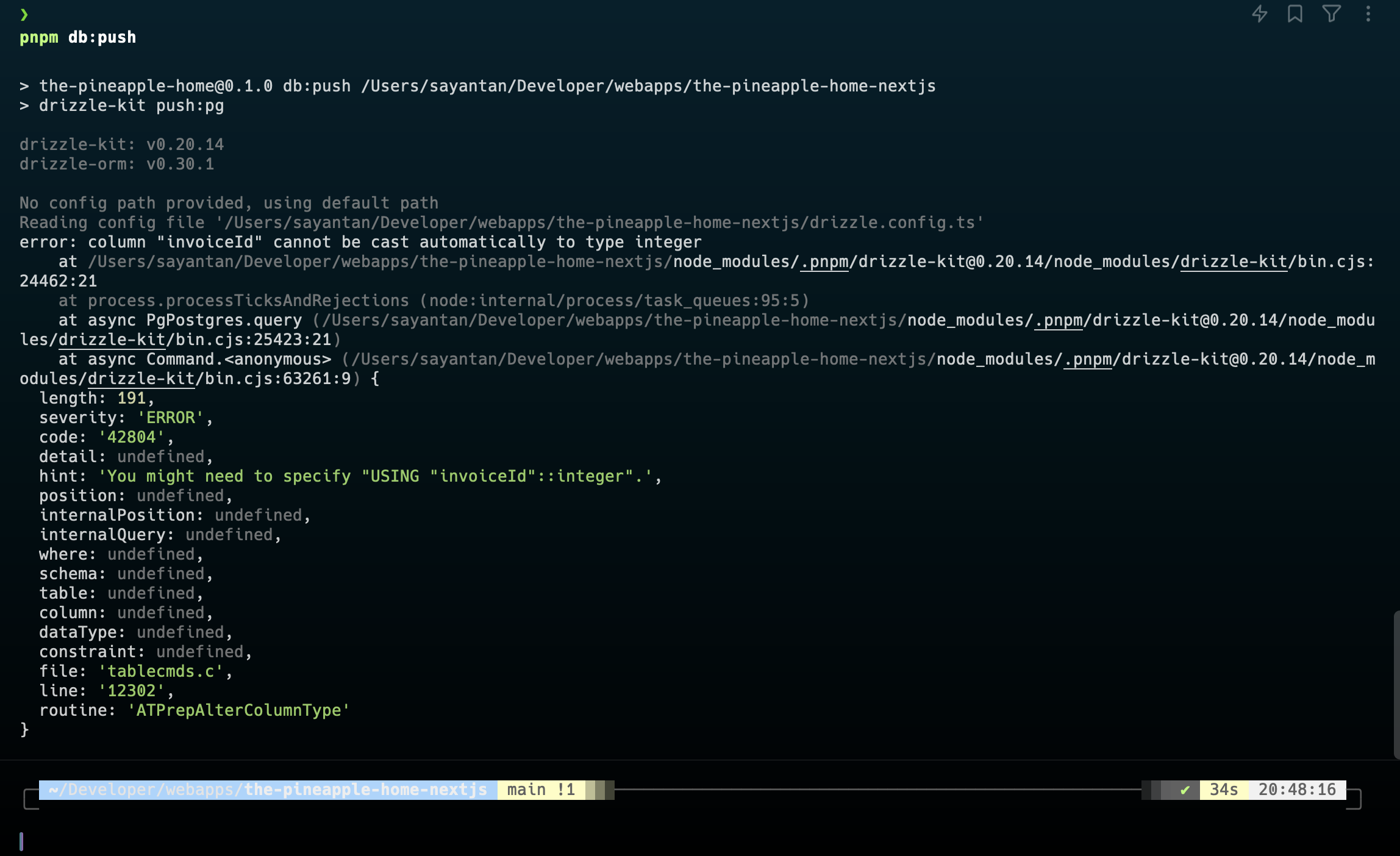
Hello, @bhoboghurey! It should work. Could you please tell me what driver are you using & provide data for this query:
Hi @Mykhailo , I'm using postgres as driver and invoiceId in this particular scenario was 3.
While debugging, I deleted the table data and then ran db:push to get the following error, it seems like a foreign key as integer type is not matching with the primary key which is of serial type.
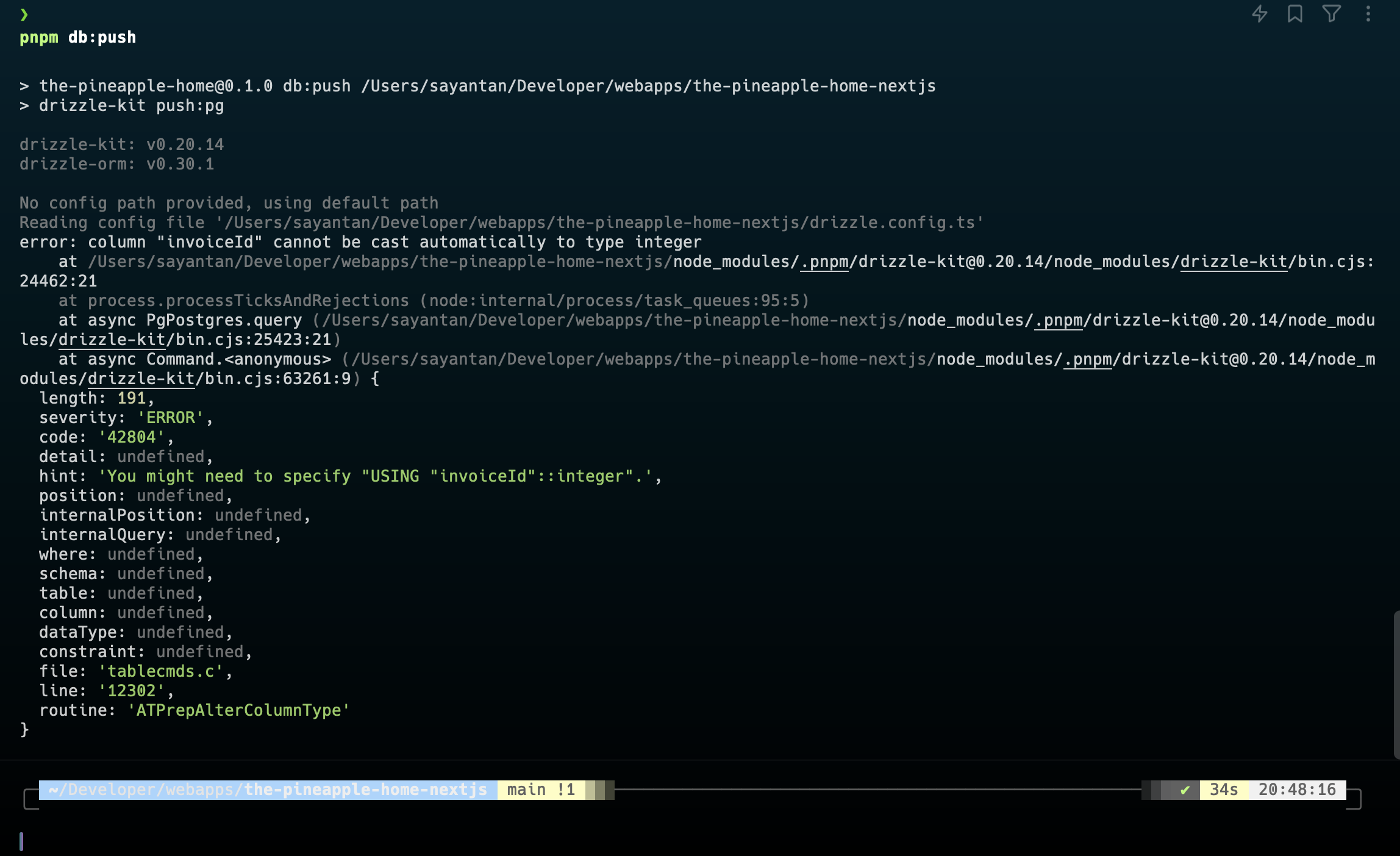
Strange, I could not reproduce the issue. Could you drop
invoiceAmenities
table and its constraints and then run drizzle-kit push
again? Or is it possible to provide reproduction repo?
@bhoboghurey You likely had a non-integer type initially but then changed it to an integer, which is why you are encountering this issue.Thanks, dropping and recreating the tables solved the issue! Not sure, how it was caused in the first place though as I created the tables using drizzle's db push only. But anyway, can proceed now. Thanks a lot.