Random Generator method
could someone help me with this method? if the list contains a number we should generate a new random number that is not in the list
28 Replies
its not ideal but you might want to create loop and loop till you find number thats not in list and then break out
and of cousrse add a limiting case so you dont get stuck in the loop forever
Also, you can use Random.Shared, rather than creating a new one.
Also, make sure you doublecheck the inclusivity/exclusivity of the bounds, otherwise you'll have a bad surprise.
Also, no need to set the result to 0 in the else, it's already set to zero.
Just FYI, if you're creating new instances of
Random()~each time, chances are you'll be getting a *lot* of repeated values
Either use a single instance of it and keep it in a static private field
Or use
Random.Shared.Next()` insteadcould you explain what this method actually does?
Shared
is a static property on the Random
class that's already initialized with a new Random()
So you can easily reuse it without instantiating it yourselfI'll try this now!
should I loop through the empty list first or should I loop through something else?
I cant find this property
What version of .NET are you using?
where could I check that?
In the csproj file for example
Or project properties
8 I believe
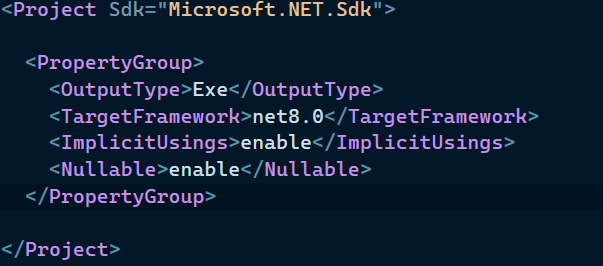
is this correct?
Random.Shared.Next()
should work, thenit wont run now

This seems to be a whole another issue entirely
The project being on .NET 8, but you not having .NET 8 SDK installed
oh I see, I need to update
I cloned it from git
and on my laptop I did update but not on my pc
thanks for explaining
Well I imagined it something like
Enter loop
Generate random number
check if number is in list
if yes add and break
if loop is repeated more than say 100 times break and do something
or alternatively you can build checker to see if every possible number was used yet
oooooor
you could make a list1 with all numbers from 0 to maxint
then remove ones that are already on list2
and then pick a random number from that list1 and insert it to your output list2
that way you'd avoid loops altogether
still wont budge

It tells you why
It's not
it's not
It's
Exactly as I said a good three times now
like this? int result = new Random.Shared.Next(minimum, maximum);
No
Read my code again
Whhere do you see
new
?
like so? int result = Random.Shared.Next(minimum, maximum);
Yes
that wont run :
'RandomGeneratorOpdracht.exe' (CoreCLR: DefaultDomain): Loaded 'C:\Program Files\dotnet\shared\Microsoft.NETCore.App\8.0.2\System.Private.CoreLib.dll'. Skipped loading symbols. Module is optimized and the debugger option 'Just My Code' is enabled.
'RandomGeneratorOpdracht.exe' (CoreCLR: clrhost): Loaded 'C:\Users\Gebruiker\Source\Repos\TheUnquiet\RandomGenerator\RandomGenerator\bin\Debug\net8.0\RandomGeneratorOpdracht.dll'. Symbols loaded.
'RandomGeneratorOpdracht.exe' (CoreCLR: clrhost): Loaded 'C:\Program Files\dotnet\shared\Microsoft.NETCore.App\8.0.2\System.Runtime.dll'. Skipped loading symbols. Module is optimized and the debugger option 'Just My Code' is enabled.
'RandomGeneratorOpdracht.exe' (CoreCLR: clrhost): Loaded 'C:\Program Files\dotnet\shared\Microsoft.NETCore.App\8.0.2\System.Console.dll'. Skipped loading symbols. Module is optimized and the debugger option 'Just My Code' is enabled.
'RandomGeneratorOpdracht.exe' (CoreCLR: clrhost): Loaded 'C:\Program Files\dotnet\shared\Microsoft.NETCore.App\8.0.2\System.Collections.dll'. Skipped loading symbols. Module is optimized and the debugger option 'Just My Code' is enabled.
'RandomGeneratorOpdracht.exe' (CoreCLR: clrhost): Loaded 'C:\Program Files\dotnet\shared\Microsoft.NETCore.App\8.0.2\System.Threading.dll'. Skipped loading symbols. Module is optimized and the debugger option 'Just My Code' is enabled.
'RandomGeneratorOpdracht.exe' (CoreCLR: clrhost): Loaded 'C:\Program Files\dotnet\shared\Microsoft.NETCore.App\8.0.2\System.Text.Encoding.Extensions.dll'. Skipped loading symbols. Module is optimized and the debugger option 'Just My Code' is enabled.
'RandomGeneratorOpdracht.exe' (CoreCLR: clrhost): Loaded 'C:\Program Files\dotnet\shared\Microsoft.NETCore.App\8.0.2\System.Runtime.InteropServices.dll'. Skipped loading symbols. Module is optimized and the debugger option 'Just My Code' is enabled.
The program '[10972] RandomGeneratorOpdracht.exe' has exited with code 0 (0x0).
nvm
thanks!
No idea what those errors could be tbh
Ah, they're just messages telling you VS is not debugging framework code
Which is expected and good
You can turn off those messages, I see: https://stackoverflow.com/a/55866842/6042255
I'll look into that
if the number is in the list I need to generate a new number that is not equal to the previous generated number
yes.
Console output:
and program ends with exception being thrown
I'm a little late to this thread, but I have multiple articles I've documented that seem relevant to this. In particular:
https://github.com/ZacharyPatten/ZacharyPatten/blob/main/Articles/2019-12-18.md
"Generating Unique Random Data"
If it is relevant and you have any questions, feel free to "@" me 🙂
@TheUnquiet