Struggling with constructor
I don't understand why I'm getting a red line under the Pet constructor
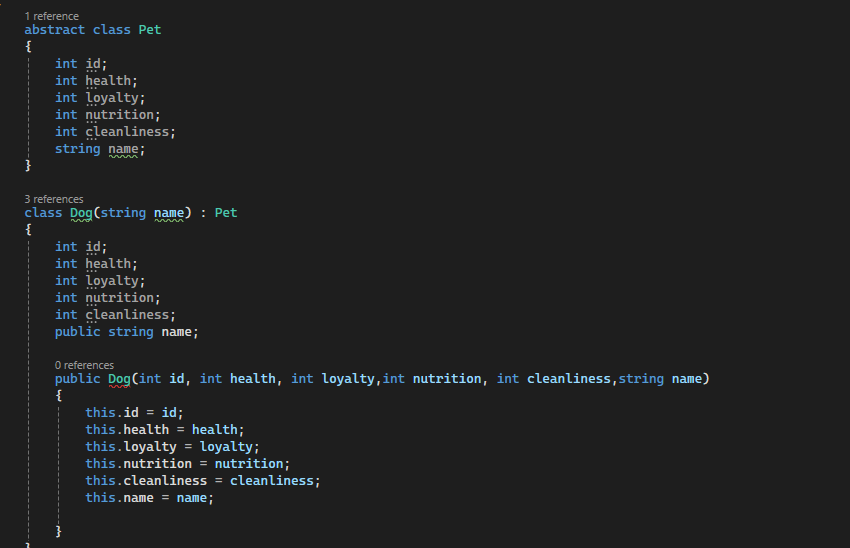
82 Replies
Can you hover over the red squiggle?

Can you paste the code here?
namespace Ponis
{
abstract class Pet
{
int id;
int health;
int loyalty;
int nutrition;
int cleanliness;
string name;
}
class Dog(string name) : Pet
{
int id;
int health;
int loyalty;
int nutrition;
int cleanliness;
public string name;
public Dog(int id, int health, int loyalty,int nutrition, int cleanliness,string name)
{
this.id = id;
this.health = health;
this.loyalty = loyalty;
this.nutrition = nutrition;
this.cleanliness = cleanliness;
this.name = name;
}
}
}
First problem that the properties are doubled
wdym
Your
Dog
class has two constructorsoh
Since you extends on Pet
so how do I fix that
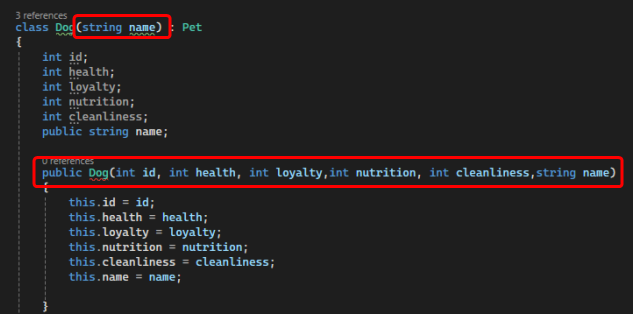
You dont need these
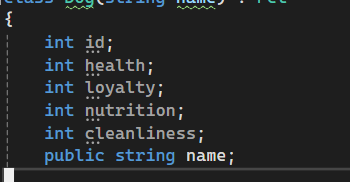
Those are fields
Regardless, the issue is because of two constructors
It tells you to call the one with fewer params from the one with more params
Using
base()
This one works fine but the id to cleanliness are null
WAIT
so have I been like
an idiot
Have you used java before c#?
python
Oh
I was imagining it like I was passing in the name and the rest were gonna be set later
But you know access modifiers?
set get?
private public
i dont know terms
There
The default is public
okay
The default is private
C# defaults to the least-accessible modifier
OMG SORRY YES PRIVATE
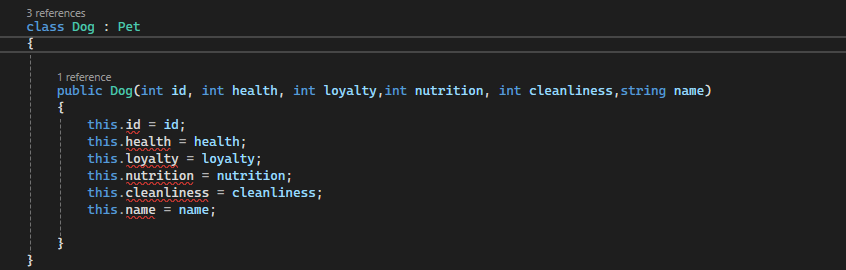
so um
In my mind im typing private 😄
Regardless, to explain the issue with constructors
is the same as
The fields of class
Pet
are private
Private to Pet
abstract class Pet2
{
public int id;
public int health;
public int loyalty;
public int nutrition;
public int cleanliness;
public string name;
}
class Dog2 : Pet2
{
public Dog2(string name, int id, int health, int loyalty, int nutrition, int cleanliness)
{
base.id = id;
base.health = health;
base.loyalty = loyalty;
base.nutrition = nutrition;
base.cleanliness = cleanliness;
base.name = name;
}
}
There
Classes that inherit from
Pet
will not have those fields
So far as Dog
is concerned, they don't exist
This is the simplest as it can get
I think
okay so is base the same as this?
Super
or is this and base diff
this = this
super = base
OHHHH
Java -> c#
okayyy
never touched java
...
Also, if it's public it should be a property, not a field
Just saying
m
so
just wondering...
how would I do that
$structure
Thats too complex for starters i think
This is the structure of a C# program
I think I understand all of that
This is how you call the base constructor
Remember that thats the simplest as it can get
I'm not the best at c# but I hope i made things clear 😄
okay so one more thing
nevermind I figured it out
thank you for the help
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Okey
Pls explain tho
@TeBeCo
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Does that mean the class itself can be the constructor?
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
okay
Thank you
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
see
$structure
for most C# usage
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
But do code conventions really matter too much if it's the style of the developer?
People like me for example use camelCase on things like functions and variables and use PascalCase for classes so there is a clear seperation
For me the key is consistency but I don't know what's right in that case
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
I see
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Does my approach sound acceptable :SCtwocats:
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Yes
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
:SCshocked:
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
I see
Thanks
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Ye
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Then what is the correct convention for classes, functions, variables and event
events*
Does the bot have a command for that.
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
What abt baking
Naming*
Not baking.
Cause I start all my events with "On"
For example
"OnClick"
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
:SCgetoutofmyhead:
Great I can refactor half my code base
Unknown User•15mo ago
Message Not Public
Sign In & Join Server To View
I see