How to use ref both inside and outside(parent) component?
I have parent component with this code:
And this is Dialog component:
So, I can open dialog from parent component because ref of dialog works as it should in the parent scope, but I can't use close() of ref of dialog element inside my Dialog component. What am I doing wrong? If I don't split this in two components then it works. But I would prefer to have this Dialog component separate and just be able to open it from a parent component.
8 Replies
I think this tutorial is relevant maybe, but it doesn't do anything with ref inside Canvas.jsx:
https://www.solidjs.com/tutorial/bindings_forward_refs?solved
U can most likely solve it by making the ref a signal instead of just a let
Interesting, thank you, I will try that
It kinda works but the code looks a bit weird to me. Maybe I'm doing this wrong? 😄 I've read some information about refs but I don't understand exactly how to think about what's happening under the hood so to say. I don't fully understand what is asigned where and when...
I've read some information about refs but I don't understand exactly how to think about what's happening under the hood so to say.it can be handy to look at the compiled output in the playground. the following code: would log
before
and not after
.
because refs as regular variables
is a common pattern in solid, they do a custom transform for ref-props: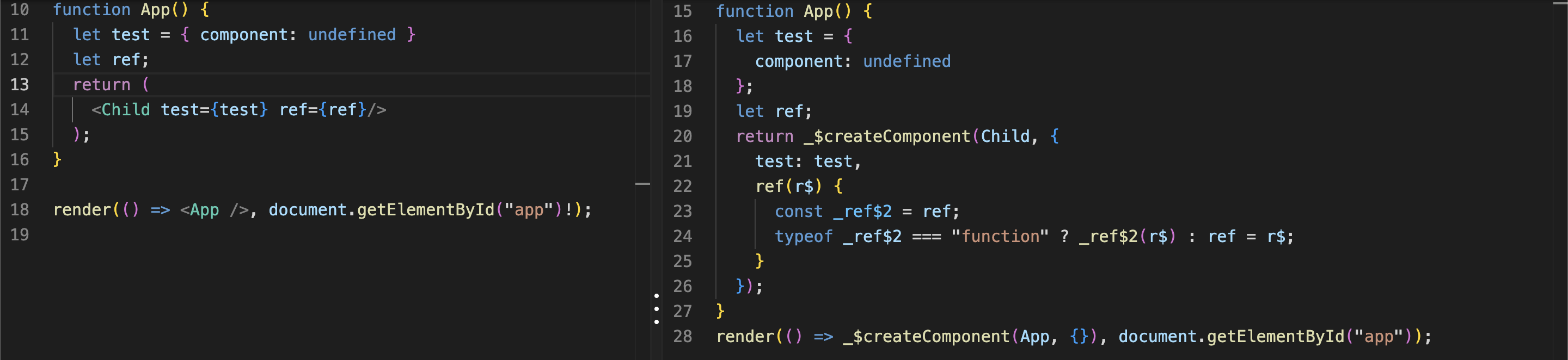
but that means you can only access the ref from the parent, and not the child
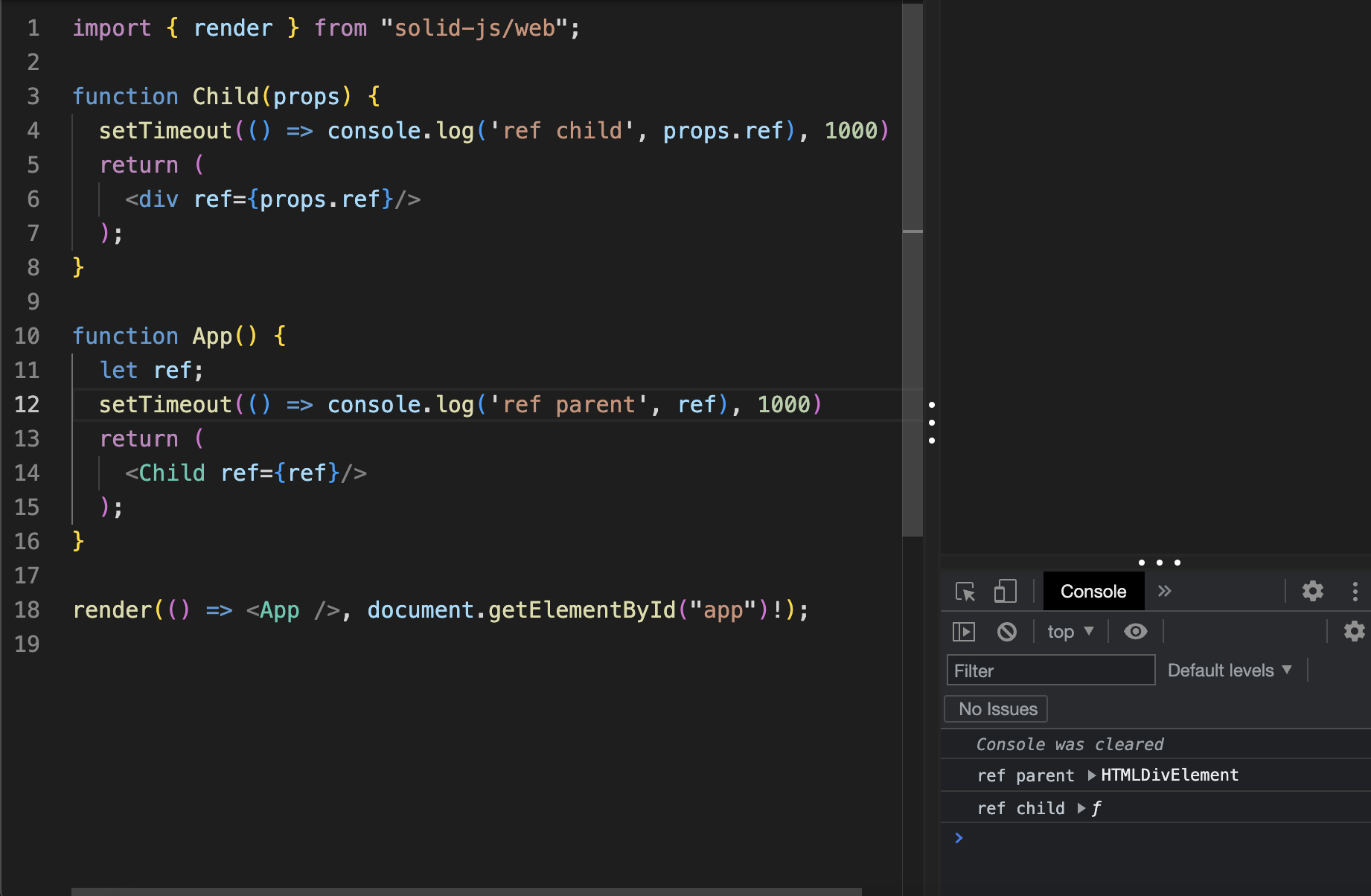
if we would not use ref as prop, but instead use another prop-name we would get the result I shown with
before/after
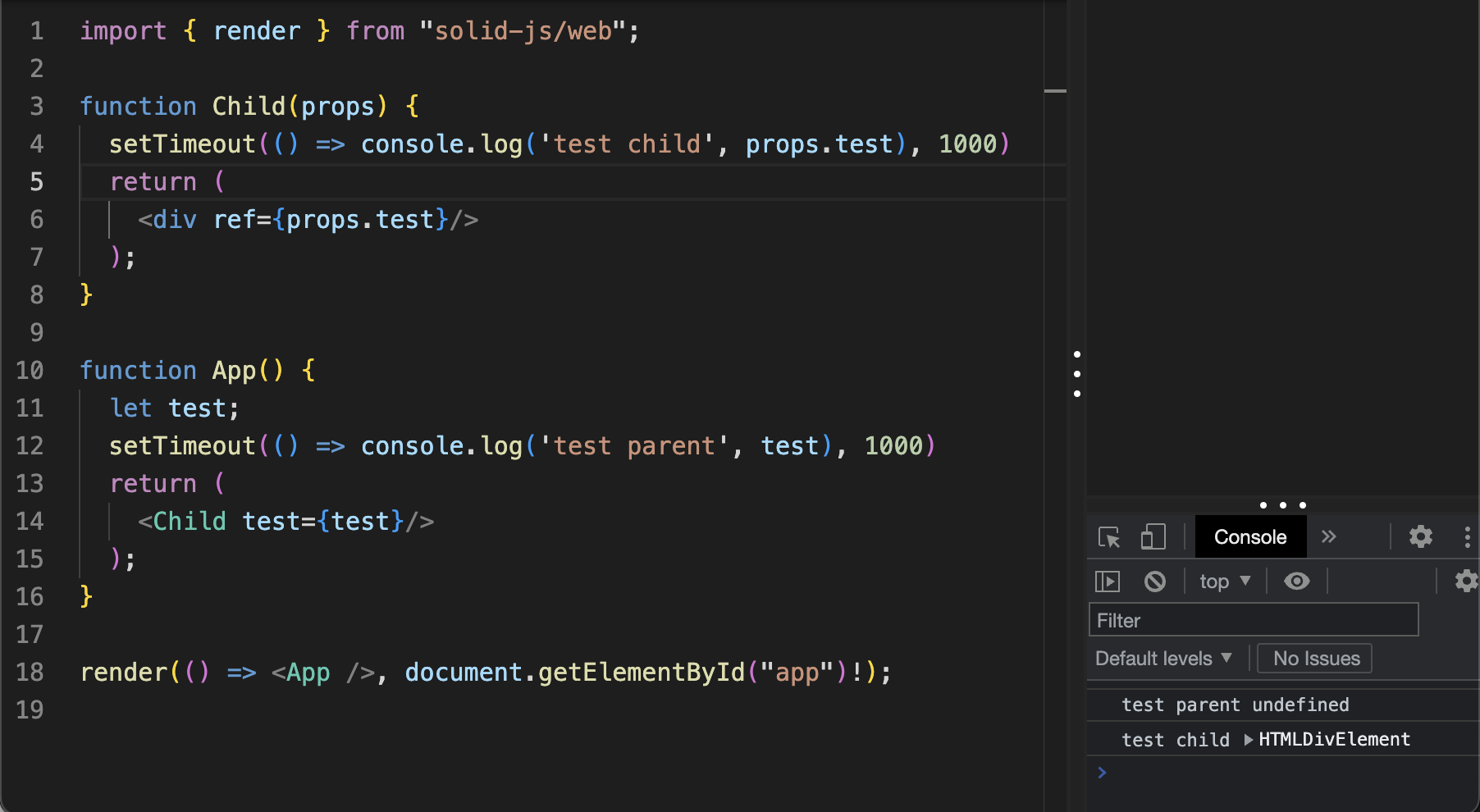
another possible solution would be to not pass a primitive, but an object
this would log
after
translated to solid's jsx:
would log parent HTMLDivElement
and child HTMLDivElement
(learned a thing or two myself while responding this question 😁 )
the props-transform is handy in 99.99% of the cases, but that 0.01 can be pretty unintuitive. if it's a good or a bad thing that solid transforms props is a topic of frequent debate in this discord.
if you don't like either of those options, you can also choose to use mergeRefs
from solid-primitives
: https://github.com/solidjs-community/solid-primitives/tree/main/packages/refs#mergerefs
probably the cleanest option