advanced relational query builder
hey, i have a use case where i need to return rows from one table based on filters requiring on connected table
here's prisma equivalent code
how would this be possible with drizzle
10 Replies
Hello, @Oreki! You can do something like this:
yeah but that is not what i want to do, in that particular example i want all the cards who have a relation to characters table and the respective character's element is lets say for example fire
ok, as I see you want to get cards with characters relation and filter by character element
here we get posts with comments relation and filter by comment createdAt
i tried that as well, only if i had a
where
key in my relational query buildercould you please provide your query code?
yes gimme a sec
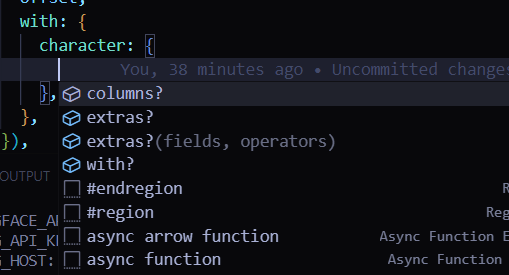
i don't get a where key for some reason
a card can only have one character, but a character can have many different cards
@Oreki got it, there was a twit with a similar problem. You have to change a bit your query, because you can’t filter character because it’s many to one.
https://twitter.com/Jaaneek/status/1749025589208519062
You had to query by character first and then look for cards because it’s one to many.
something like this, but unfortunately there is no
offset
there
Miłosz Jankiewicz (@Jaaneek) on X
@DrizzleORM Sure: schema https://t.co/mbQu9WjkP2
My current query that is working: https://t.co/0yYq6dTlrT
Query I was trying to make:
https://t.co/YRmNegpnih
Twitter
Hmm I thought so as well, thanks