Seeding data from csv file
I have a model like this:
I try to seeding data from my csv file like this:
When I run the program, I got the error:
Unhandled exception. System.FormatException: String '' was not recognized as a valid DateTime.
The format for date time for call_date
in my csv file is dd/mm/yyyy. The format for endtime
in my csv file is HH/mm/ss.15 Replies
How should I modify the Seeder to get rid of this error and have the data in csv file written in my database ?
i assume the formats you've specified for your datetime parsing don't match some or all of the values in your CSV file
specifically it looks kinda like some entires don't have dates/times specified
this is how the format in my csv file looks like, could you suggest a way to make it match ?
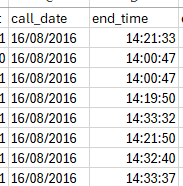
does every row have values for those columns?
yes
because
String '' was not recognized
means there is an empty string getting in there somewherethere're some records that looks like this, maybe they're realized as an empty string ?
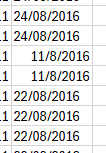
i can't tell if that's actually what it looks like or if that's just formatting in that application, but maybe
if it was me, i'd add code to check values[2] and values[3] to see if they're empty and use that to find which row is causing problems
or put the record creation in a try/catch and set a breakpoint in the catch
so I guess formating the csv file is an option, but more advisable to use some trim function better ?
you're getting ahead of yourself
we haven't established that this is actually the problem
wait we dont?
the first step is to find out for sure which rows are not working
you're guessing, and you might be right, but i'd rather find out for sure than spend time on something that ends up not being the problem
aight, i'll check as you suggest then
As you are not getting an ArgumentOutOfRangeException there is probably a line in your csv looking kind of like this ",,,, ,,,".
I would open csv file in a notepad and check if this kind of entry exists.
It would not be visible in excel but notepad will show this kind of entry
I did as you said too, and pretty much I've solved this problem by changed to just using csv helper instead. For the csv format, I download the new one and opened it in notepad too, if I open in excel, it will be mutated and the program cant read it probly anymore
basicall, i've solved my problem in this post already now