Make JavaFX Image Nearest Neighbor (every frame, multiple times per frame)
I'm making a voxel game with JavaFX as a base. No, I'm not using PerspectiveCamera, I'm making my own engine. The issue is that javafx makes images smoothed when they are stretched, which is ABSOLUTELY STUPID AND I HATE IT. You see, voxel games have low resolution textures (something like 16x16), and when you get up close and personal with a block, that could be stretched to thousands of pixels if you have a good monitor. I have tried:
- Changing requested width and height in image constructor (this kinda works, but memory inefficient)
- Setting fit width and height in image view
- Looking through javafx.scene.effects to see if there's an effect to set the smoothing to 0
- Asking chatgpt
- Searching for about 5 hours on google
- Using Canvas (PerspectiveTransform doesn't work, so no good)
- Using java swing BufferedImage
- Looking in the javafx code
Some things that would work but are too slow to be run many times per frame:
- Pixel Reader/Writer resizing
- Loading image from disk with different RW+H
- Loading image from disk to byte[], then using ByteArrayInputStream
- Processing every pixel with canvas
I feel like I forgot one or two things in those lists, but those are enough things for you to (hopefully) understand my frustration. I don't really want to use external libraries as I want no ties to anything other than JavaFX, but if there is no other way, I can do it.
After writing this, I feel like I forgot to clarify what I mean by "smoothing". Image 1 is what I want it to look like (done with RW+H, but that's inefficient), and image 2 is what it looks like normally.
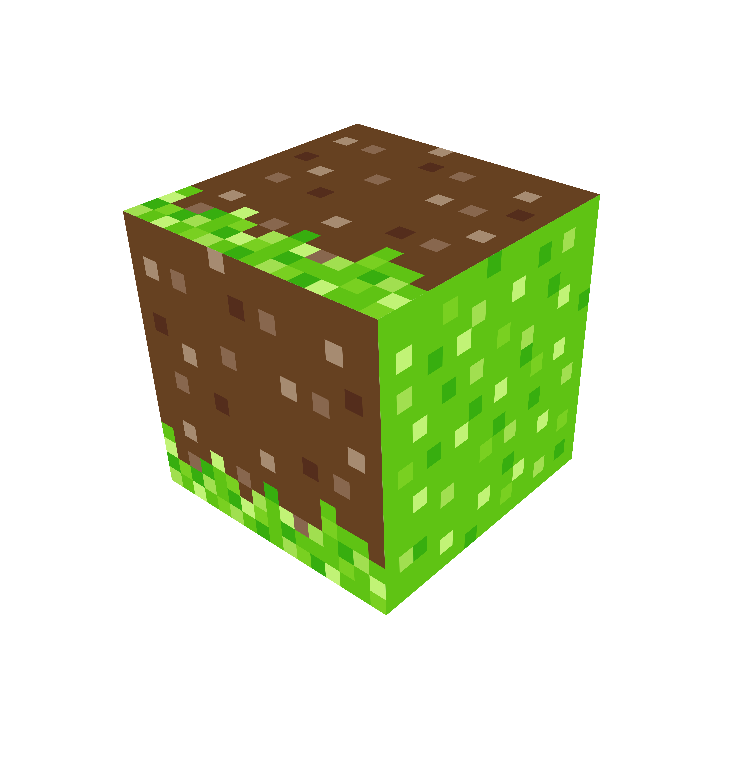
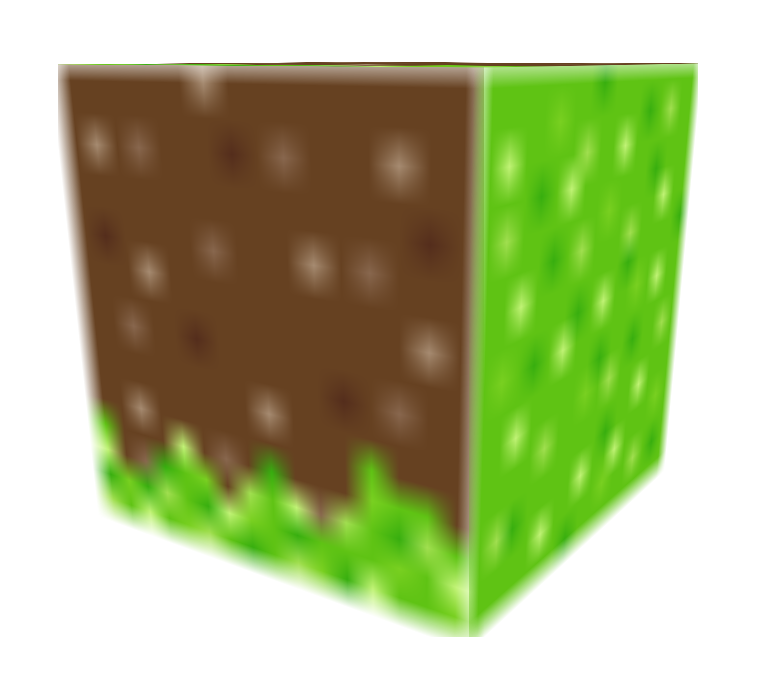
89 Replies
⌛
This post has been reserved for your question.
Hey @The Typhothanian! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
Yes, I know that the textures are rotated wrong and are on the wrong faces, I'll fix that later.
What are you rendering with, trianglemesh?
and phongshading?
Neither, as I said, I am using my own engine
That's adding an image view and setting the effect of the IV to a PerspectiveTransform
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
How do I do that?
Sooo, using Graphics(2D)?
Wdym
GraphicsContext2D?
If so, then no.
Hello?
Show me some drawing code
So I can figure it out lol
K
Projection code:
if you format them, after the 3 backticks, type java so you have syntax highlighting
Like how? just put java after the backticks?
yeah
You can even just edit your pasted code
much better 😉
There
So, that is the projection code
Yeah seems normal
I just call the project method to get 3d to 2d
Where's the drawing code?
That
is approximately 500 lines
and is not needed for this, so I'll just explain the important parts
yeah, but your projection has nothing to do with the smoothing...
That's all in the drawing code
import static java.lang.Math.*;
also gross lol 😄
I hate static importsWell I don't have the old simplified version
I have two Math classes
one sec im typing
I keep pasting but it becomes a text file, that okay?
sure
Hmm, you can't view that in discord
Hold on
Affine (JavaFX 21)
declaration: module: javafx.graphics, package: javafx.scene.transform, class: Affine
You do know that exists?
I have absolutely no clue what that is
Well, too late for that to help me. Wish I found that two weeks ago
It took me two days to make that projection matrix
Anyway, here's the important part of the draw code:
Time for a refactor 🙂
ProjectedFace
Can you send that one?
hold on
The P3D[] is 8 points, and those absolute points are after it has been moved and rotated and such, don't need that part
ProjectedFace is just an ImageView with extra stuff for block placing/breaking:
Ignore the getOutline method
aaah, that's an imageview
ok
I have no clue why I have to do 2, 3, 0, 1 instead of 0, 1, 2, 3, but whatever
ok, in the constructor of projectedface
add
setSmooth(false);
And see if that worksTried that didnt work
What does that do?
setSmooth?
yeah, what does the output look like if you do that
No difference for true or false
At least, not that I can see
Ah yes I have claimed the 69th message (this is it)
Yeah, unfortunately imageview does not allow nearest neighbor filtering
And that's stupid
Do you know what method smooths it, so I can override it in my projected face and be done?
None really, the property sets something internal in the rendering engine
UGH
lemme check rq how it works
Yeah, back when I was trying out the PerspectiveCamera thingy, I got around this by setting the diffuse color to black and setting the SIM to the image.
That screwed up lighting but I wasn't planning on using the built-in light engine anyway
Then I decided to make my own engine and have it super optimized for my game
My idea, grab a canvas, grab it's graphics
This is the full render of the underlying imageview
Seems easy enough to just do that drawing by hand
Protected means I can't override it, right? Or is that final?
And this is the draw for that coords thing
That's in a different class
NGImageView, can't get to that
So the render content is in IV?
nope, NGImageView
absolute displeasure
Yeah, image views are not meant to render textures
Plus you're only getting an affine transform
And the draw method is where?
I believe that will give you PS1 textures 🙂
that's what
coords.draw
does in the first listingFirst listing?
in rendercontent
Okay, I'm getting confused. What are you suggesting again?
I got 26 min then I have to go
So, grab a canvas, grab it's graphics, and do the draw manually
GraphicsContext2D?
Although that might not be enough
I think if you really wan't a 3d engine, javafx is not going to cut it
Unless you're happy with the smoothing
Oh, it is going to cut it as LWJGL is impossible to work with
Lets see...
lwjgl is just opengl, it's not that hard
It is if there's no tutorials at all
There's always the trick of just scaling your image 16 times with nearest neighbor in a photo editor and using that
the smoothing will be less
as you already start from a larger image, so less scaling is needed
Yeah, but 1. Takes up too much memory and 2. can still be blurry when close up
yeah
And I can do that in image constructor
Hold on, lemme implement the canvas thing and i'll get back to you
It's more for 2d drawing
whatevs
What's this ImageObserver thing I'm seeing in Graphics.drawImage?
tjoener?
nvmd was using wrong class
I have that implemented, but I'm just seeing a white screen?
should render fine, I've done canvas before
Might need to reposition things
hmm
I'm stupid, the canvas is 0 by 0
ARGH the canvas gc doesn't have x1 y1 to x2 y2, it has x y w h
And I have no clue how to do the transform thing
like I said, might not be the best tool for the job
Hmm
Well, in LWJGL, I had the exact same issue. Do you know how Minecraft fixes it?
And I gtg in 2 min
with the fuzzy rendering?
Yeah
Maybe it could give me a nudge in the right direction
Yeah, there's a shader calculation you have to make
oh great
or GL_NEAREST
but that might not be enough
Welp, I know exactly what I'm doing tomorrow. Figuring out an efficient algorithm to replace PT.
It feels like only two weeks ago I had to make a complex mathematical program for my game
Oh wait, it was two weeks ago
Well, gtg.
ill post the code here once i figure it out, dont close the thread
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
uh huh i know
Yeah, if you thought you could do game programming, or writing your own engine with only a little math, boy do I have news for you
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
I'm almost there
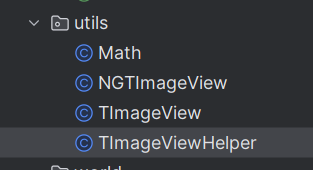
I tried canvas but it was too laggy for just two blocks
I cannot express my supreme amount of disappointment.
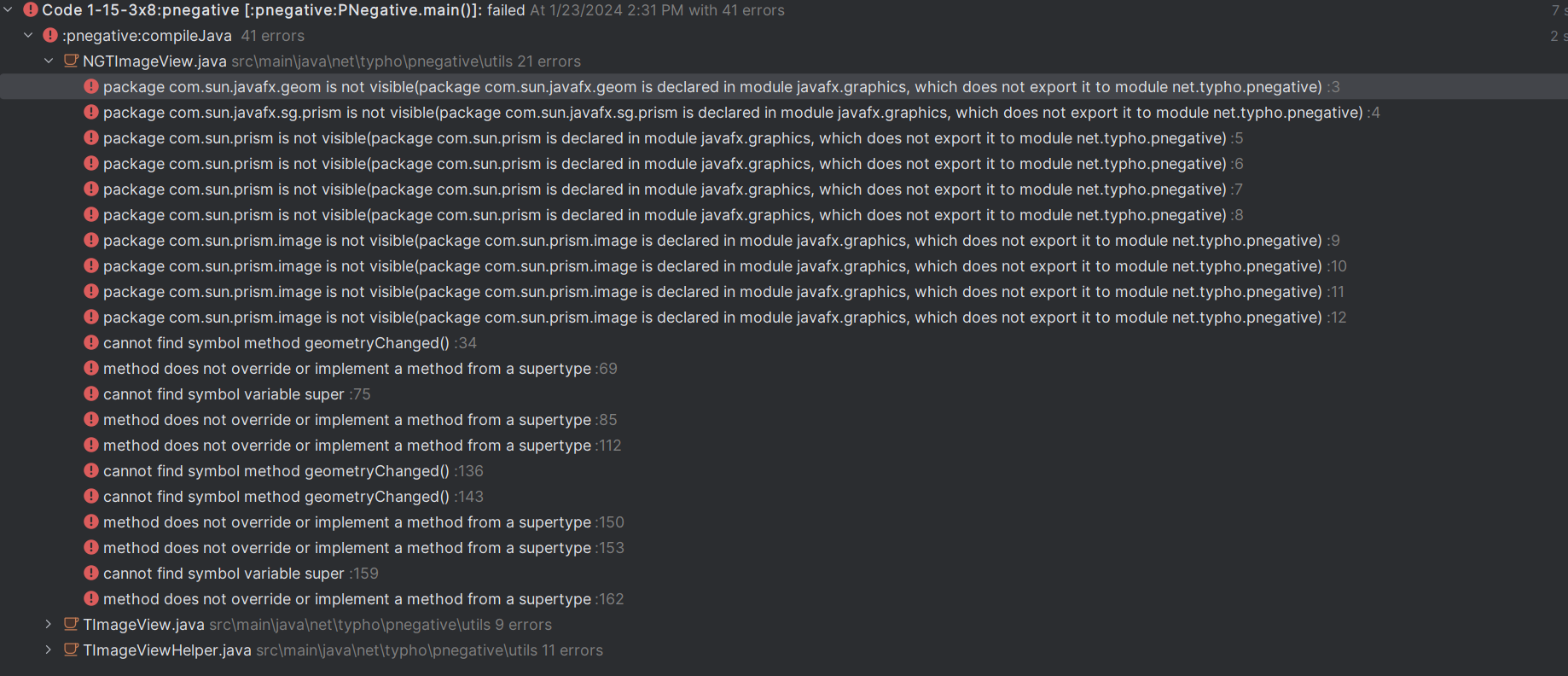
@tjoener Do you know how I would go about making my own window manager? I just need mouse and keyboard input, and direct access to each pixel on the screen.
eeeeh
I have no idea what you're actually trying to make
Me neither
But, like I said, the canvas is too laggy, so I want to try making something like GLFW.
Screw it I'm using GLFW
use lwjgl then at least
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.