Need help for ContextMenuCommand
I have the below code:
And it errors on the
part.
What's the cause and how to fix it?
28 Replies
What's the error?
Let me grab it real quick
That's all
It still doesn't runs.
@Helpers
Solution
@Jarvo
And you also have this error:
As an aside, what makes your errors formatted like that if I might ask? That's not how
tsc
formats its errorsOoohhh. Damn. Thanks
thats literally tsc
why is it json 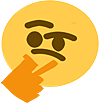
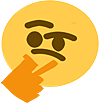
oh
looks like LSP response ngl
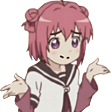
And you also have this error: - .setColor(User.hexAccentColor) + .setColor(User.hexAccentColor ?? null)I already got that fixed. And from where do I import
UserContextMenuCommandInteraction
@vladdy?discord.js
Okay
It's saying
Application did not respond
Defer before doing anything else. You're likely not responding within 3 seconds. Also the second parameter is superfluous, interaction.user is the default. Constructor is also superfluous, you're not changing anything versus the base constructor.
Okay I am not that good, but I don't understand what you're saying.
I have to defer it... Why? Because at other commands I also work with the same PaginationEmbed
presumably you're not responding within 3 seconds
between fetching a member and all the fields you're adding with all the data you're adding that can easily run into the few seconds.
(not really but when you add all latencies up, it can happen)
Ah.
How to register handlers? Like the buttonhandler?
I have the buttonhandler in a file. The content is:
https://www.sapphirejs.dev/docs/Guide/interaction-handlers/what-are-they
Also please create new threads for proper question tracking.
Sapphire Framework
What are they? | Sapphire
These are interaction handlers! A simple class you can extend to handle almost all the interactions you may receive in
Thx
Okay I am gonna re-open this because it still won't send...
I have the .run(interaction) at the bottom and I don't why it still won't send.
@Favna
Here's the code again:
You're returning early, as helpfully stated by ESLint as well
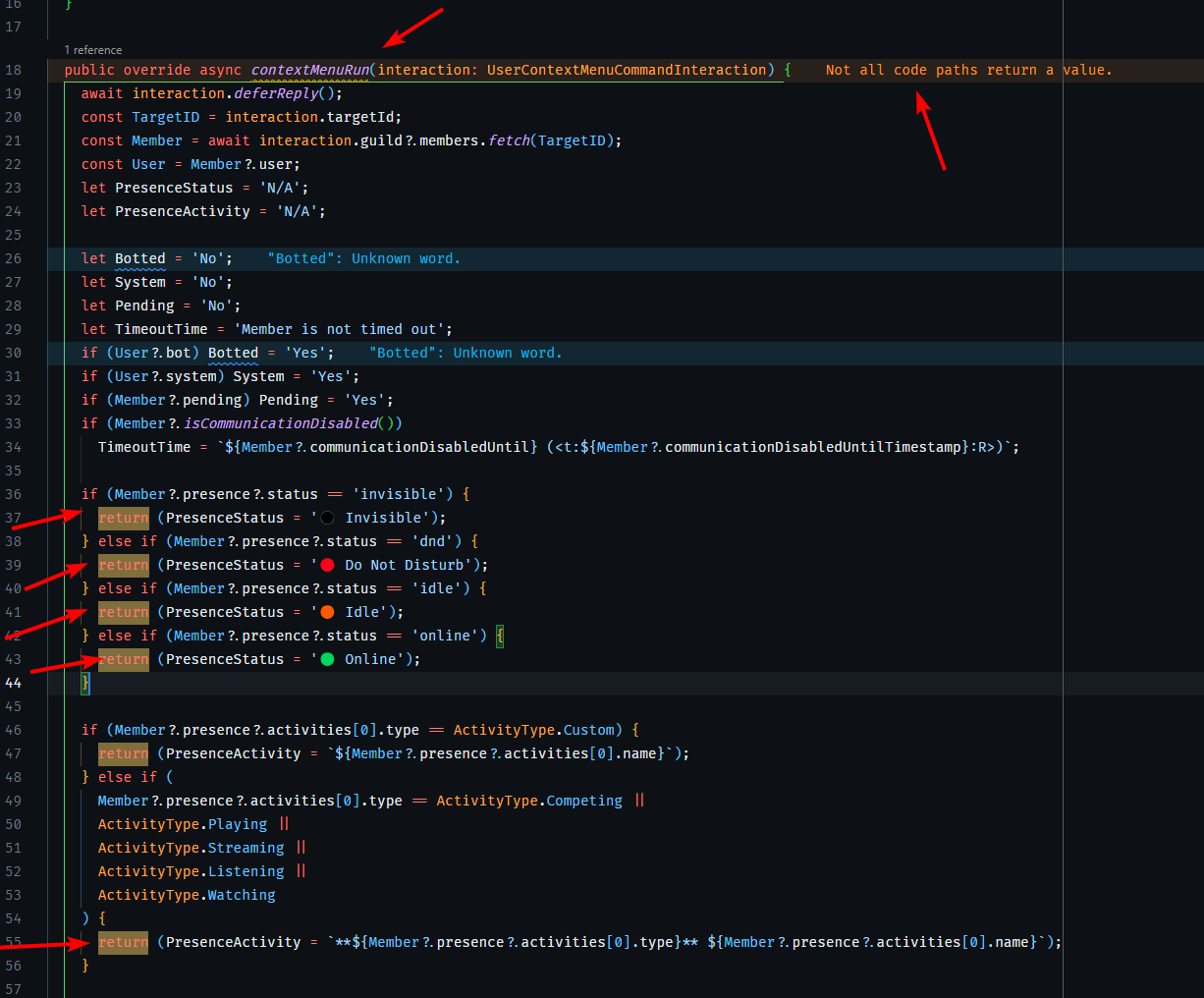
What's with the first line of code?
it's where the eslint warning shows up
because it's the function
Not all code paths return a value?
I got the info from https://www.sapphirejs.dev/docs/Guide/getting-started/creating-a-basic-context-menu-command
Sapphire Framework
Creating a basic context menu command | Sapphire
This section covers the absolute minimum for setting up a message context menu command. We have an entire
I don't know how I can explain this in another way mate
returning from the function before you call
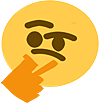
new PaginatedMessage
etc
that code never gets executed
You either shouldn't return at all there, or you should analyze which return is being triggered and make sure it doesnt
this really is JavaScript for beginners teritoryGot it fixed.