Exam Prep OOP(Constructors , Inheritance , interface , polymorphism , Exception Handling
topics i will be covering
inheritance
constructors
static
sealed for both methods and classes
polymorphism
interface
Exception Handling
Overload
Override
Abstract
sorry for the the long list
141 Replies
hello so lets start with exception handling
so for try
i only write the code
which might cause an error
for catch i write the type of error i expect to happen and what should i do when i encounter such a problem
for finally i write what i want to pass regardless of errors or not
try can have a catch but no finally
and can have a finally but no catch
is all of that correct
Correct
but you cant have catch and finally without try
You can have
also i have another question
if i did 3 catch cases
it will only choose one
and skip the others
even if they are the same catch exception
You can always try and see
Angius
REPL Result: Failure
Exception: CompilationErrorException
Compile: 368.365ms | Execution: 0.000ms | React with ❌ to remove this embed.
how do i use this
what if i dide exception first
then smaller exception later
it wouldnt cause an error right
but only the first catch
would activate
The easiest way to check is... try
Use $csharprepl
$repl
(Did you mean
!eval
?)
MODiX REPL (server): https://github.com/discord-csharp/CSharpRepl
Command line interface REPL: https://github.com/waf/CSharpRepl
https://media.discordapp.net/attachments/569261465463160900/1151055959444160582/image.pngGitHub
GitHub - waf/CSharpRepl: A command line C# REPL with syntax highlig...
A command line C# REPL with syntax highlighting – explore the language, libraries and nuget packages interactively. - GitHub - waf/CSharpRepl: A command line C# REPL with syntax highlighting – expl...
Or go to #bot-spam and use $eval
Compile C# code in #bot-spam, use
!eval
Example:
$csharprepl
Or use Sharplab
if i write a code after catch
not a finally
but a code after catch
and catch has been caught
does it execute that code too
$tias
You should really look for some environment to run C# snippets and just test those questions.
If you get stuck or don't know how to do that, someone here is probably willing you to help getting started.
is there an online compiler
Like Sharplap or one of the other recommendations by ZZZZZZZZZ
I prefer the terminal repl, but yeah, Sharplab is an online option
There are multiple: dotnetfiddle, replit, sharplab apperantly can run code too somehow.
Or just install .NET 8 SDK locally, install VS or VS Code, and go to town ¯\_(ツ)_/¯
Which is probably the best way to go about that in the long term, since you will need that anyways if you wanna work with C# in some capacity.
thanks i learned alot
hello can someone explain interfaces
so interface is like a contract
i put in it abstract unimplemented methods
and a class can have multiple interfaces implemented and the same interface can be implemented by other classes
is that correct
but what i want to know
can the class leave the methods as abstract
Yes that sounds about right.
Do you know under which conditions a method can be marked abstract in C#?
it doesnt have a body
joschi what happened to ur colour 😦
My color?
anyway continue
under which conditions
No that is what it means to be abstract.
My question would be: "Is the following valid C# code?"
no
class isnt abstract
if i want to implement the method then the class either has to be abstract
or add body to the method
is this correct
Yes
another question
you know the may be null error
Let's keep at your original question for a second.
Can an abstract class implement interfaces?
hmmm
they both do the same song
thing
so i would say yes
is that correct
guess
Yes that's correct.
interfaces cant do : classname right
only classes can implement interface
not the other way around
No an interface cannot inherit from a class
ok
can it inherit
from another interface
An interface can implement another interface
So
ok
thats good to know
is that all when it comes to intefaces
i cant declare smth static
inside an interface
In C# 11 and .NET 8 you can
damn
There are also default implementations.
But I think they are a bit frowned upon.
yeah dw my professor wont bring them
You can do
her slides from 2009
ok
good to know
so yeah this is all when it comes to intefaces
Yes, pretty much
what is the when clause
in catch blocks
doesnt catch automatically catch an exception
if it occurs
whats the reason for the when
Could you give an example?
ok
try {int x = Convert.Toint32(Console.Readline());} catch(format exception fe) { console.writeline("hi);
if i enter hello
in the x
i will get an exception
format exception
FormatException
so the catch will be triggered
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/Just as an example for statics on interfaces and how to use them.
thanks
anything in interfaces are automatically static and abstract though right
except when i do default
so static and abstract in interfaces are redundant
correct?
No nothing in an interface is automatically static!
They are implied to be abstract.
But you explicitely need to add abstract for static members.
ok
You would mostly use
when
clause when you're doing something like this
Let's say you have an exception that has a property "ErrCode"
You can do
ok
so the class errorcodeexception needs to first have
a property
like that
to justify using when
Yeah you would need to "check" for something to justify using them
So in that case we were checking for the value of
ErrCode
cause its abstract it needs to be implemented
but the 2nd one isnt?
No, the second one is not abstract.
This is probably mostly because of older language limitations, where you could not have statics defined by an interface.
Why don't you try to do the following:
1. Implement IHaveStaticMembers in a class.
2. Create a method that uses both members defined by IHaveStaticMembers.
ok
When you have a static abstract member in an interface, you have to implement it in the class that implements the interface
When you don't have abstract, if the static member is a method, you HAVE to provide an implementation for it in the interface itself
lol
my version is c 10
i cant update it rn
power outage
barely any internet
ok thanks
now for another question
if you guys want to chill its ok
i asked a lot of questions
you can answer this in your free times
now
why do i have may be null errors
on string and objects
and can checking for null
remove all these errors
like
if (employee != null)
Could you send a full example, where you face the issue and can't resolve it?
ok let me bring you
If you have for example this class
And we create an instance of the
User
class isn't it possible for Name
to be null
?true
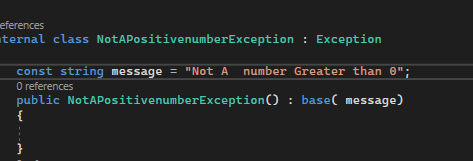
if i remove the const
its an error
ik why
const means this cannot be changed
so 0% of chance of it every getting modified to null
Nullable reference types allows you to give the compiler a hint on whether it can expect this refenece instance to be null or not
So
The
?
after the type string
gives the compiler a hint that yes, Name might be null
So when you use it for example
The compiler would warn you that hey, it might be nullNo that's not why.
What does the error tell you if you remove the const?
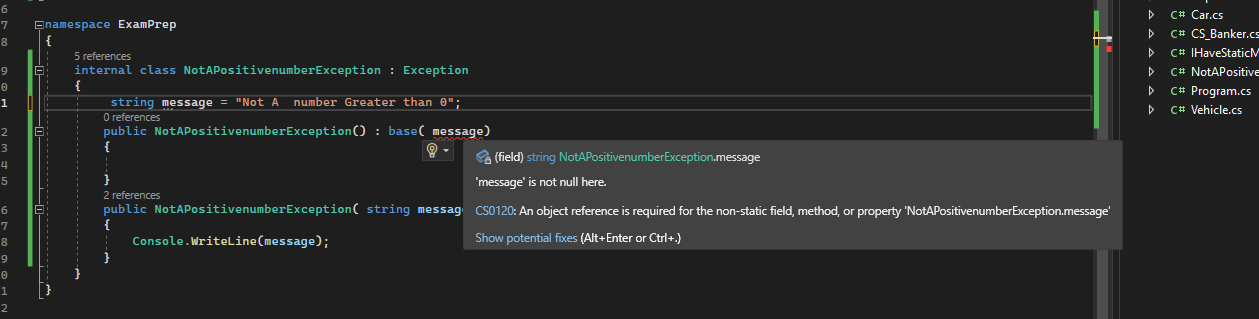
message is not null here
why he wants it to be null
That's different
It's not that it wants
null
That is not the error. The Error is the line below
It's first saying, that here
message
field is 100% not nullok but if i remove the text
then it will say
it may be null
right
if i dont initialize it
No, you can call the base consturctor for Exception without passing any message
yeah
But your error there has nothing to do with nullability
damn fr?
thought it was
ok why does const
remove it
Read the full message. What does it tell you below "message is not null here"?
object reference required
Basically what's happening is this
message
is an instance field right?true
A constructor creats the instance
so i need to use object.message
to use it
right
But doing this
Means call the base constructor before calling my constructor
So the instance is still not "created"
const makes
message
be on the type itself
Hence why you can do
not quite understanding
Okay let's try something different
How would you access
MyStatic
If I am accessing it
Inside myclass
It would be MyStatic
If it's in another class
It would be MyClass.Mystatic
Is this correct @Kouhai /人◕ ‿‿ ◕人\
Yes, that's correct
So where does MyStatic "live", it lives on the type itself right? @Gonzo
Yeah it's not an instance
It's not tied to a specific object
Exactly👍
When you make a field
const
It also lives on the type itselfOooh
Thanks
hello
another question i have
are null errors
I assume you mean warnings?
yeah
why do they warn me
if smth may be null
like i know bro
its a data type which might be null
like string or object
whats the best way to avoid null errors
just say if (objectname != null)?
Can you show a code sample where it warns you, because it depends on the context whether you want to the reference type to be nullable or not
Noll errors are usually not about "this can be null" but rather, "this can be null but you're not handling this eventuality" or "this can be null, but you're passing it somewhere where it cannot be null"
how do i guarantee 100%
my code is free from null errors
do checks for null
using if?
Yes you want to make sure that nulls are always handled somehow.
If possible gracefully, or you will run into null reference exceptions. Which can be really annoying and sometimes hard to reproduce.
Nowadays, you would have nullable reference types enabled for the project
So it shows you warnings whenever there is some issues with null
And you have plenty of ways to deal with it. Starting with an
if
of course, through pattern matching, null-coalescing operator, null-conditional calls, all the way to more rare ways like null-forgiving operator or specific attributessup guys
if i want to use a method polymorphically
i first have to check if the superclass has object type
of the derived class
for example
animal isnt the class
its the reference
to the object
Nowadays, you would use pattern matching
animal is Dog
Angius
REPL Result: Success
Result: bool
Compile: 240.167ms | Execution: 24.841ms | React with ❌ to remove this embed.
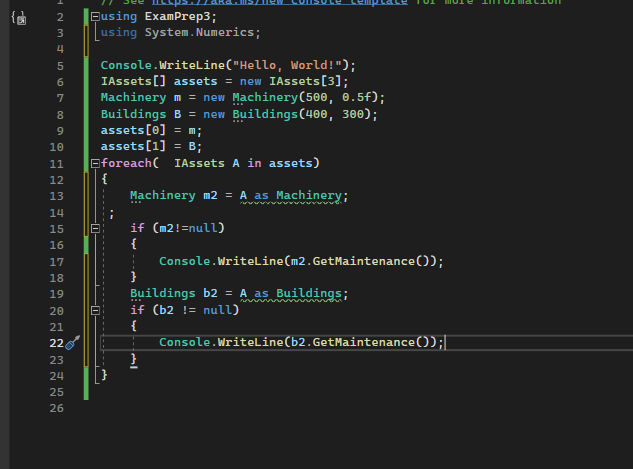
@ZZZZZZZZZZZZZZZZZZZZZZZZZ
there is no relationship
between machinery and buildings
would this output exactly how i would want it
They both implement
IAssets
though, don't they?Angius
REPL Result: Success
Result: bool
Compile: 277.841ms | Execution: 24.729ms | React with ❌ to remove this embed.
yes
they both implement them
Then you can easily use pattern matching
Pattern matching with a cast, even
will this avoid exception errors
i want to see what what will avoid
exception
Well, you are guaranteed that
b
here will be a Bar
and not a Quz
, so it will avoid exceptions connected to thatif i want to test methods
then i will do
lets sa
b.Genericmethod();
right?
Yep
is there a way to use as
like this tho
since as will never throw exceptions
I guess you can use it similarly
as
will either cast to the desired type, or return null
if it cannot
So check for null and you're good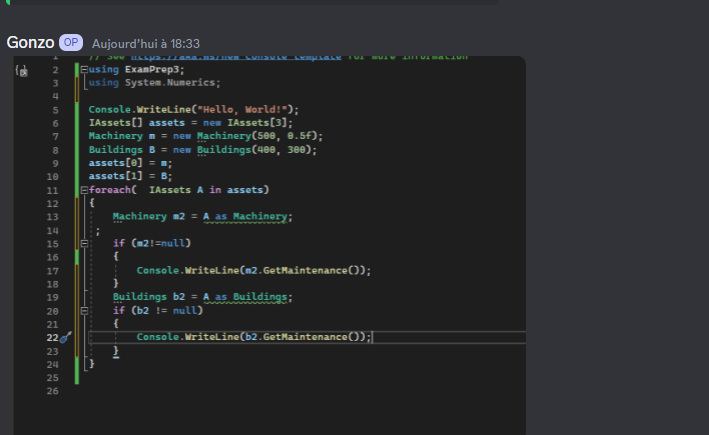
is this a correct implementation for as
Close,
m2
should be of type Machinery?
(remember how as
can return null
?)
Same for b2
, should be Buildings?
ok
so just do the ? at the end
to i guess
give the fact
that it could be nullable
Yep
T?
is a way of saying T or null
its like saying
a territary operator
where the true output
is T
and false output is null
Ternary, but yeah, I guess you can look at it that way
Console.WriteLine("Hello, World!");
int x = Convert.ToInt32(Console.ReadLine());
try
{
if(x < 0)
{
throw new OutofBounds();
}
if (x > 1000)
{
throw new OutofBounds();
}
} catch(OutofBounds ob) when ( x < 0) { Console.WriteLine("hi"); } catch(OutofBounds ob) when (x > 1000) { Console.WriteLine("Hello"); } hello will this code access
} catch(OutofBounds ob) when ( x < 0) { Console.WriteLine("hi"); } catch(OutofBounds ob) when (x > 1000) { Console.WriteLine("Hello"); } hello will this code access
Access what?
if i enter an x less than 0
will it access the first catch only
or is my implementation incorrect
Try and see
my visual code studio has a problem
it doesnt debug
tried on sharplab
but it doesnt recognize
try and catch
only tryblock
and some catchblock
$vscode
Follow the instructions here on getting started with DevKit for C# in VSCode: https://code.visualstudio.com/docs/csharp/get-started
Get started with C# and .NET in Visual Studio Code
Getting Started with C# and .NET Development in Visual Studio Code
Make sure you install the appropriate plugins
Or better yet, that you install Visual Studio 2022 instead of VS Code
Or if you are a student you can get all JetBrains IDEs for free.
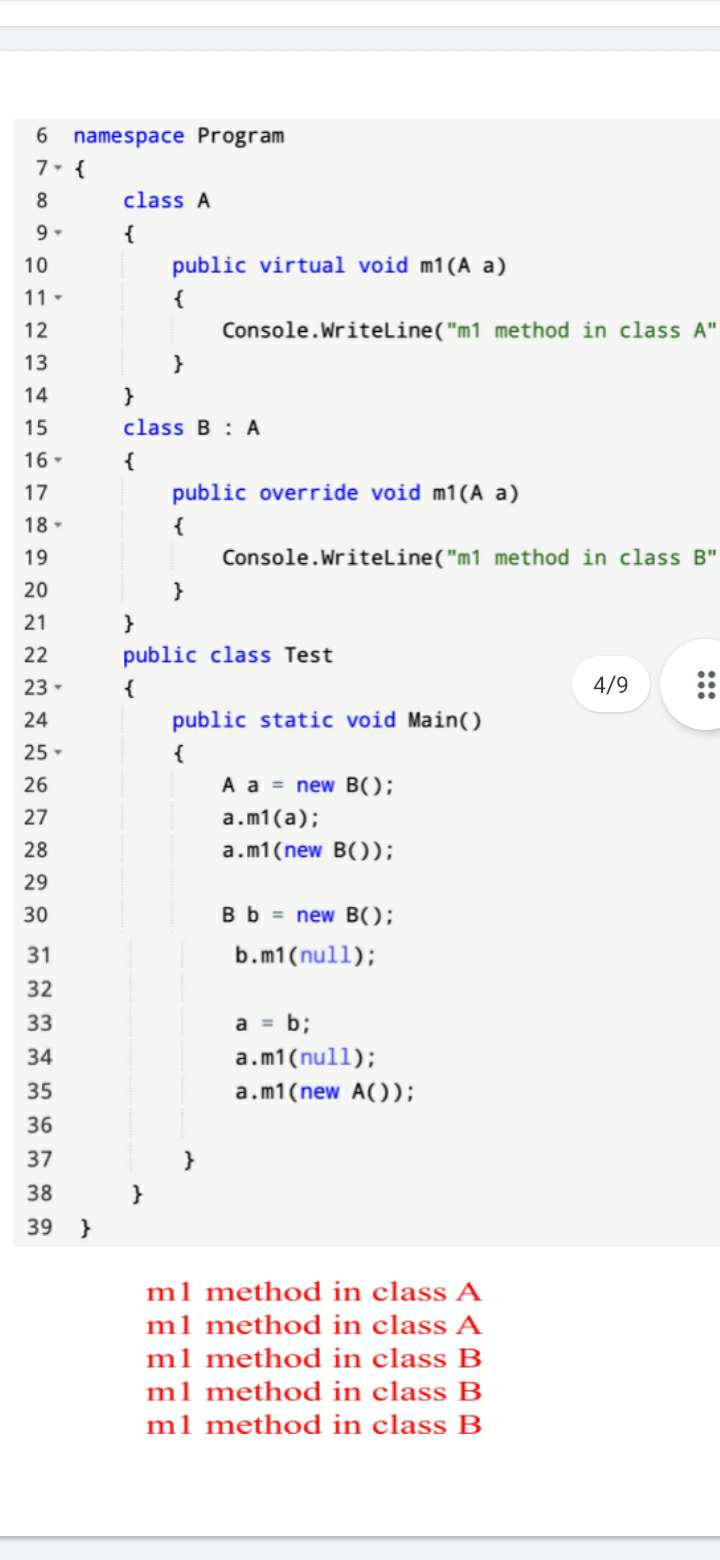
Shouldnt first 2 outputs be m1 method in class B