Entity Framework Core - how to setup this navigation correctly?
Hi all.
Very new to Entity Framework Core. I have three classes right now that I want to be database tables. In my Leaderboard class, I have an ICollection called Scores which is meant to hold a list of all LeaderboardScores that link to that Leaderboard. Though, I am unsure how to get it to actually link so the LeaderboardScores that reference that Leaderboard populate that navigation list?
My classes are below. I combined them into this one file for ease of sharing, they obviously aren't all in one file in Visual Studio: https://gdl.space/qoxeretoha.cs
15 Replies
convention is for the entity PKey to be called
Id
in its own class
so PlayerId
should just be Id
inside the Player
class, but remain as is when referenced as a foreign key (in LeaderboardScore)
other than that, this seems mostly okay. are you getting any errors when generating migrations for this?That makes sense. I'll change it to be like that
also, personal preference, but I really dislike using attributes to configure my models
I much prefer using
IEntityTypeConfiguration
classes 🙂I'm not getting errors, but this line doesn't seem like it is working correctly.
It should be a many-to-one relationship. So a LeaderboardScore can be connected to one Leaderboard. But a Leaderboard can have many LeaderboardScores as part of it.
But EFC doesn't seem to know to populate this line with LeaderboardScore's that use that Leaderboard

Ill look into that! Thankyou
hm...
why are these nullable?
surely a score entry cant exist without both these
Yeah a score entry can't exist without them. They are only nullable because Visual Studio gives me a warning if they aren't

Ah, well instead of marking them as nullable to hide the warning, I suggest you fix your models
in this case by making the fields
required
the nullability modifier actually makes EF think they are nullable
Ahh thankyou, I didn't realize that was a keyword
with these models and these configurations, it seems to work fine
the migration generates as expected and looks fine
Thankyou! I'll try this out
hm
I found an inconsistancy thou
I'd expect a
Player
to have a Scores
property of some sort
they are currently linked to a single leaderboard
can a player not be part of several leaderboards?Yeah, so right now a player is unique to a leaderboard. That is purposeful right now
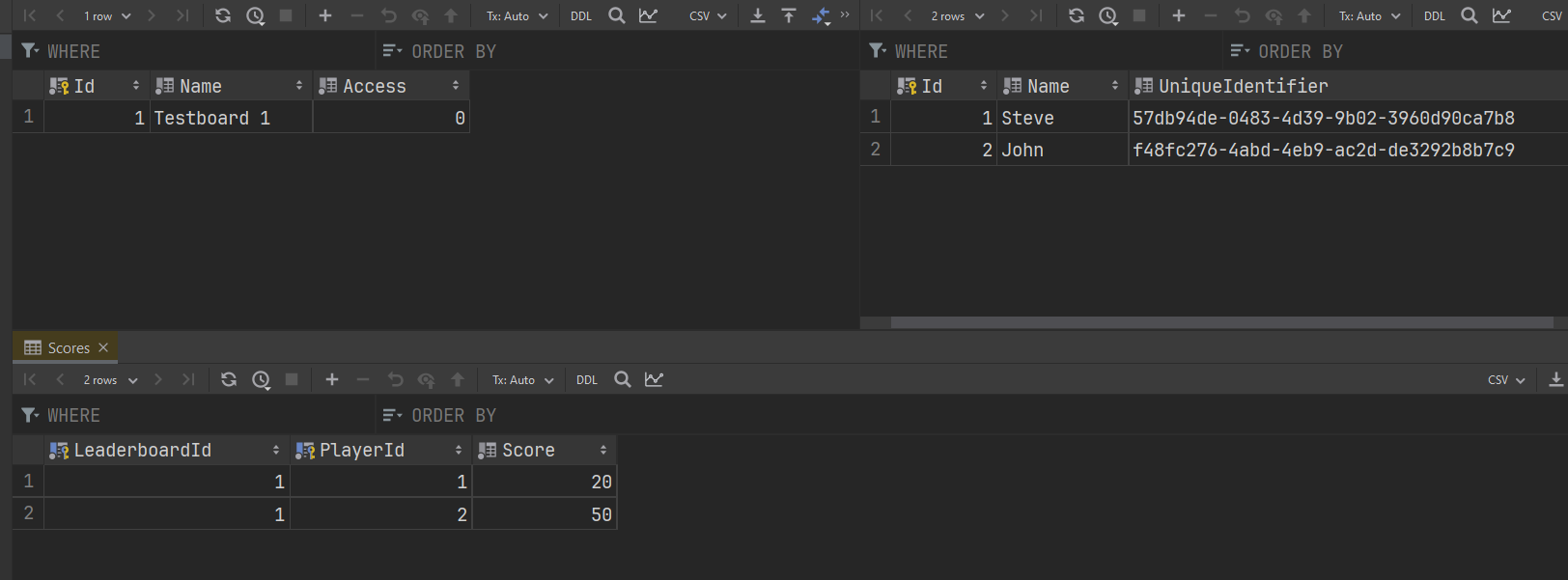
looks good to me 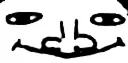
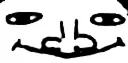