"No Such Column" Error
I'm using TypeScript, DrizzleORM, and SQLite (better-sqlite-3)
How come I get error of
no such column: share
or no such column: todo
?
Thanks in advance for the help 🙂 🙏12 Replies
Hello @christrading! Could you please provide your schema code? This error occurs when you don't have these columns in your schema.
Your typescript schema might not be in sync with your database
You didn't forget to push or migrate?
This is my
drizzle/schema.ts
file
continued
Drizzle Studio shows that I have these tables created
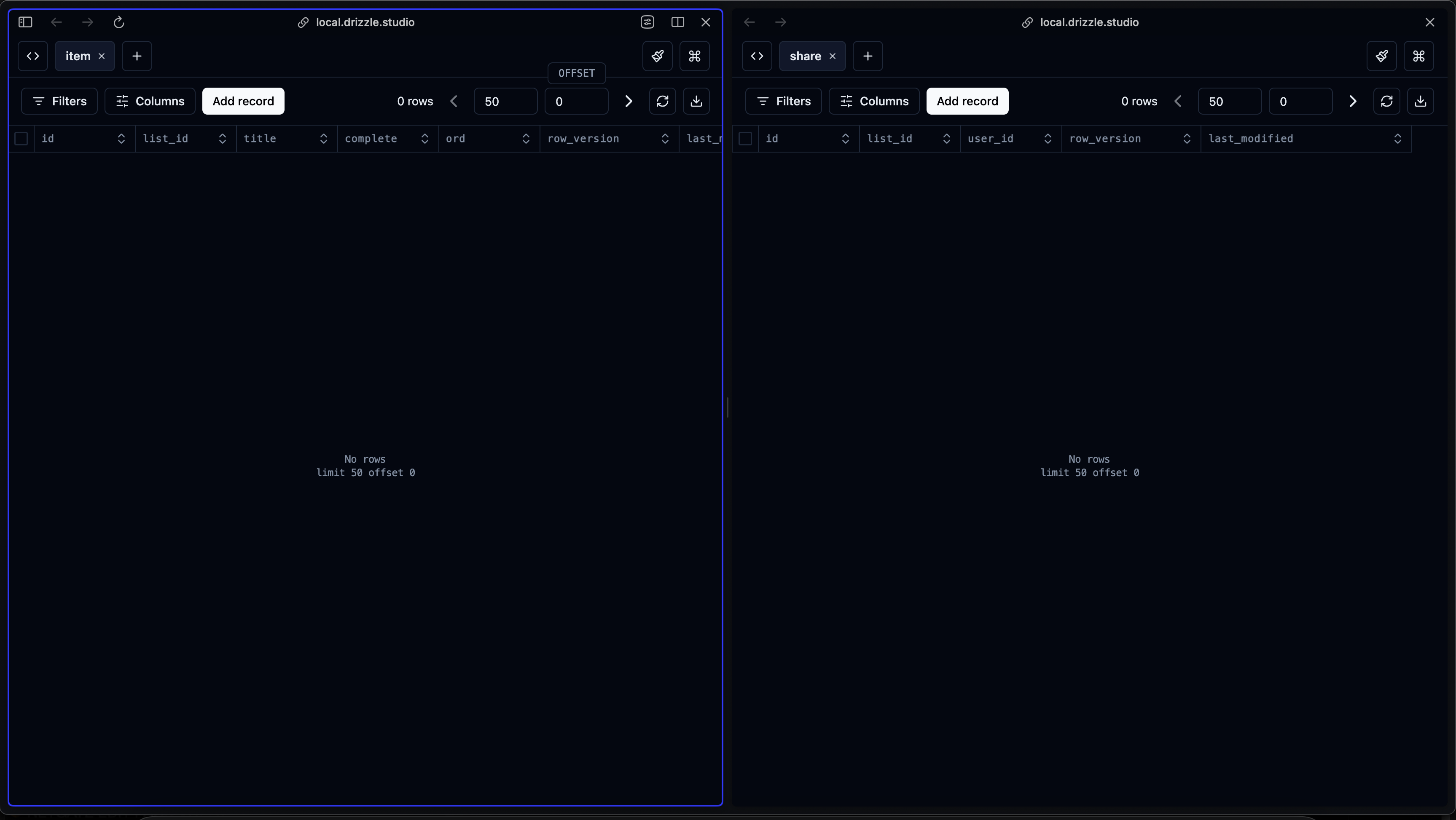
Dirzzle studio is based on your schema in typescript
You could run raw queries to see what's on your tables
Perhaps I am mistaken, but in the select statement, you are specifying the column
share
from the share
table. But you don't have such a column. The same goes for todo
Oh yeah, you're right @solo ,
Are you trying to select a constant or a column??
I am trying to select the columns
id
and rowVersion
from the share table and adding a column to the select result of key type
value share
. And then similarly for the todo table. This is so I can distinguish the returned select results between the share and todo tables
I am adding the column to the return result in the way that Dan Kochetov suggested https://discord.com/channels/1043890932593987624/1043890932593987627/1172440868544860192I think that for SQLite you need to wrap the string in double quotes " or single quotes ', you'll have to test
I don't have a way to test right now but I believe that should work. Or the single quotes
Ah, yes. Wrapping the string with single-quotes on the inside worked
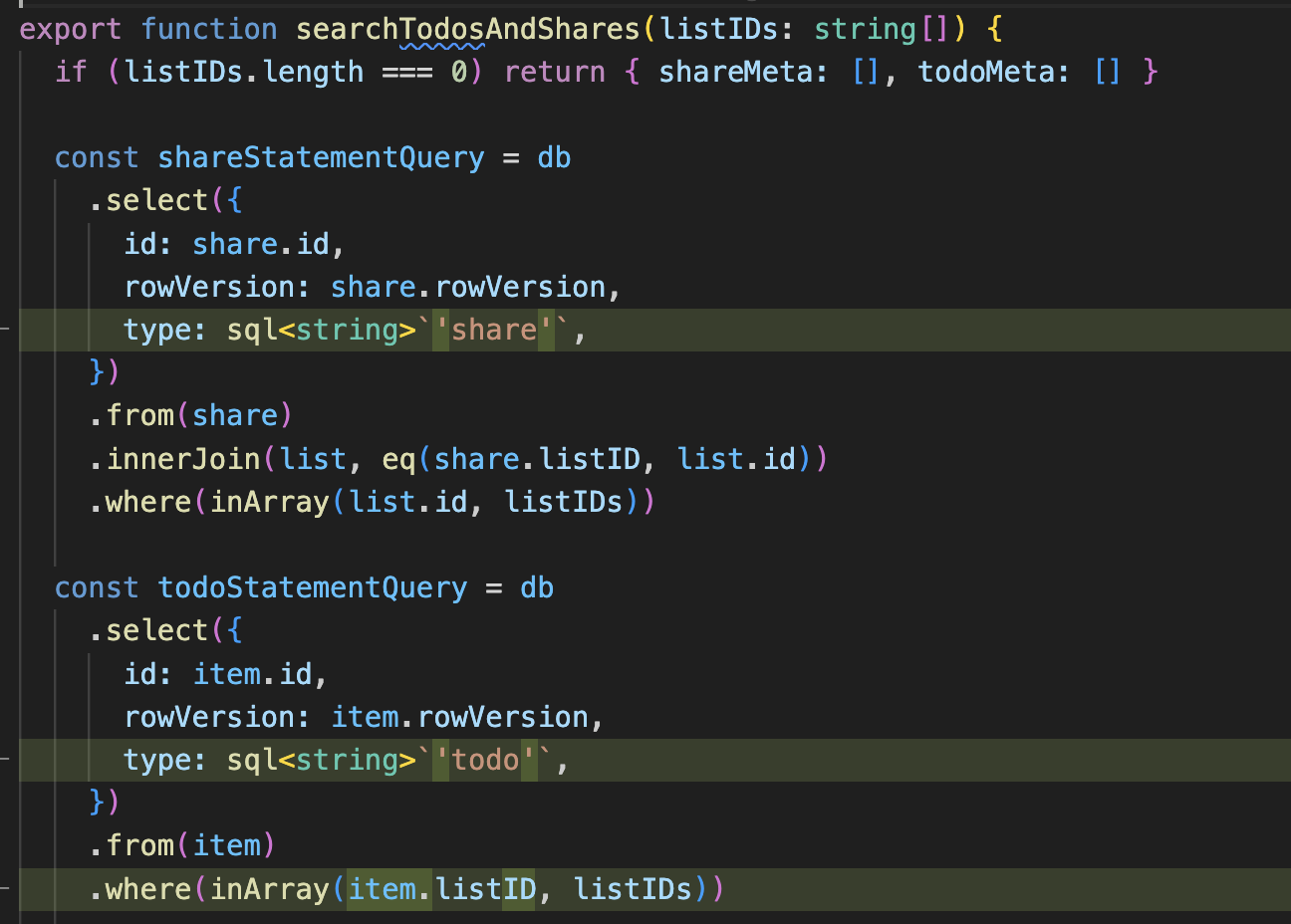
🙂 🙏 Thank you, Solo and Angelez!
This wasn't explicit in the documentation (among a number of other things I've worked through figuring out). Or is there another source of docs that I'm not aware of? Are the docs able to be expanded to explain a bit more comprehensively? Is it open to open-source contributions?
The team is working on updating the docs and guides, yes