How to use cascade with a multi-column foreign key?
When using
references
I can tell a foreign key to cascade on a delete easily. However:
I have a table with a composite primary key:
and a table referencing that table with a composite foreign key:
How do I activate cascade on delete in this scenario?7 Replies
oh, that's a nice feature request
we don't have this option now and should definitely add it
I see, no problem! Haven't had a look at the codebase yet, but do you think this would be a doable first contribution?
This is an easy one because the underlying class already supports it. You just need to accept the parameter in the function. This a nice first contribution
Alright thanks, I'll see what I can do then ^^
oh, I think I literally just ran into the same issue ... I think?
https://discord.com/channels/1043890932593987624/1183062166690082826/1183062166690082826
yes, this functionality is needed asap 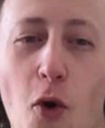
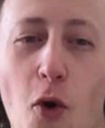
This is being worked on https://github.com/drizzle-team/drizzle-orm/pull/1636
GitHub
feat: Add actions to foreignKey(...) by cegredev · Pull Request #16...
Changed:
Added parameter actions to function foreignKey in foreign-keys.ts for postgres, mysql and mysql-lite which allows for onUpdate and onDelete actions when using multi-column foreign keys.
Ex...