✅ Nested views failing
Hello! so, I've got a small issue.
I have this view called Edit which has the following button:
I am trying to get it so that when this button is clicked, it takes the user back to having the details view opened in this part of the dashboard view (note the page with the submit button is currently opened in there)
this is my current js
here's the end point it hits:
and this is the Details end point:
I have some almost identical code that works fine, but this one seems to just open the view as a whole view instead of nested inside the first
29 Replies
It's been ages since I used jQuery, don't you need to call
preventDefault()
on the actual event? Can you really just use it loose like that?
Also, any reason why you opted for an <input>
instead of a <button>
?in js you call it in the event
I'm not sure what magic does JQuery to allow that 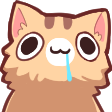
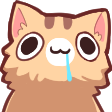
Yeah,
I would assume jQ is similar in that regard
anyway js > jquery 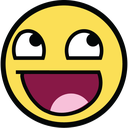
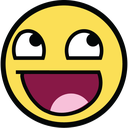
I wasn't sure if I needed to use a input for the forum or if I could change it to a button as from memory a input is what came in the views template.
Well it's not inside of a form in the first place
Or at least your code doesn't seem to have it inside of a form
So
I'm not very familiar with front end at the moment sorry
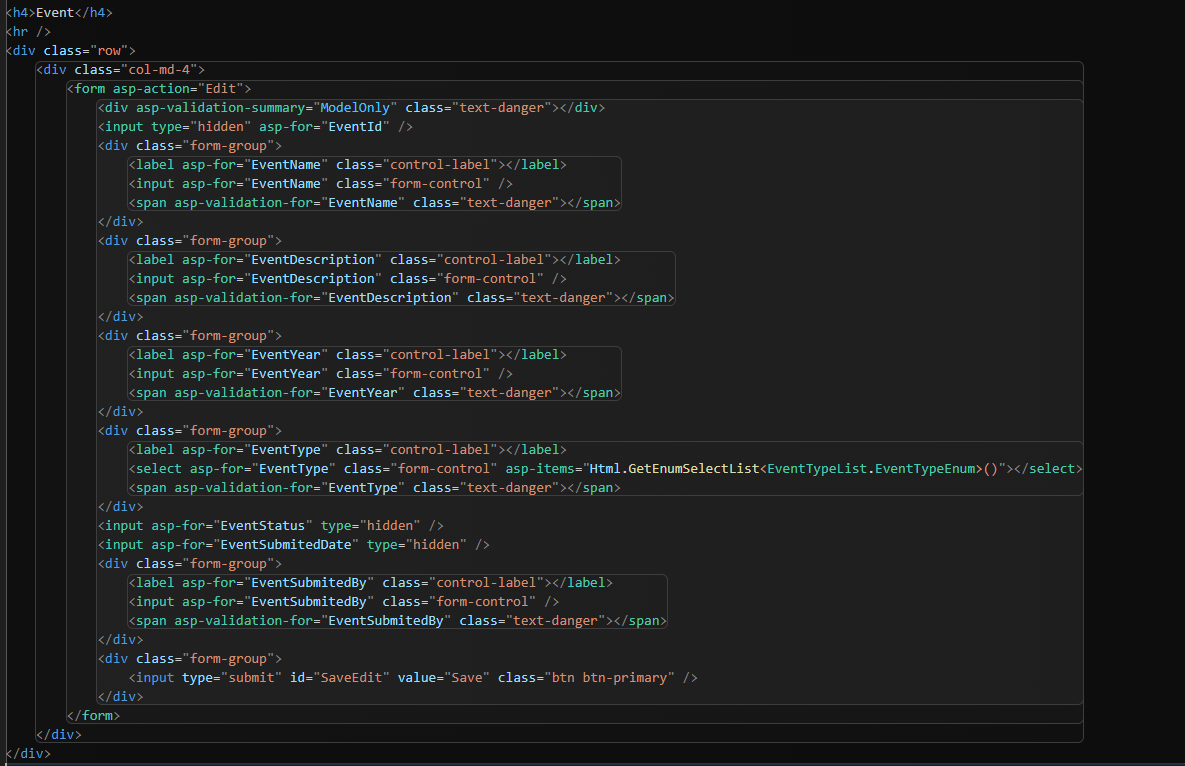
Wait
So you want to send a form
And at the same time you want to do something else?
Or do you want to do that something else instead of sending the form?
Because submitting a form in a normal way reloads the page, or redirects you somewhere. So whatever other JS code runs after that, gets halted
I think it's at the same time. so what my goal is, is the edit view is nested inside another view. when you click cancel edit it returns you back to having the details view nested instead of the edit view (works), when you click save, it saves the edit and then returns you back to the details view being nested inside (does not work)
Just so we're clear, there are no "nested views", you're just getting the raw HTML from a URL and place it onto the page
right okay, I don't really know what words to use so I just came to the conclusion that might be correct, sorry
And so, the data from your form just doesn't get saved, correct?
Or does it, but the HTML substitution doesn't work?
it get's saved, and then opens the details view in full screen where my goal is to make it replace the edit view which was inside another view
Because both cannot work at the same time
If the form is sent, it reloads the page, or specifically it redirects to the URL specified in the form's
action
That stops any and all JS execution, halts everything, cancels it, and just reloads the page
That's where the redirect is coming from, I would assume
Your loose preventDefault()
strikes me as something that would not work
But if it did, it would prevent the form from submitting
So you would get no redirect, as desired, and you would fetch the HTML code and substitute it, but... the form would never be submitted
If you want both, you will have to send the form with JSI think this should show more or less what I mean
ah, okay. makes sense
Yeah, I get it, you're trying to create a pseudo-SPA
so what I need to do is send the data with the JS?
Idk
Haven't used jQuery in ages
Hit up the docs and see
will do. thank you for the help!
check for the function
fetch
If you want to ditch jQ, that is
I'm going to guess I do want to. I have very limited knowledge of js, but I at least have some where I know nearly nothing about jq
If so, then this
becomes this:
https://javascript.info
this website is a nice resource to learn js
I sees ty
I will look into that ty!
I got it working! tysm for the help!!
Nice
you can do /close to mark the thread ✅
oh okay