16 Replies
I am thinking about adding indexes to some fields, but I am not sure. For instance, one of my queries looks like this:
Would it help if I add an index to the debate name? The debates table has something like 200 rows and won't grow past that.
What does need an index is the post relation I think. should I index the createdAt field for faster sorting? or should I index the posts
debate_id
? what about the post's author_id
column?
I have started learning about indexes today and I heard the following rules of thumb:
"Create an index for anything that goes into a WHERE clause"
"foreign keys/pointers to other tables should be indexed"
since my relations are mostly dependent on ids that point to other tables (posts.author_id
, posts.debate_id
, debates.team_id
) my guess is that indexing all of them should be an improvement.for tables with 200 rows there won't be any notable difference
If this is for learning, yes add all the indices you think you need
But in real applications you should add them when you know you need them
This is a real app unfortunately, it blew up on twitter and it got to 5 billion row reads, which forced me to learn about indexes lol
Is there any problem with "over-indexing"?
Uff, yes, add the indexes
The only ptoblem would be storage
There's bigger fish to fry
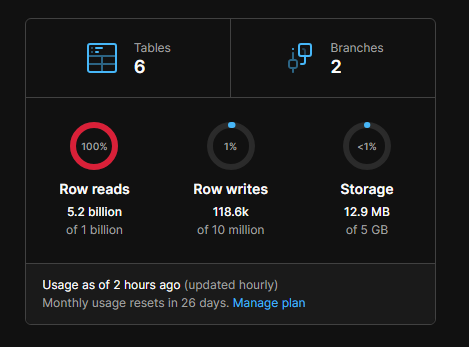
Yes, just add them in all the where, foreign keys and orderbys
Thanks a lot! should I add them both in the source table and the field which references it?
Is this planetscale?
You have explain analyse in mysql
for instance:
Yes
Make sure your queries are not doing a full table scan
Oh they are
that's what planetscale support told me
and they advised me to look into indexes
these are absolutely nightmarish stats
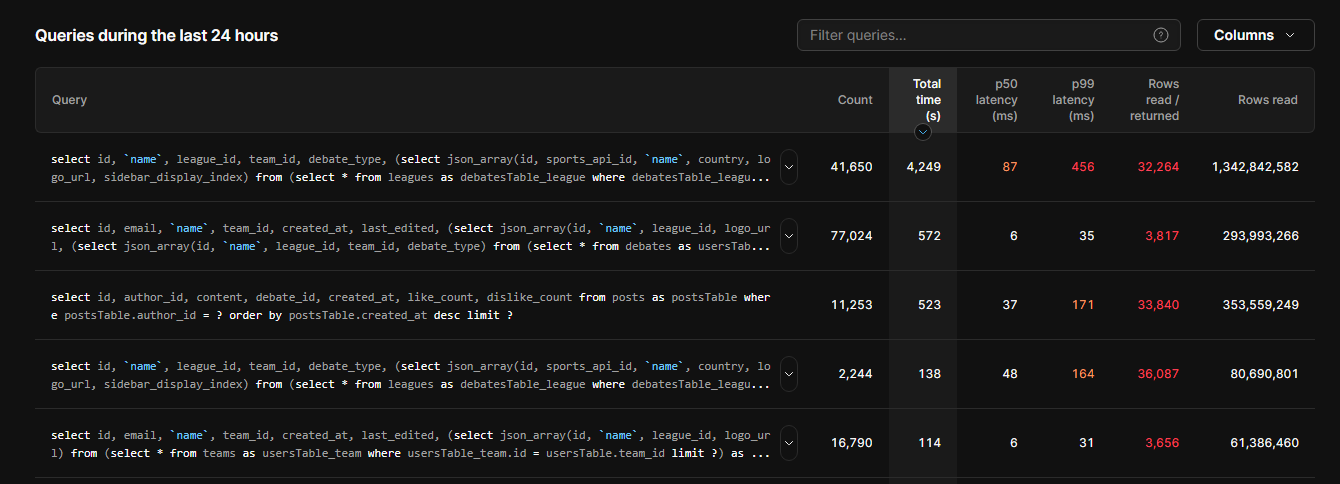
Use drizzle logger, copy the query, go to the console, paste it and put
explain analyze
in front
Start adding indexes and the do that again and compare
Good luckwill try, thanks!
could you please explain this? I am kind of confused
for instance, if I'm pointing to a primary key, should the field that points to it be indexed?
I don't think that index is necessary
It's already covered by the primary key
Unless you are ordering by it descending
Ok thanks!