ASP.NET MVC
How do I check that my input (html) (from the view) matches the data in the database? (For example, like matching Username and Password).
While also using DataAnnotations to validate that the input = database stuff?
Also would like someone to do some pair coding to help me out if they have time.
23 Replies
If by "html input" you mean a form, then you can bind to the form data
I was using a form.
So, I can "bind" that parameter in the controller to my "<input>" using a tag-helper?
No need for tag helpers
Input named
name
goes into parameter named name
Updated. Still don't know if it works.
Well, try it?
If that doesn't work, you might need
[FromForm]
attribute on the parameter
Also, where are you getting the questions
from?
"questions" is coming from the razor model (@model List<Question>) on the top of my razor page.
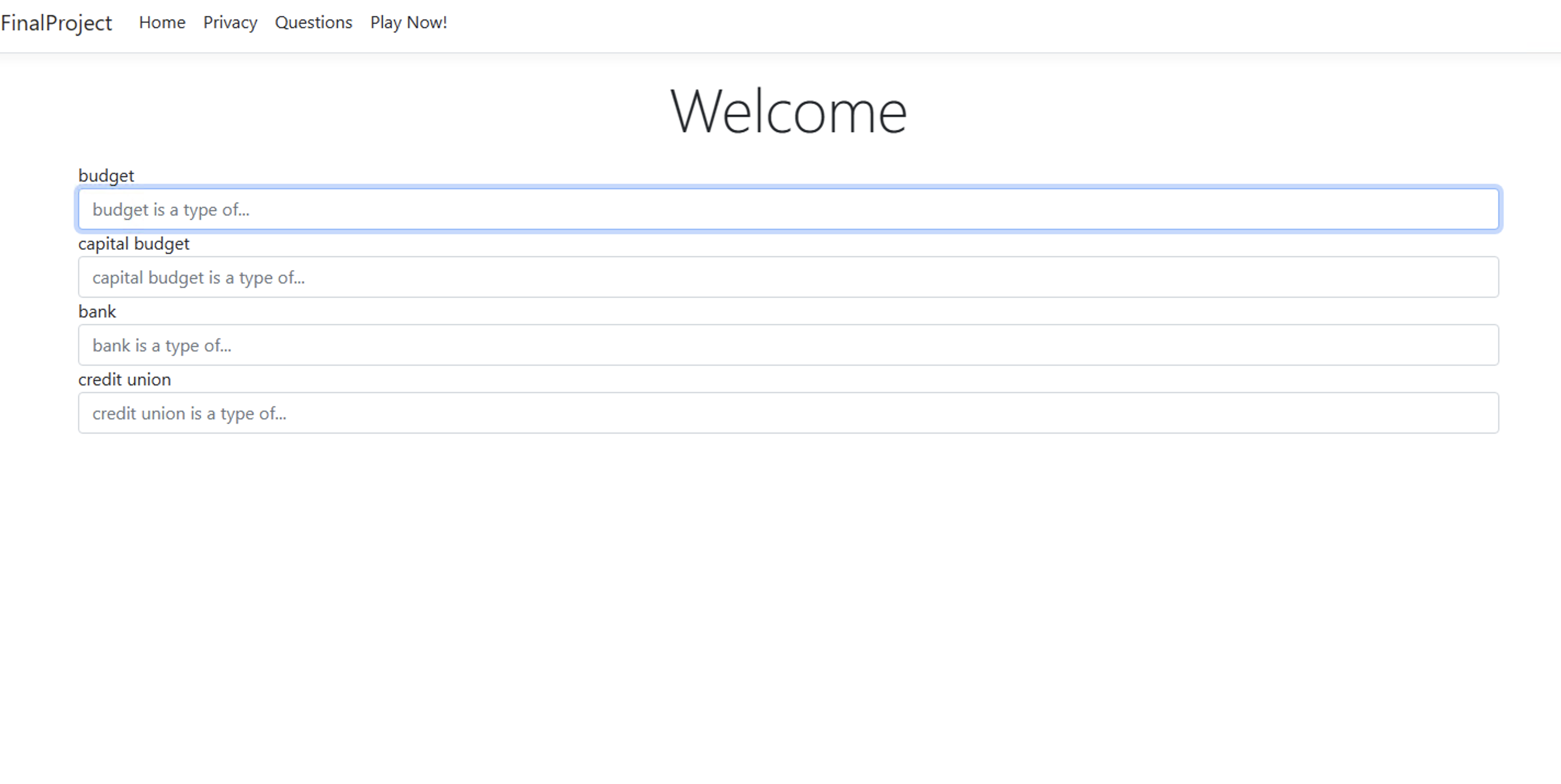
Above. Is the example of my code running.
Ah, so you want a list of inputs, essentially, gotcha
@ZZZZZZZZZZZZZZZZZZZZZZZZZ What do you think about my using of the asp tag helpers like asp-controller , asp-action, and asp-route in my input?
You'll have to look up how to deal with dynamic forms, then
It's a game where you have to match the definitions basically
Inputs don't have any URL-like attributes, so
asp-controller
, asp-action
, etc. are useless on them
The form defines where the data is sent
So the form needs that
This is what I changed it to
So the "name" tag helps connect the view to the controller?
Esp. the parameter
Yes
Input with [name] -> parameter with [name]
But in your case, you have a dynamic list of inputs
Each should have an individual name, too, since they refer to different questions
Ah ssibal
So, again, you should look up how to deal with dynamic forms
Ok I will
One more thing, can I use the "Enter" button to submit my <input> or do I need to connect a button to it?
Might need Javascript for that
Okay, I don't know Javascript haha so maybe just use a button
Going to bump my thread to see if anyone can help me.
I am still having issues with my program.
This is my controller
This is my view
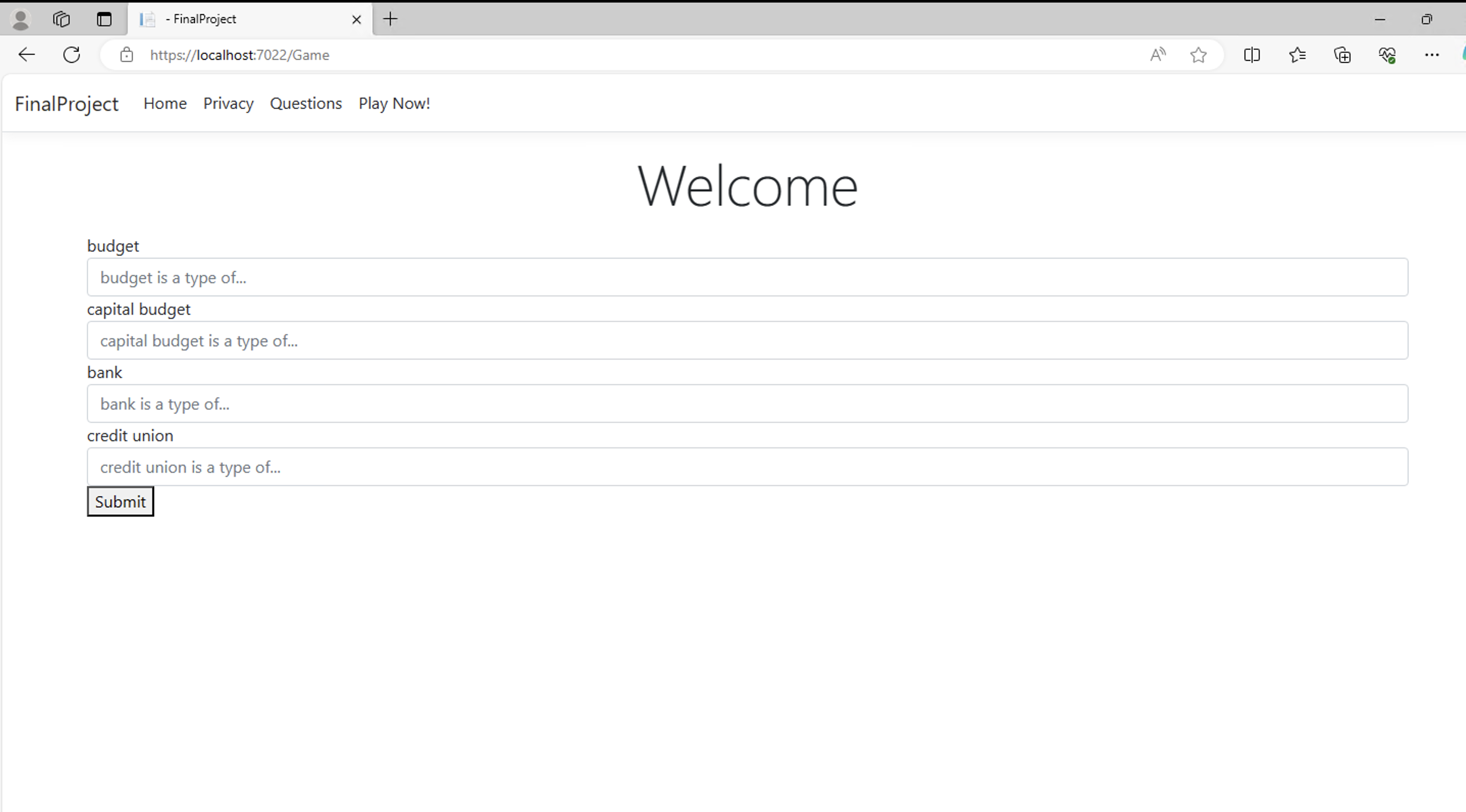
This is my website
I don't understand how the form is submitting
I am not able to transfer my data to the controller
If I switch my asp-action="Save" in my view then it will crash on submitting
Saying it can't find a save view
One thing is that my ModelState.IsValid is returning false, and I don't know why this is.
I want it to send my viewmodel to my post
Ok well I've made progress
But my current issue is now my data is not being passed correctly to my controller
I think it is how i coded my view
But this is very difficult for me
I think my ViewModel is formatted wrong for what I want for my game to work
aswell