Supabase/postgres get query is stuck on prod app
Hi,
I'm coming across an issue with my discord bot, I currently have a query that checks if an user exists in the database. But for some reason, after a while (I believe) this query gets stuck and my bot becomes unresponsive because of it.
I currently have this app hosted on railway and I can see from the logs is that it stops after it queries the DB.
I've checked the supabase logs and it shows that the connection is received and authorized.
Honestly, i'm not sure if this is a Supabase issue or a drizzle issue. Maybe someone knows what could be happening?
--
configs:

12 Replies
looks like it could have been that I have the postgres property
max
set to 1
😬
After a while it still stays trying to query 😔
I added redis because i was making lots of calls to the DB to offload, but it still gets stuckI have a discord bot that makes sql queries whenever an event happens; for example, when people talk in a channel, the bot checks if the channel is of certain criteria set on the DB - this means there's a call everytime someone talks in a channel. I believe this caused issues, because when channels become very active this requires many DB calls. To offload DB queries, I added redis and it helped a bit but it seems that my app after a certain amount of time gets stuck making calls to the DB.
It looks like I get errors back but they just come back as an empty {}. I am not sure how to debug this. I am not sure if this is a drizzleORM issue or a supabase issue
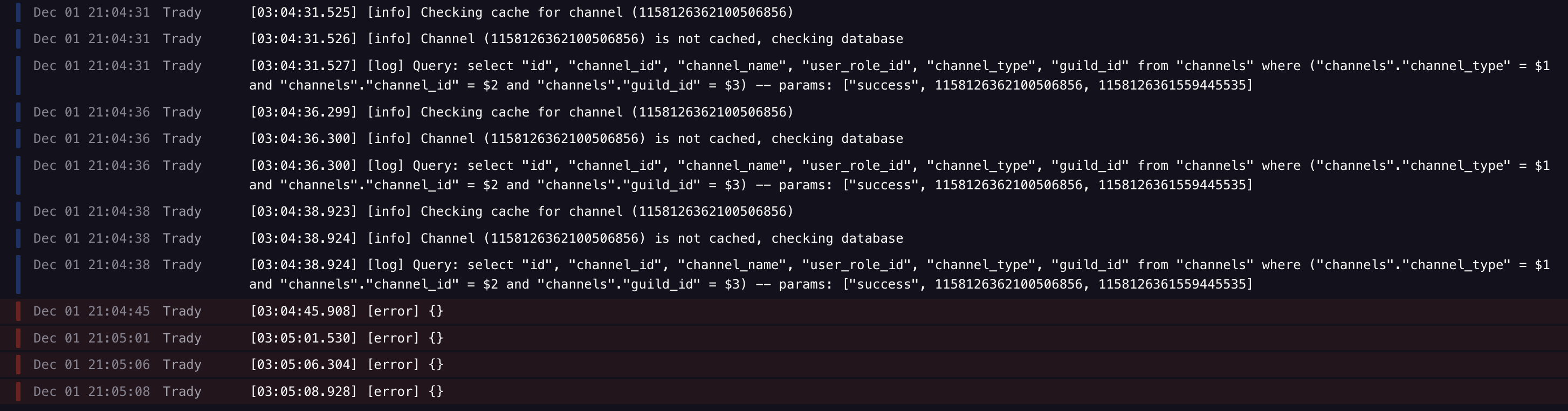
so you are using postgres.js
but I can't see anything from with drizzle setup so far
it may be supabase issue
I guess you can try to run this in docker Postgres
or neon
as long as all of that is postgres compatibale - you can just change connection url
if you will see that issue is gone - it's supabase issue
if not - I can check more into drizzle
@Andrew Sherman it's very hard to debug because it does not happen in dev. I do have a supabase dev environment on my local at this time and it works fine.
In production, the queries work but after some time it just stops working, so it might be a supabase issue!
yeah, seems like it
Yup, thank you for taking a look!! I'll report back once i find a fix
Hey @Andrew Sherman sorry to bother you once again.
So it looks like it could be a client issue, I was able to gather some logs:
Have y'all ever seen this
unsupported frontend protocol 0.0: server supports 3.0 to 3.0
issue with postgres?
I also changed my database's password since it looks like auth fails sometimesnever saw this one
seems like it's postgre.js driver issue
have you seen any on their GH issues page?
nothing
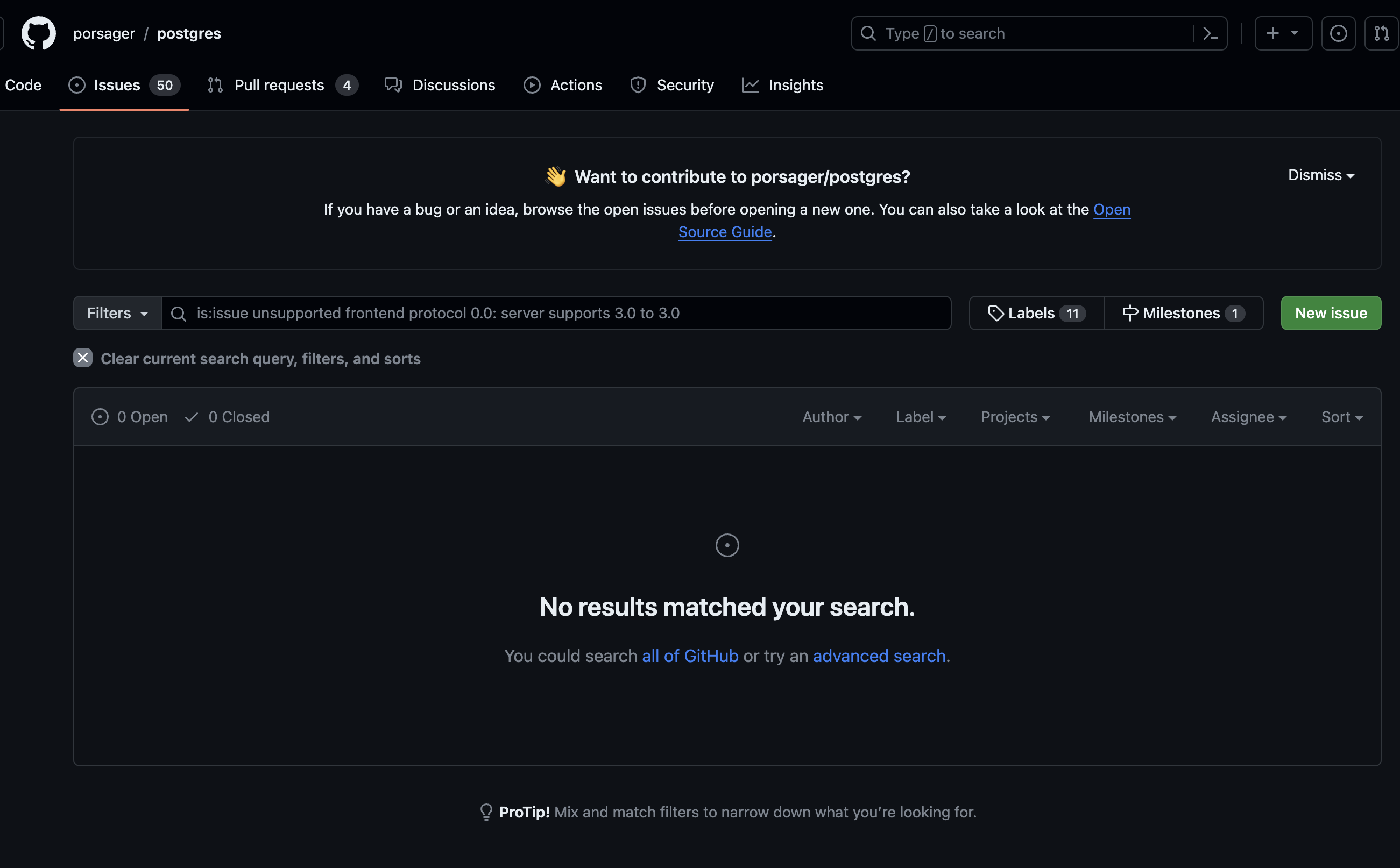
Yeah weird, found some explanation https://pganalyze.com/docs/log-insights/connections/C31. Could be my host as well
@Boxer did you try the new Supavisa connection Pooler? Might resolve things
Which one?
For your Supabase connection string