table count status
I want to create a table that counts the number of lines by status of the 'Demande' database.
so I do this:
i you have any idees it will be nice 🙂 Thanks you !
28 Replies
For information, my result is this :
but i have 586 lines in my database "Demande" so it's make no sense
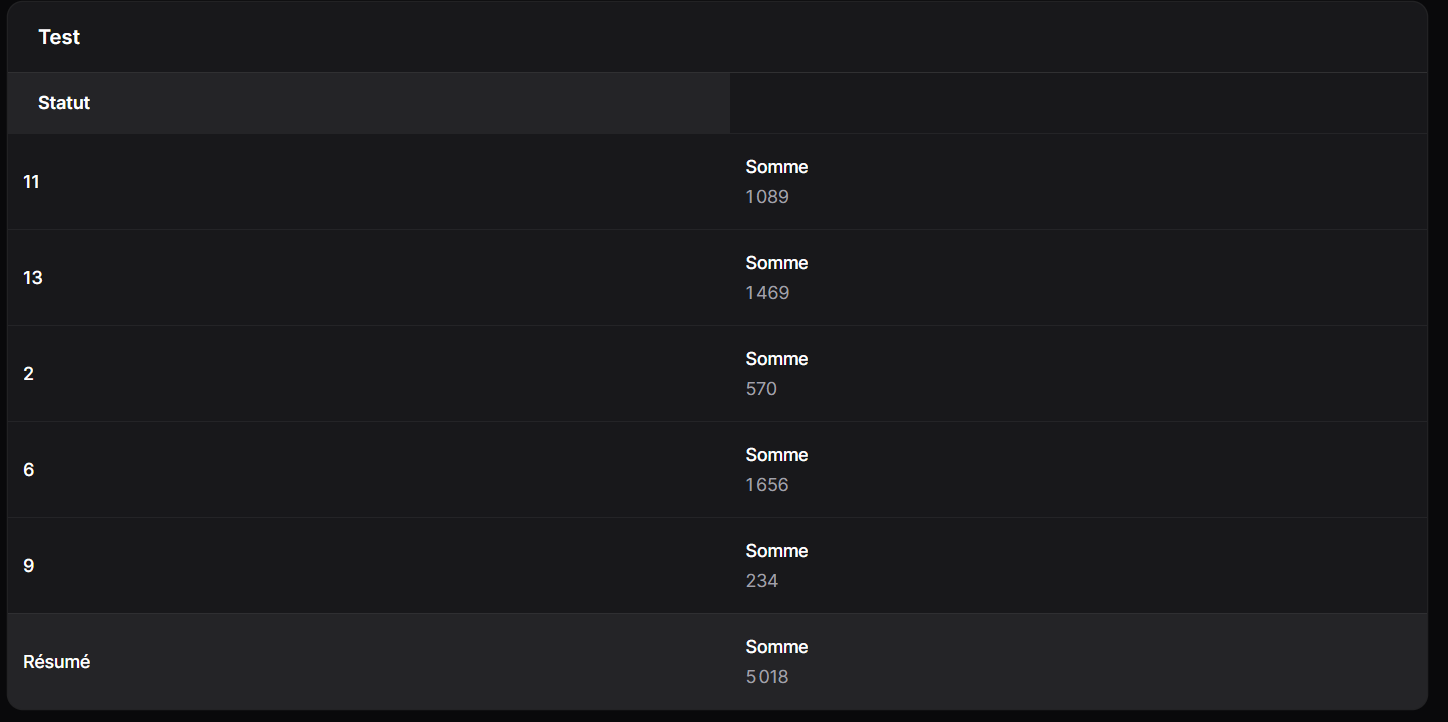
Um, it's the sum of all the values? I think you want to count them, right?
So you can try
->summarize(Count::make('statut')),
instead
Or you can do it using SQL (select count(1) ... group by statut
) but that's a bit more involvedthanks you a lot it was this :
and you know how to customize the "Compteur" on the screen ?
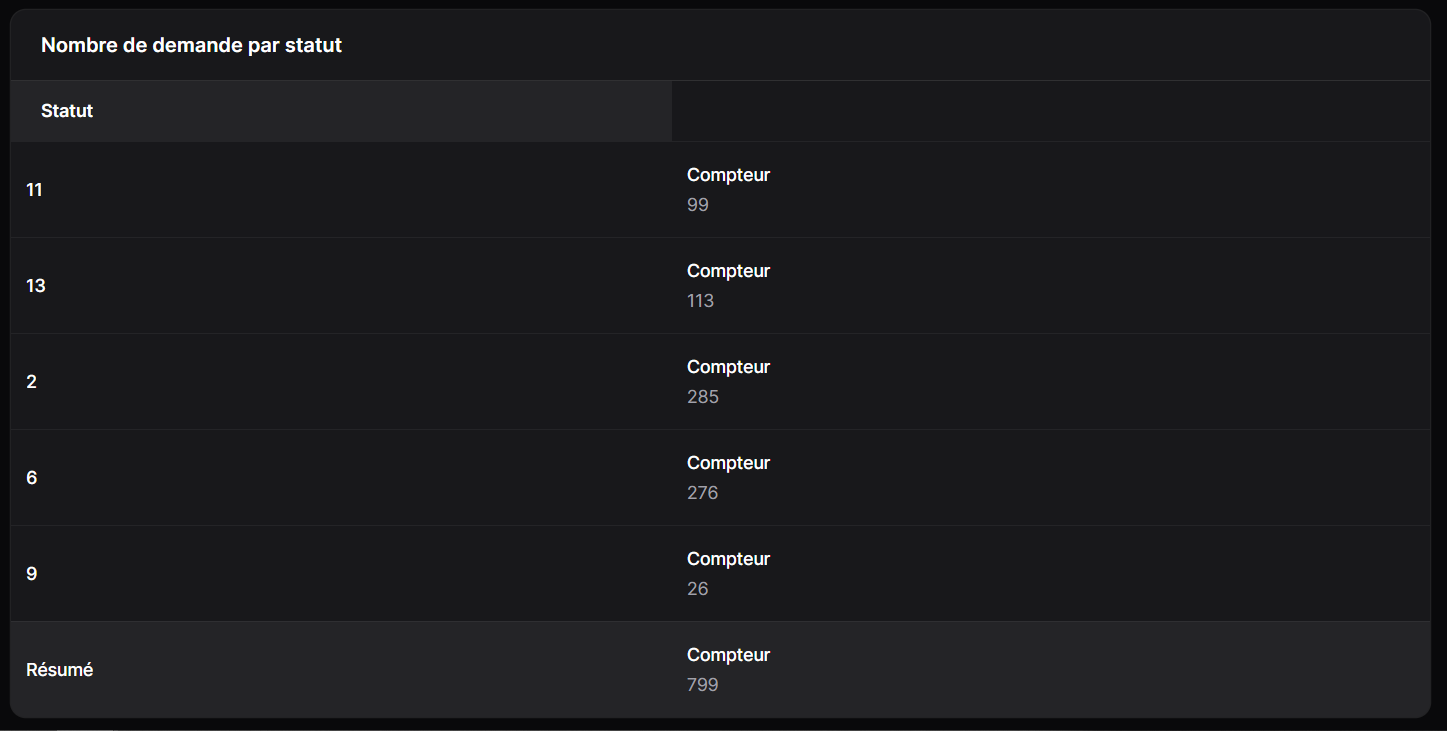
I think you can do
You'r a monster thanks !!
I have a last question :
I want to remove the status number (like 11 = Create, 13 = End, ...) so i create my Enum for status.
so in my page DemandeRessource.php, if i do this :
its works and my status label replace my status number.
But in my page Widget\NumberDemandes.php, i do this and my dd() is not detected :
if i remove dd() and i put just that didn"t works
You know how to do it ?
what is the value of
$state
?
Also have a look at this page, it might help simplify the code https://filamentphp.com/docs/3.x/support/enumsAnd what does the
getStatut()
method do?getStatut() take status number (1 for example) and return the label ('Create' for example)
$state value's is the status number
Ok, if you follow the instructions in that page, you won't have to do any of this 🙂
But i can't use number on my bdd if i follow the instructions of the link ?
You need to add the
HasLabel
interface add a getLabel
(like the example)
What do you mean?
it doesn't have to be strings, it can be numbers
can you share your enum?And don't foget this
When using an enum with an attribute on your Eloquent model, please ensure that it is cast correctly.https://laravel.com/docs/10.x/eloquent-mutators#enum-casting
getStatut -> take int and return string
getStatutId -> take string and return int
Yeah this can be 'simplified'. Also, it's not a great idea to get an enum from a string since strings can be translated
BTW your enum is wrong heh
Gimme a sec
I would suggest you read a bit about enums. You don't need to explicitly give them values unless you want/need to.
ok I put that in my code but then how do I display the status correctly? because according to the link there is the ->options() method, but it does not apply to the TextInput
Why do you want options on a TextInput? Use a Select ...?
I can't because Select don't know
"Method Filament\Forms\Components\Select::summarize does not exist."
I don't understand what you're trying to do. You want to be able to change the status from the summary table?
No it's the same problem :
I want to change the numbers to the statuses that correspond to them
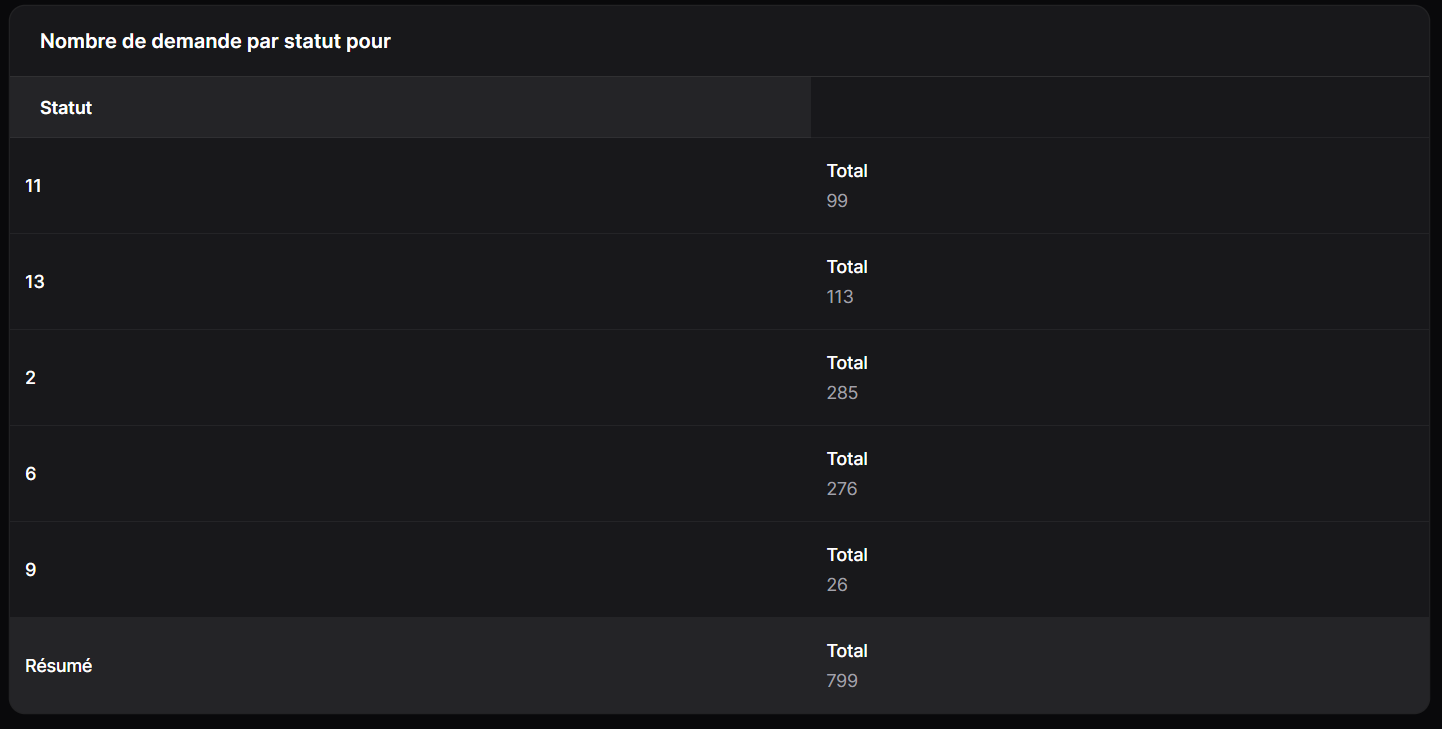
So 13 = finished, ....
Ok, this is a table. Nothing to do with TextInput. You need
TextColumn
. Follow the instructions here https://filamentphp.com/docs/3.x/support/enums and it should work as expectedI follow the instructions but it didn't work, I have the same result and the same problem :
my StatutEnum.php :
my Filament\Widgets\NombreDeDemande.php :
I think it's because nowhere do I say that the status of the NumberRequests file is linked to the status of the StatusEnum file
have you any idee ?
Do you cave a cast in your
DemandeResource
model?
Man thank you a lot for all 🙏
Have a good day !
Haha did it work or are you just not interested any more? 😂
It's work !
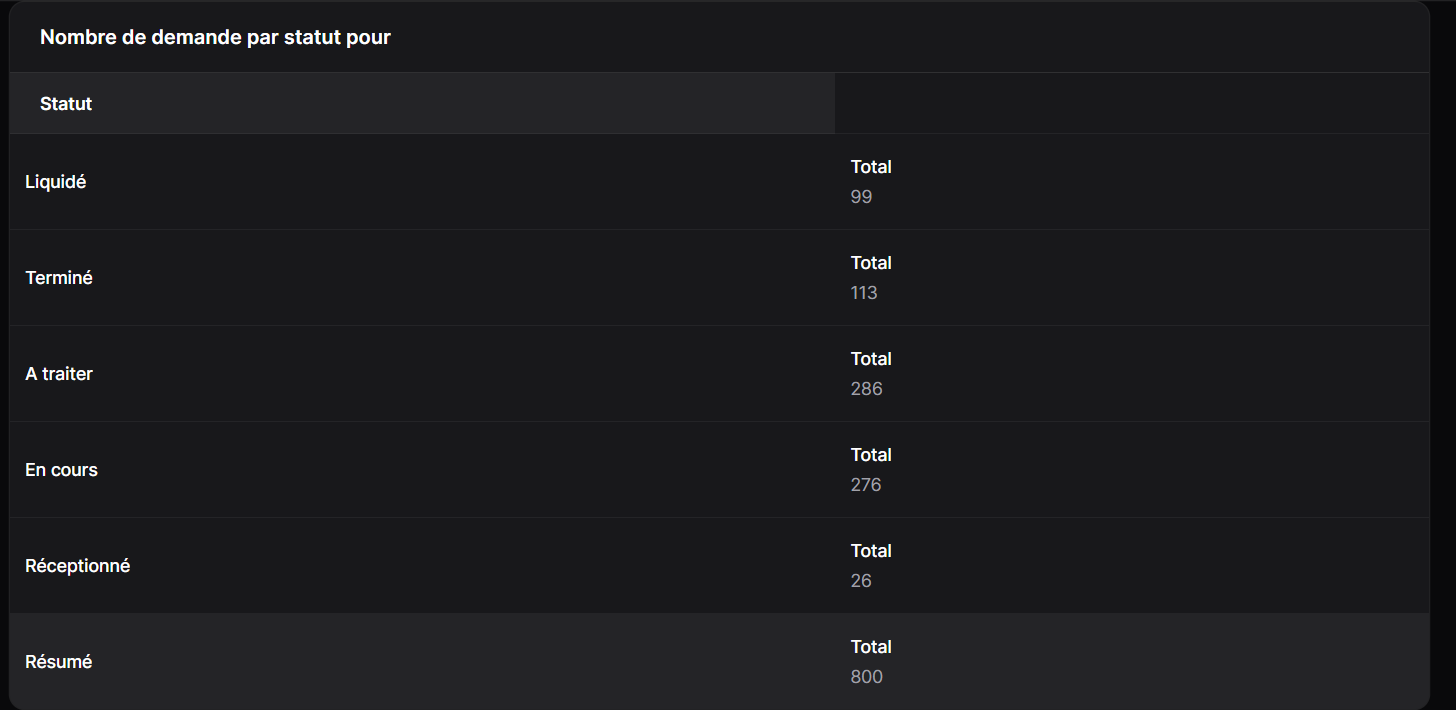