How can I replace enum of Badge column?
I know that the documentation saus that it is removed, but in v2 I used enum with the Settings page. How do I approach this now?
22 Replies
Something like
in your
Status
enum you do
You can do the same with the label
Make sure you have cast your field in your model.
https://filamentphp.com/docs/3.x/support/enumsThe upgrade guide says:
BadgeColumn::enum() removed You can use a formatStateUsing() function to transform text.Also the above mentioned way should work
Hello, Ive tried this but I have gotten it to work yet. Im using SettingsPage as well, and I think that complicates things a little bit. How do I specify the enum() from v2 to the enum class?
Yeah, I mentioned that I saw the comment that it was removed, but now im not sure how I can implement it in the class
I would suggest you read a bit about enums though
It can be much nicer if you change it to something like
Also, make sure you cast it in your model
Hello! With this, I only get the id of the status
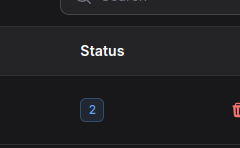
Ah, I see now, I change it to
The message literally said: „it was removed, use this“ 🤔
@Matthew Please actually read the documentation here https://filamentphp.com/docs/3.x/support/enums
It also handles showing different colors based on the enum value. If you follow the directions, your end result will be much simpler
And if you want the text to be translated, translate it in the
getLabel()
methodThanks, I will look further into it. Ive never worked with enums before
Hello again. I followed this approach. However, this gives me the wrong results. Instead of getting pending, I get approved.
Im not sure I understand what Im supposed to do. I read the docs, but I dont really get it still
If you have an enum and a cast for that enum you can entirely remove formatStateUsing
Have you made any changes to your enum and form? How do they look like now? (the code)
I havent made any changes, because they all led to errors, or wrong output
I will share again
for some reason it seems unnecessarily complicated 😅
Does
status_id
have a cast to enum?it does not
Why not?
That’s exactly what I told you in the last message
Dont I need to do something like this?
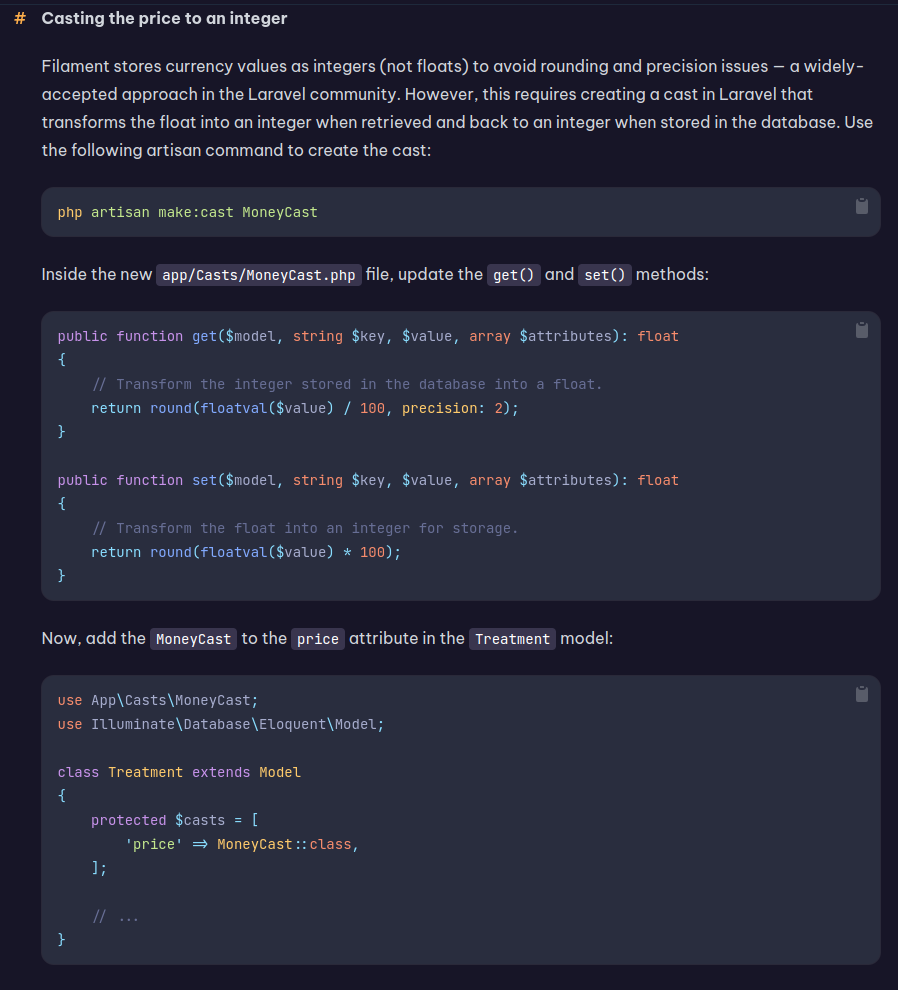
No. There is a cast for Enums. Check the Laravel docs
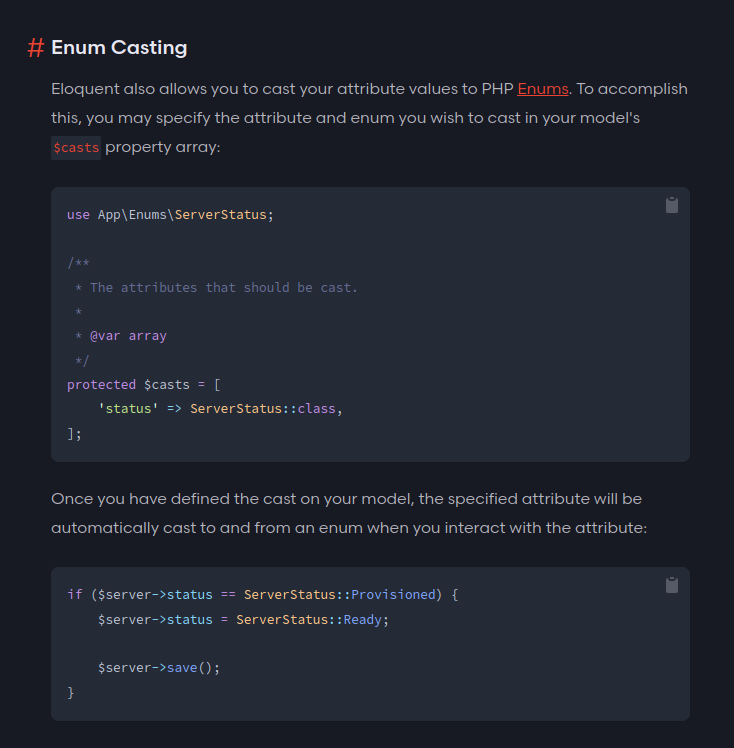
Yes
Im getting error: "1" is not a valid backing value for enum App\Enums\TimeRegistrationBadgeEnum
You have done literally none of the things suggested here
Still using
BadgeColumn
Not followed either the instructions in the documentation or suggestions posted here (like casting to enum
)
You must read about enums - the error you're getting ("1" is not a valid backing value for enum App\Enums\TimeRegistrationBadgeEnum
) is probably because your value from the DB doesn't match the enum values.
Here is your final solution
If you do the rest of the steps as described, this will work.
Good luck