db.update fails with undefined 'relation.referencedTable'
Running into a strange
undefined
error when running db.update
on the lone table in my SQLite database.
The offending lines are db.update(todos).set...
where db
is the connection to the database through Drizzle, and todos
is a table defined by:
I can't find anyone who's run into this same issue, hoping someone has some insight on whether I've screwed up the syntax here.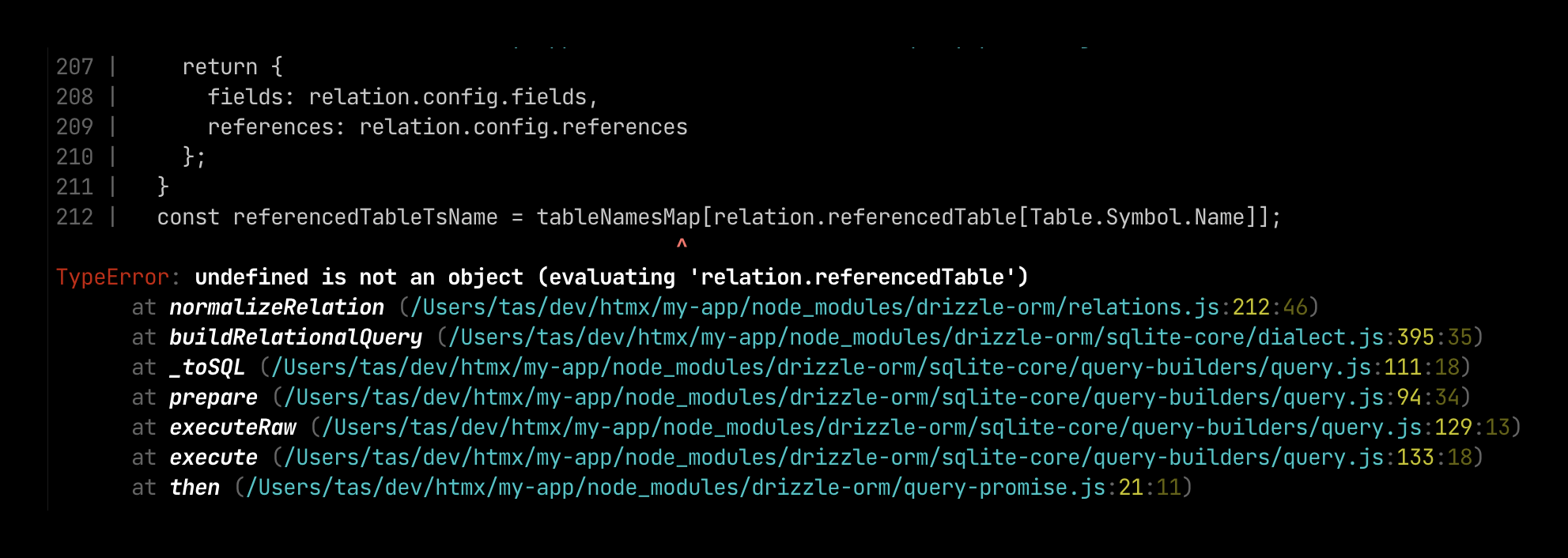
4 Replies
This is a common error when you don't define or pass all of the relations properly to the drizzle function
The relations have to be defined on both sides
That’s the thing - there are no relations in my db. It’s only the todos table.
Do I need to pass some empty list of relations?
In that case, this is the source of your error:
I think that you meant to write a where clause there. The
with
is to bring in your related tables
I'm surprised you're not seeing a type error in that lineOh. I see! Thank you so much.
I’ll go give that a try