can someone help me with my abstract class
I've created my abstract classes and no we can't make instances of them, but i keep getting errors even after following youtube videos to the t.
here's my first file using abstract classes
25 Replies
in my main program, i know we can't do, for example, " RailroadCar railroadcar = new RailroadCar()," but in the exmple on youtube, i could do something like "RailroadCar railroadcar = new TankCar();" but that doesn't work either. I am still new and trying to understand, but I am so stuck
Does
TankCar
inherit from RailroadCar
?yes it does
one moment
It has to work, then
Angius
REPL Result: Success
Compile: 566.461ms | Execution: 36.685ms | React with ❌ to remove this embed.
here's a tiny bit og tankcar
of
i don't see what I am doing wrong then
Your constructor is protected, that might be why you're getting errors
bc i keep getting the same error :///
But it's hard to tell without seeing the actual error
ill take a picture of it one momebt plz
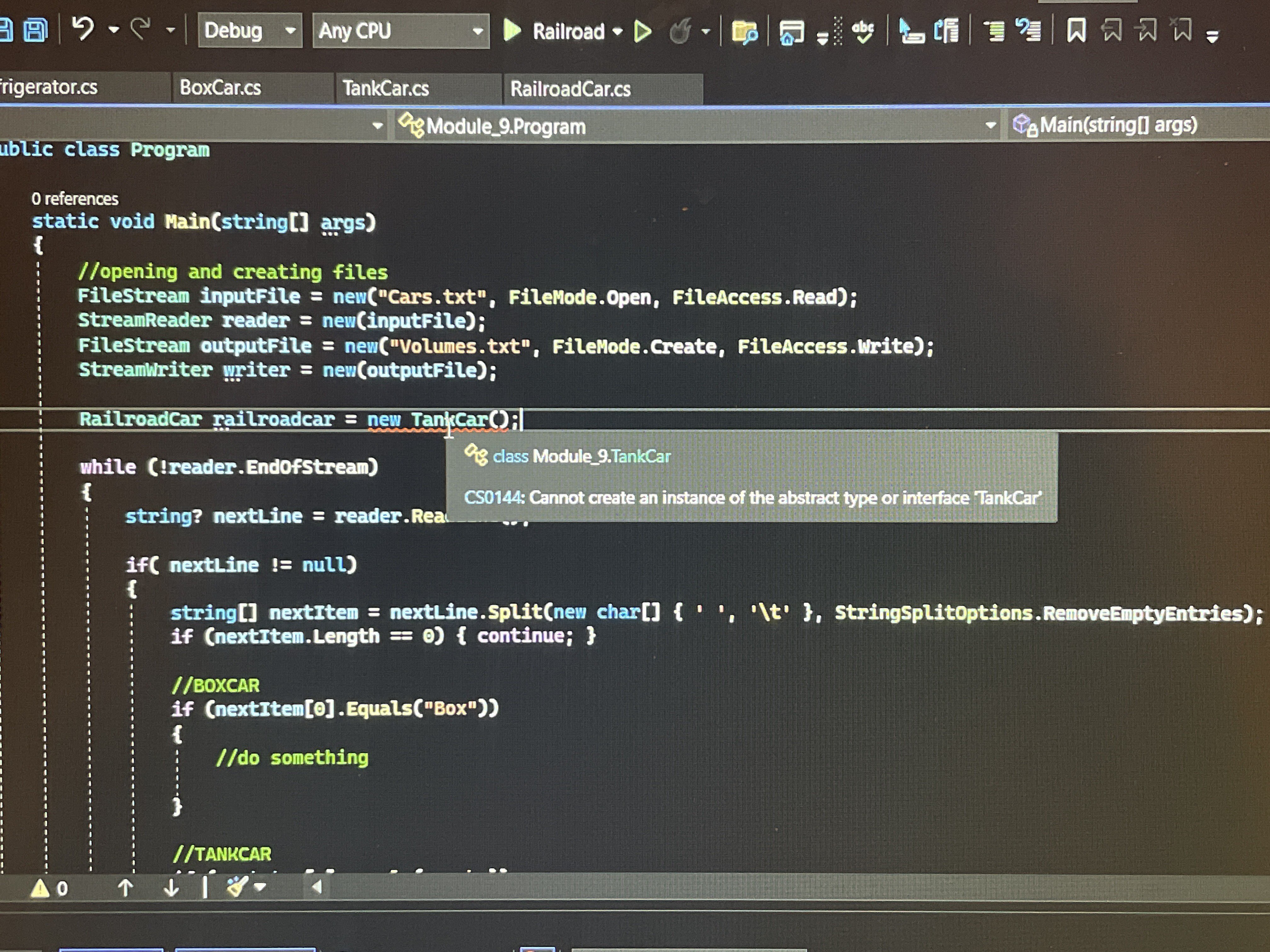
should they all be public instead of protected ?
Ah
Well
Your
TankCar
itself is abstract
And, as you said, can't instantiate abstract classesone moment lemme try fixing that
okay i made them now a public class derived from the abstract classes but it’s still not working
Is the derived class still abstract?
yes
that's how my prof is doing it too
It's not
It cannot be
Because instantiating an abstract class is not possible
The base class can be abstract
But classes that inherit from it can be not-abstract
oh no wait sorry
the base class is abstract
the derived classes are not
they're all public
And in your example the derived class is still abstract
Hence the error
oh wait let me show up my updated version
here's the base abstract class
heres the TankCar class
Now it should work
why is there still a squiggle ugh
lemme refrsh plz hold
What does the squiggle say?
okay i just needed to save and refresh
VS does that soemetines, it's annoying
tysm !!
u were a big help
Anytime 
