67 Replies
It's a bunch of LINQ code
.Distinct()
gets only unique elements
.Where()
filters the collection by the predicate
.Count()
gets the amount of items in the collection
.First()
gets the first element
condition ? iftrue : iffalse
is a ternary expressionremoves duplicates
x => stuff
is a lambdai don't get this
meaning?
but idk what it means in this context
Angius
REPL Result: Success
Result: WhereArrayIterator<int>
Compile: 574.081ms | Execution: 98.215ms | React with ❌ to remove this embed.
filters with a condition
i thought => was to return a value
Angius
REPL Result: Success
Console Output
Compile: 782.019ms | Execution: 141.873ms | React with ❌ to remove this embed.
Kinda-sorta
The lambda
number => number % 2 == 0
will return a boolean
If that boolean is false, the element gets filtered out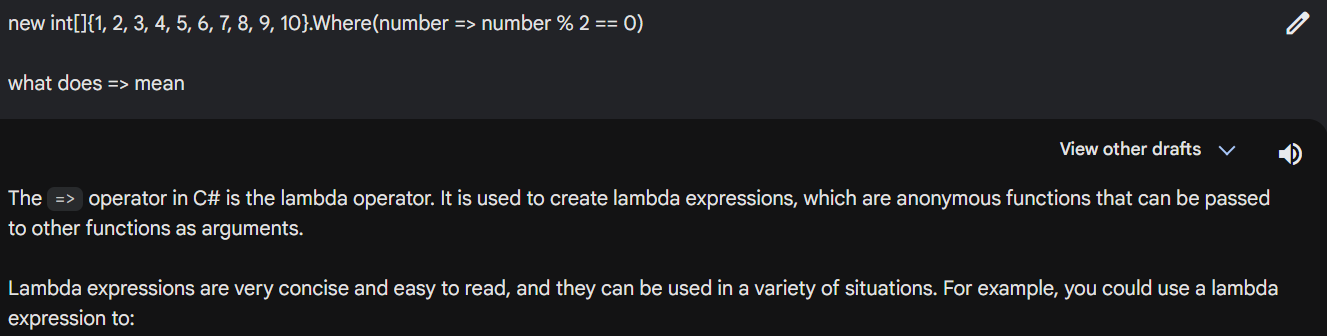
is
number
a made up var?
number
=> number...
that one
is basically a shorter, anonymous version of
It's a parameter
is it only for lists?
like
No, you can use a lambda whenever, wherever
the
but in this context
is it for lists
Angius
REPL Result: Success
Result: int
Compile: 596.015ms | Execution: 63.795ms | React with ❌ to remove this embed.
=> is for returning
If you're talking about LINQ, the
.Where()
part, then it's for any IEnumerable
Including lists
It just happens to take a Func<T, bool>
as a parameterso
public static int? Closest(int[] arr)
{
var min = arr.Distinct().Where(x => Math.Abs(x) == arr.Min(Math.Abs));
return min.Count() == 1 ? min.First() : (int?) null;
}
that's saying
Distinct removes all dupes
math.abs(x) == arr.Min(Math.Abs))
the absolute value of x
is equal to the minimum absolute value in the list?
Yep
so like
.Where()
removes all elements where that is not trueyeah so it filters to the condition
new int[]{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
in this list
min abs value is 1
and the value of x is in the first iteration 1
yeah bro i'm so lost
with the logic too
like i was confused with
but i learned the first section is when you define x, next is the condition, and next is what happens when the condition is met
without Distinct() would it still filter?
Yeah
or do you need Distinct() and Where() together
so distinct removes dupes and where filters after dupes have been removed?
Exactly
shoot
You can chain LINQ methods almost at will
i'm basically new
beginner
i'm sixth form y12
next yr we gonna make websites so i gotta study c#
a lot
Well, lambdas and LINQ are definitely not beginner topics
i
have known lambda from time
basics of it
so to find the number closest to 0
find the smallest possible value in abs form
that's literally it
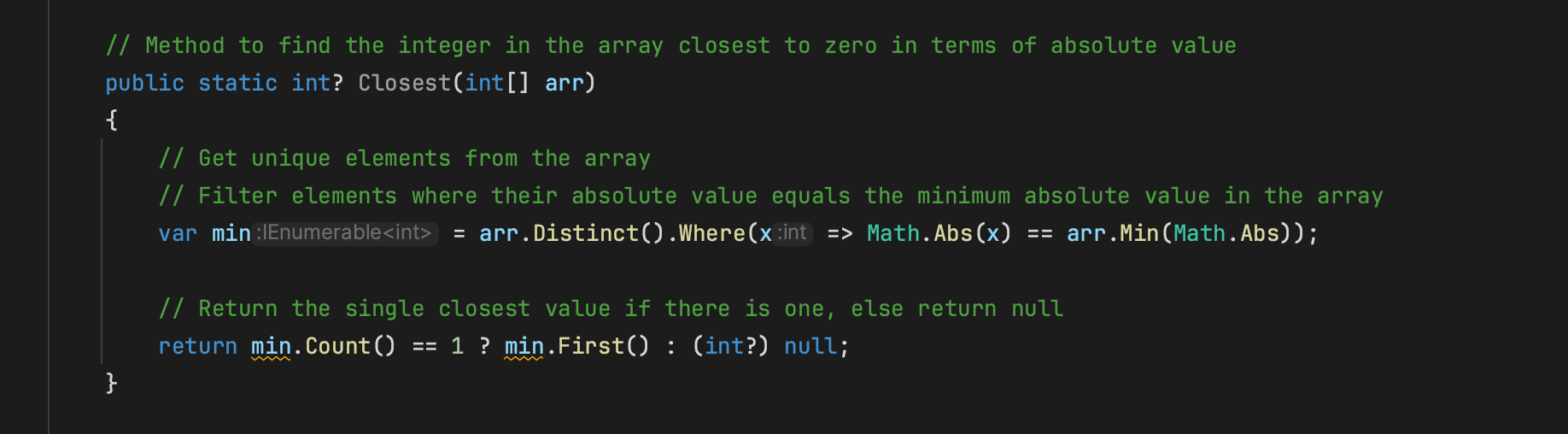
what's the point of all that when you're tryna find the number closest to 0
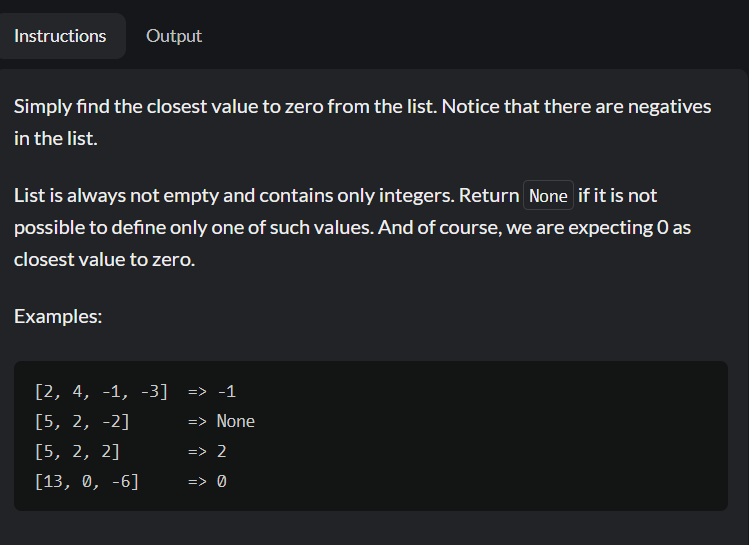
Am just explaining your answer, You never sent your qn
no i wasn't tryna be rude
no worries, send the question
what's min.Count() and min.First()
akhahmed
Quoted by
<@1153068032533483588> from #Mind explaining a code to me? (click here)

React with ❌ to remove this embed.
Angius
REPL Result: Success
Result: int
Compile: 542.876ms | Execution: 51.420ms | React with ❌ to remove this embed.
Simply find the closest value to zero from the list. Notice that there are negatives in the list.
List is always not empty and contains only integers. Return None if it is not possible to define only one of such values. And of course, we are expecting 0 as closest value to zero.
Angius
REPL Result: Success
Result: int
Compile: 541.726ms | Execution: 67.039ms | React with ❌ to remove this embed.
o
I highly recommend trying to run the code and observing the results lol
Learning by doing and all that jazz
why is he doing min.Count ==1
i know
i receive so many
Checking if the collection has only a single element
errors
cause they put it in a clas
s
If it does, then that element is the closest to zero
If there's more, that means there are more elements just as close to zero
sending my solution in a sec, i'll create a function
and explain in comments
public static int? Closest(int[] arr)
{
int closestAbsoluteValue = arr.Select(Math.Abs).Min(); // Find the minimum absolute value
var closestValues = arr.Where(x => Math.Abs(x) == closestAbsoluteValue); // Find values with the closest absolute value
if (closestValues.Count() == 1)
{
return closestValues.First(); // If only one closest value found, return it
}
else
{
return null; // If multiple values with the same absolute value exist, return None
}
}
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/look, good
i copied yours
💀
ofc
i'm not gonna use it tho
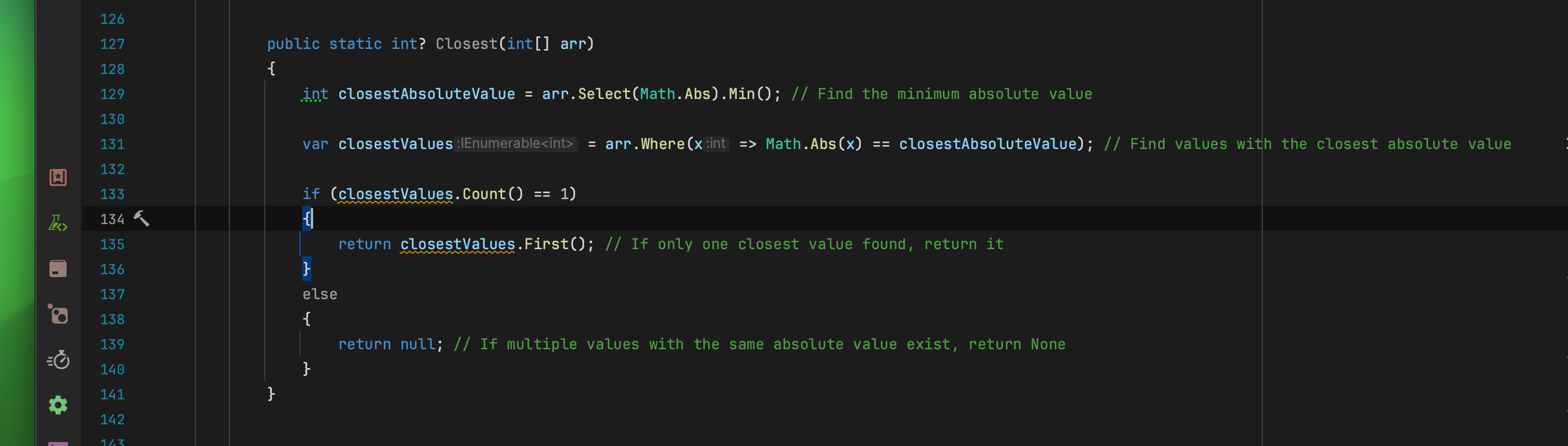
last thing i wanna know
why is he tryna find the values with the closest abs value
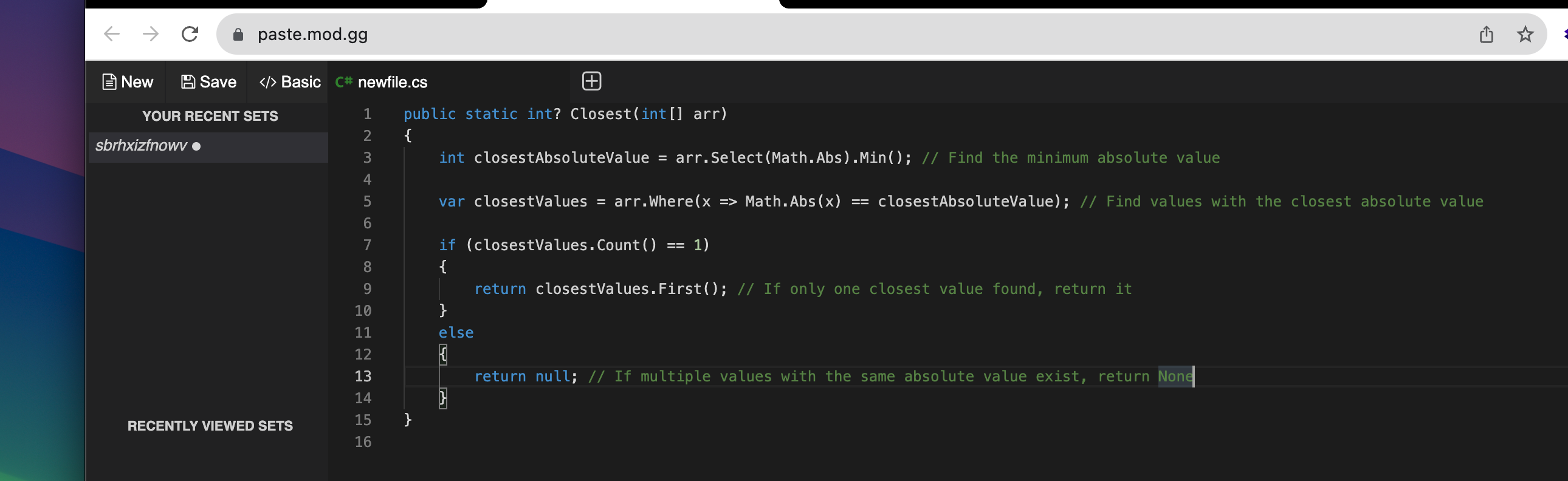
Because
-2
is as close to zero as +2
oh wait wait
So you need to treat negative and positive values the same, in terms of proximity to 0
So, by finding the values with the closest absolute value to zero, the function determines which number or numbers are closest to zero, considering both positive and negative numbers equally in their proximity to zero. If there's a unique value that's closest to zero, the function returns that value. If multiple values share the same closest absolute distance to zero, it indicates that there's no singular closest value to zero, so the function returns None.
shoot shoot shoot
public static int? Closest(int[] arr)
{
var min = arr.Distinct().Where(x => Math.Abs(x) == arr.Min(Math.Abs));
return min.Count() == 1 ? min.First() : (int?) null;
}
Distince() removes all dupes,
Where()... sorts it out so only the smallest value is left, there would be 2 values left if there was a negative variant.
IF there is either positive or negative, it will min.First(), and if there are 2 values with equal prox ( e.g. -2, 2 ) then it returns null?
why can't it be
Math.Abs holds all unique integers, and when == is set, it makes the list smaller
because basically a condition has been set
where it's equal to the min abs value
perfect, thank you so much :)))
!close
Closed!
(int?) means it's a nullable integer value, null return emply. which means (int?) will not work for non-numerical values,
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.