Repeater with only one record with Toggle active
I have a Repeater inside a form.
Every item has a toggle.
I would like to have only one active toggle for the complete set of items. It means that when i'm activating an item, I have to deselect (toggle=false) all the other items.
There is a way to do it?
I was investigating using "afterStateUpdated" but I didn't find a way to chenge the status of the other items.
Here's my code and a picture of the UI.
public function form(Form $form): Form
{
return $form
->schema([
Forms\Components\Group::make()
->schema([
Forms\Components\Section::make($this->makeTitle())
->schema([
Forms\Components\Repeater::make('exhibitionWines')
->hiddenLabel()
->relationship()
->schema([
Forms\Components\Select::make('wine_id')
->relationship('wine', 'name')
->native(false)
->required()
->preload()
// ->reactive()
// ->afterStateUpdated(function($state,callable $set)
// {
// })
->columnSpan(3),
Forms\Components\Toggle::make('in_masterclass')
->reactive()
->label('MC')
->inline(false),
])->columns(4),
...
public function form(Form $form): Form
{
return $form
->schema([
Forms\Components\Group::make()
->schema([
Forms\Components\Section::make($this->makeTitle())
->schema([
Forms\Components\Repeater::make('exhibitionWines')
->hiddenLabel()
->relationship()
->schema([
Forms\Components\Select::make('wine_id')
->relationship('wine', 'name')
->native(false)
->required()
->preload()
// ->reactive()
// ->afterStateUpdated(function($state,callable $set)
// {
// })
->columnSpan(3),
Forms\Components\Toggle::make('in_masterclass')
->reactive()
->label('MC')
->inline(false),
])->columns(4),
...
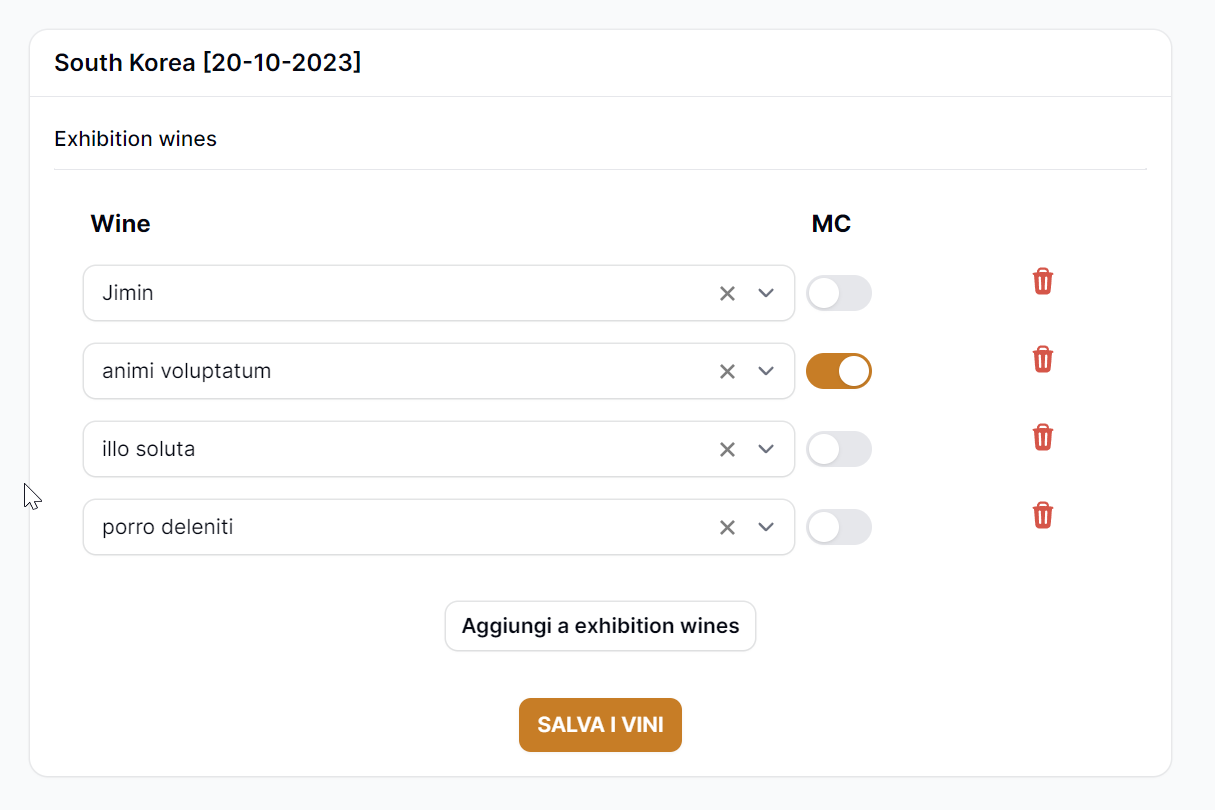
Solution:Jump to solution
At the end I was able to find a solution. Here below the code:
```public function form(Form $form): Form
{
return $form...
3 Replies
A repeater is an array of arrays so you would have to loop them all to unset them and then set the one you want.
Thank you Awcodes.
I was able to read the array you were mentioning, but not able to set value.
This is the last version of my code:
Repeater::make('exhibitionWines')
->hiddenLabel()
->relationship()
->schema([
Forms\Components\Select::make('wine_id')
->relationship('wine', 'name')
->native(false)
->required()
->preload()
->columnSpan(3),
Forms\Components\Toggle::make('in_masterclass')
->reactive()
->live()
->afterStateUpdated(function($state,callable $set, callable $get)
{
$items=$get('../../exhibitionWines');
$current_id=$get('id');
foreach ($items as $key => $item) {
if ($item['id']!=$current_id){
$set($item['in_masterclass'],false);
}
}
})
->label('MC')
->inline(false),
])->columns(4),
Repeater::make('exhibitionWines')
->hiddenLabel()
->relationship()
->schema([
Forms\Components\Select::make('wine_id')
->relationship('wine', 'name')
->native(false)
->required()
->preload()
->columnSpan(3),
Forms\Components\Toggle::make('in_masterclass')
->reactive()
->live()
->afterStateUpdated(function($state,callable $set, callable $get)
{
$items=$get('../../exhibitionWines');
$current_id=$get('id');
foreach ($items as $key => $item) {
if ($item['id']!=$current_id){
$set($item['in_masterclass'],false);
}
}
})
->label('MC')
->inline(false),
])->columns(4),
Solution
At the end I was able to find a solution. Here below the code:
public function form(Form $form): Form
{
return $form
->schema([
Forms\Components\Group::make()
->schema([
Forms\Components\Section::make($this->makeTitle())
->schema([
Repeater::make('exhibitionWines')
->hiddenLabel()
->relationship()
->schema([
Forms\Components\Select::make('wine_id')
->relationship('wine', 'name')
->native(false)
->preload()
->columnSpan(3),
Forms\Components\Toggle::make('in_masterclass')
->reactive()
->live()
->afterStateUpdated(function($state,Set $set,Get $get)
{
$items=$get('../../exhibitionWines');
$current_id=$get('wine_id');
if($state==true){
foreach ($items as $key => $item) {
if ($item['wine_id']!=$current_id){
$set("../../exhibitionWines.{$key}.in_masterclass",false);
}
}
}
})
->label('MC')
->inline(false),
])->columns(4),
Forms\Components\Placeholder::make('savePersonal')
->label('')
->content(new HtmlString(
Blade::render('
<x-filament::button type="submit">
SAVE
</x-filament::button>
')
))
->extraAttributes(['class' => 'flex justify-center']),
])->compact()
])->columnSpan(2),
])
->columns(4)
->model($this->entity)
->statePath('entityData');
}
public function form(Form $form): Form
{
return $form
->schema([
Forms\Components\Group::make()
->schema([
Forms\Components\Section::make($this->makeTitle())
->schema([
Repeater::make('exhibitionWines')
->hiddenLabel()
->relationship()
->schema([
Forms\Components\Select::make('wine_id')
->relationship('wine', 'name')
->native(false)
->preload()
->columnSpan(3),
Forms\Components\Toggle::make('in_masterclass')
->reactive()
->live()
->afterStateUpdated(function($state,Set $set,Get $get)
{
$items=$get('../../exhibitionWines');
$current_id=$get('wine_id');
if($state==true){
foreach ($items as $key => $item) {
if ($item['wine_id']!=$current_id){
$set("../../exhibitionWines.{$key}.in_masterclass",false);
}
}
}
})
->label('MC')
->inline(false),
])->columns(4),
Forms\Components\Placeholder::make('savePersonal')
->label('')
->content(new HtmlString(
Blade::render('
<x-filament::button type="submit">
SAVE
</x-filament::button>
')
))
->extraAttributes(['class' => 'flex justify-center']),
])->compact()
])->columnSpan(2),
])
->columns(4)
->model($this->entity)
->statePath('entityData');
}