❔ In need of a way to process inputs to produce outputs, other than using hardcoded if statements
Hi all,
I currently have a program that prompts the user to ask a question, then based on the parameters in the user's input, my code will output the answer to said question. However, as of right now I have it so my code is just using a lot of if statements and structured in a way which is
and I essentially have it all hardcoded for each input however this takes up so many lines of code so I was wondering if anybody could help me find a more efficient way of carrying out this process.
297 Replies
Could maybe store it as a list of classes?
Could even store the questions as JSON
hmm i think i understand
Yeah something like that. id have the parameters be an array, in the record, as well as the answer, possibly provided through a method that lets you extract data from the user question and just loop our Question instances to see what matches
surely this will also be alot of hardcoded lines tho also?
No
Just those 3
how come
Store data in a json file
Angius
Store data in a json file
Quoted by
React with ❌ to remove this embed.
but in the code above, doesnt that only write the answer for when the input contains parameters 1 and 2 for the question, what about for when it contains parameters 3 and 4 for example
thats why you use an array instead owo
ok so lets say i use a 2d array
or a 1d array
surely i still have to hardcode for every parameter
sry btw i am new so i might not be 100% understanding it correctly
There
so the top code isnt in visual studio it is in a json file
It is a part of the project, yes
sorry i have never used a json file before to hold text
how do i use the data.json to make it work with the program
Like I wrote
Place the file next to your
.csproj
Mark it to copy always or copy if newer
Donewhat do i paste it into a new text file then put that next to the csproj in the folder
The code I posted is literally a working sample
Well, almost, you'll need to get the actual
input
There, now it's a working samplebut when i psate it into vs it comes up with a load of errors
Like?
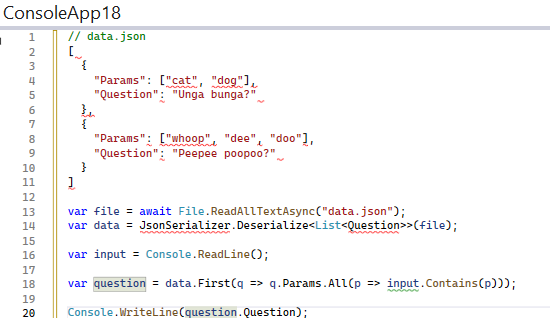
bunch of syntax eorrs
The JSON data is it's own JSON file
yeah how do i do that
Make a file?
yh do i put it in the same folder as my consoleapp is in
Yes
As I said already, next to the
.csproj
ok done
but now its sayins jsonserializer dosent exist
and Question coudlnt be foud
Use quick fixes to import the necessary namespace
And, right, you will need to create the
Question
class
Or a recordok i used quick fixes
now its saying object doesnt contain a definition for First
and theres no quick fixes that solveit
Quick fixes again
You need
System.Linq
namespacei dont understand wdym
Use the quick fixes on
First
it only says convert to top level statemetns
Just like using it on
JsonSerializer
included System.Text.Json
namespace, using it on First
will include System.Linq
Then include the namespace manuallyso shall i make a new class for First
No, why?
Include the namespace
what do you mean inclued the namespac
using System.Linq;
yeah ive typed that but visual studio greys it out and says its unnecessary
Show your code
and your error
do u want it in a screenshot or typed out
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/And maybe a screenshot of the errors
Well

Your
Question
has no propertiesyeah i know
And you're trying to access its
Params
and Question
properties
Can't access what doesn't existbut i thought they were stored in the json file
hwck is Params anyway, i thought it was some json thing i didnt know about for a moment
The json file just stores the data
ok
yeah, we're in C# baby, everything needs types here
so what do i do
and also how do i fix the First error
* The record has no properties
* That's an issue
* How do I fix that issue?
Take a guess
Gotta add the Params and Question properties to your record so c# knows how to interpret your json file
Deserializing from Json will put data from a property of name
X
in the Json file, into a property of name X
in whatever class you give itoh
so it like converts it
json is rly just a way to store data in a (very commonly used mind you) format.
Deserializing is essentially grabbing the data and putting it into your class
so whats the point of storing it in a json file if it needs to be redefined in c#
Because it's easier?
you only define the properties in c# rather than all the actual questions and answers
ohh
if you have a lot of data, this will save a lot of hardcoded crap
yeah
plus json is used all over, its very useful to learn it, even if this particular application doesnt rly "need" it
Would you rather define one class and data in the Json file, or still define that class and the data as
so how do i define the properties of the data in my json file inside my question record
You wanted to avoid hardcoding the data in your source code
That's how
Angius
Could maybe store it as a list of classes?
Quoted by
React with ❌ to remove this embed.
Refer to this
what so like this
that might work with modern c#? im not sure
Of course it will
You will need to adjust the parameters though
Look at the Json
There's
Params
that's an array of strings
And there's Question
that's a single string
Those will have to be the properties of your recordso ?
Yep
yeah thatll do :3
so how do i fix this error with data.First
you still get it?
yh
might need to make it a List<string> instead of array
Nah
.First()
works on IEnumerable
CS1061 'object' does not contain a definition for 'First' and no accessible extension method 'First' accepting a first argument of type 'object' could be found
An array does implement
IEnumerable
So
For some reason
data
is of type object
Huh
What if you replace that var
with List<Question>
?
The var data
with List<Question> data
man this third person debugging shit is weird, feels like a skill on its own
i had to change the name of the record to QuestionChooser because the string parameter was called Question btw
Ah
5head
Maybe that was the issue, yeah
Good thinking
but im getting more errors now i changed var to list haha
it says data is already defined
Do you have another variable named
data
somewhere?yeah
but it was like that before
and the error didnt show
So you... declared a new variable called
data
Instead of changing the var
here to List<Question
like I said
Or, well, List<QuestionChooser>
nowno thats in teh code above
Show your code
my code now as it stands is
yeah you have two variables called
data
cant do thatsebt#6912
Quoted by
React with ❌ to remove this embed.
It was named
question
Why'd you rename it?wtf my bad
i didnt mean to
idk how that happened
no stress lol
im still getting this error now though even after renaming it back to question and having it as List<QuestionChooser> question = ...
Because it's a
QuestionChooser
now
Not a list anymore
You can just use var
tbhok i put it back to var
yeah var auto figures out what the type is. the problem before is that you tried to give two different variables the same name. compiler gets brainzapped by that
yeah
i gotchu
but the compiler is still giving me the same error as before
what error was that?
CS1061 'object' does not contain a definition for 'First' and no accessible extension method 'First' accepting a first argument of type 'object' could be found (are you missing a using directive or an assembly reference?)
Show code again
dafuq lol
This should, by all accounts, work
data is List<QuestionChooser> which certainly has a First()
can you try
data.First()
without the magic, see if that does the same thing.
id also put a breakpoint to check what it thinks the value of question
is at that point
just for debugging purposesyeah data.first();
still the same error
if you hover over "data" what does it say the type is
object?
lolwhy
what
It has no right to be
object?
man i dont have my code setup so i cant even see wtf is going on rn
fucking yolo cast it as a list? XD idk fam
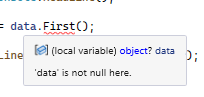
i dont know what to do lol
ok fuck this im booting into windows lol
this is insane
haha
Wait
Crazy theory
Did the quick fixes on
JsonSerializer
perhaps create a new class named JsonSerializer
?ohhhh
Do you have any other files in the project, any other classes?
yeah it did
thats gotta be it hahaha
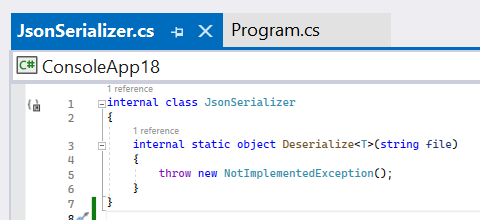
i didnt see the using statement i think
Ah
Yeah delete that
the entire class>
?
The whole file
the entire JsonSerializrr file
not ur actual code
ok done it
Now,
JsonSerializer
will be highlighted in red
Use quick fixes
And pick the fix that says to include the required namespace
Or include the namespace manually
using System.Text.Json;
ok done
it fixed it
now im getting another error but for questionchooser

Becase the property is
Question
Not QuestionChooser
usually u ctrl+. and see if u can import the thing, cuz what it did now is assume JsonSerializer is a class you made rather than a .NET class
ohh
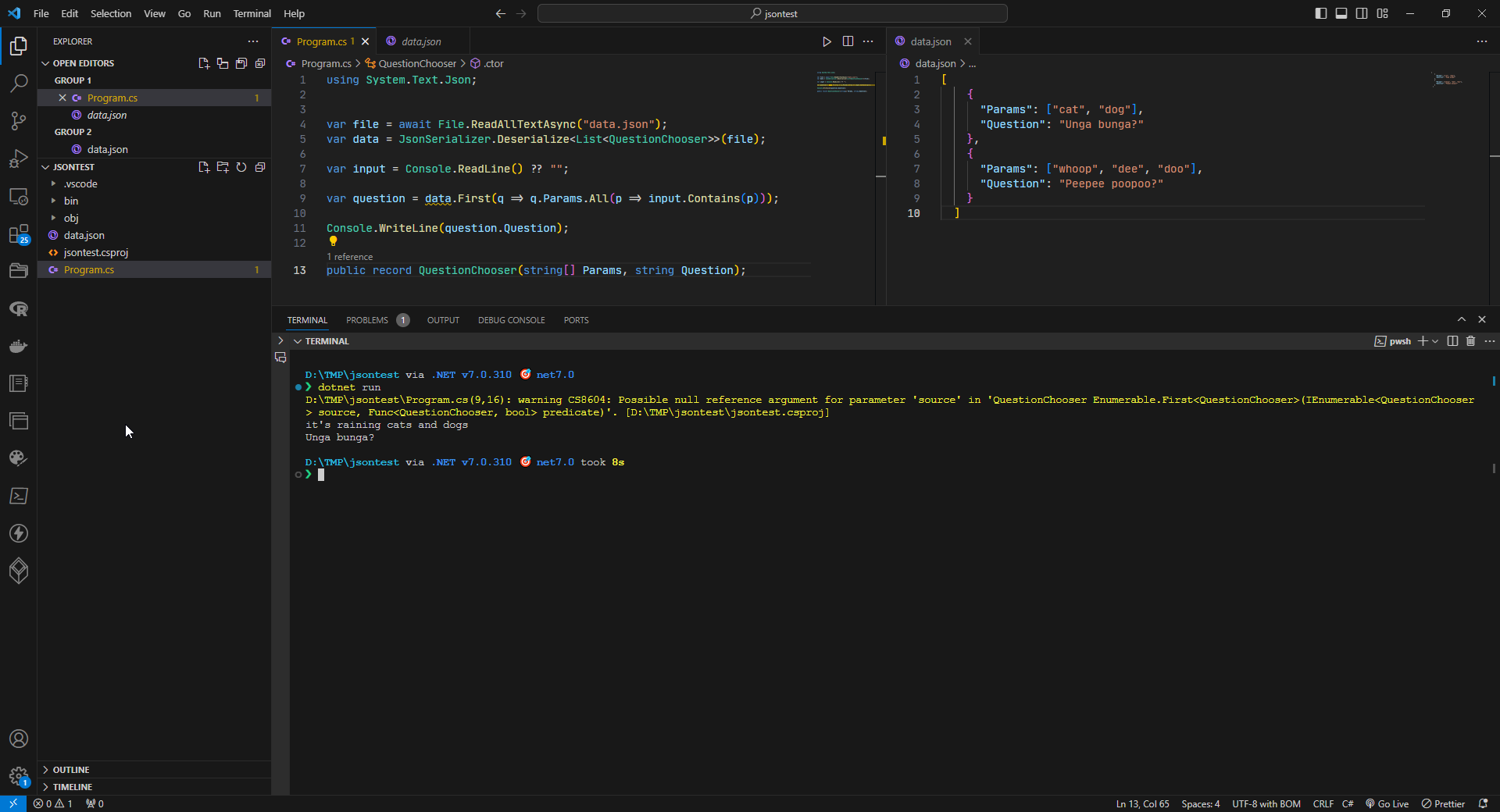
Works on my machine
now i can run it but when it runs it says it coudlnt find the fil yet i have the file in ConsoleApp18 folder next to the csproj
yay thats gooder
Is the file copied?
Right-click it, open its properties
Select copy if newer or copy always
the path it tries to read is relative to where ur exe is, so /bin/Debug/muh.json
copy if newer essentially puts the file alongside ur exe whenever u compile
where do i find copy if newer
im on windows 11
vscode or vs2022
what
can i not do it from properties
what code editor do you use? visual studio 2022 or visual studio code
visual studio 2022
ok, u dragged the file into your project in visual studio?
u should right click the file in the file list in visual studio and click properties
The json file should be there in the solution explorer
there youll be able to set it. i dont remember what the specific property is called tho..
its in the solution explorer
but its one of em, it opens a list that lets u pick what happens with the file

i think
if this is the solution explorer
nah its gotta be on the left or right where u open your files
bro this is hard xD
here
???
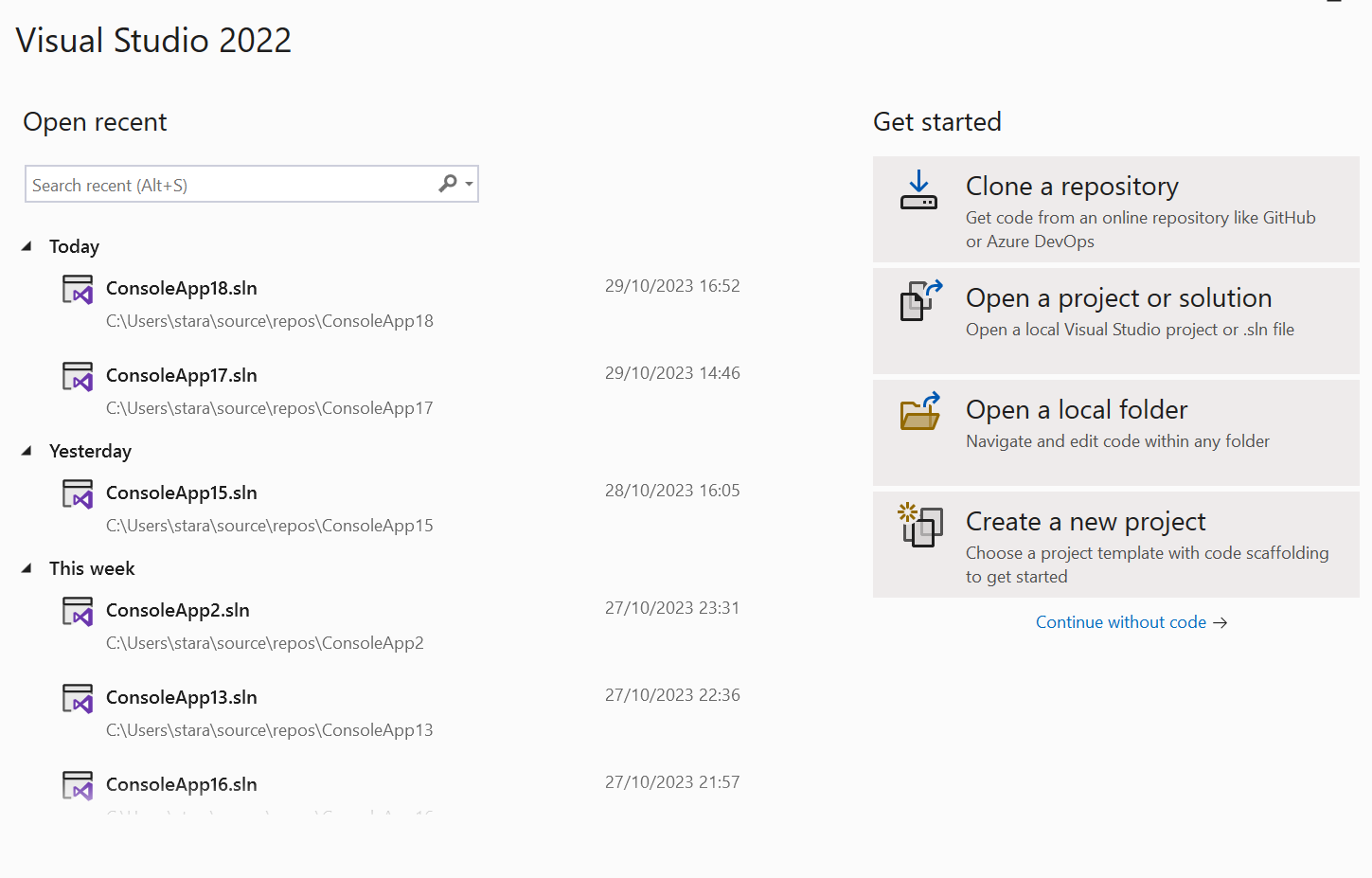
nah
it should basically be a file in your project
leme fimd a screenshot
k ty
this thing
it should be in that list alongside program.cs
where
what thing
i dont think the image came thru
oh
ah ok
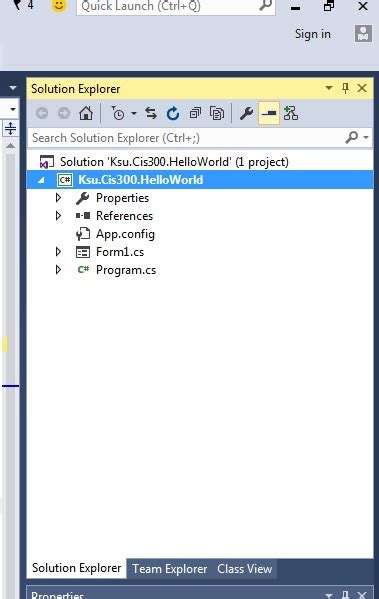
so shall i set to copy if newer
yes :3
and then i think ur there 😄
dont worry, it gets way less annoying once you get used to it
its stuff we all ran into getting started
ok i set to copy if newer
and im still getting the same error
lol
wtf
if u go to the project folder in windows explorer, in the bin/debug folder
do u see the json file there?
screenshot ur solution explorer if not pls
wheres the bin/debug fodler
rightclick ur project and "open containing folder" or something similar
in there should be the bin folder, in that the debug folder
yh
its in there
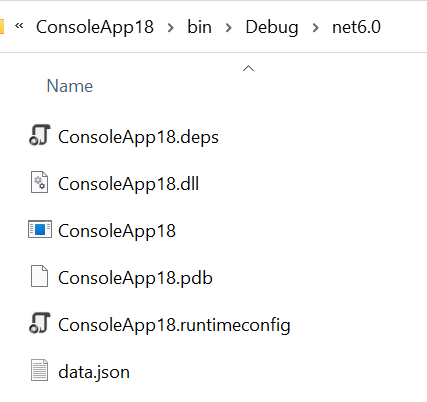
and your code also says data.json right?
yeah
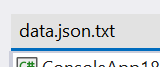
and what was the error exactly?
or is the txt
ihh
not making it work
yeah txt bad
what i did was make a new text file then copy and paste the info into there then save it as data.json
first of all, in windows explorer u wanna enable show extensions
how do i do that
How do I show file extensions in Windows 11?
Show file extensions for known file types in Windows 11 by following these steps.
yeah
ok
i gotchu
then rename the file in ur project root (not bin/debug)
then remove the wonky file from ur project
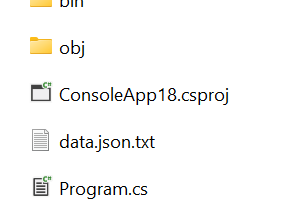
rightclick project, add file existing file and select the proper renamed file
wait
so i renamed the file
now its a json
actually
yep heh
then where do i remove the file
actually, if visual studio doesnt complaina bout ot, ur good. just set it to copy if newer again
sorry lol.. im used to older version of visual studio
wait my solution explorer just disappeared
how do i access it again
is it tabbed or did u click x
nvm i got it
alright
im getting fucked by visual studio right now
ok so i changed it and made the new json file to copy if newer
yeah, been there lol
now im getting anotha error
whats the error
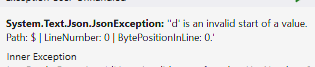
alright thats good, thats just json syntax being fucked
print ur json again please
wym
theres an error in your json file basically
so it cant parse it
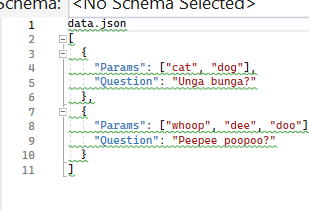
line 1 shouldnt say data.json
thats why it cant read it
oh
ahh yeah ik the problem
it was commented
thats why its there
but i removed the // not knwoing what i was doing
heheh yeah its pretty strict
usually you dont rly handwrite json either but it does unfortunately seem to be the best way to deal with data like this
its more often used to communicate data between a webserver and a browser, or programatically written for data processing
ok
so
the code runs now
ayy nice
but i dont know if it actualyl works
because it prints nothing at the start until i input something
and then it says
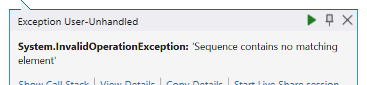
so i think this is to do with the code now rather than the file reading
but i dont know what im supposed to input
@ZZZZZZZZZZZZZZZZZZZZZZZZZ did you have to input something in order for the code to print the contents of the json?
its because First() must have something
otherwise it crashes
try FirstOrDefault() then it will return null instead of crash
firstordefault gives me this error when i input something
but you would have to do
if (question == null) Console.WriteLine("no match")
or something along those lines
yeah, cuz without the null check, it will still crash
basically it says it didnt find a match, which is probably a code problem, but we still need to have the program not explode when an undefined question is asked
do you actually know what .All() and stuff does? i feel like its not the best approach for a beginner anyway if ur trying to learn
i have no clue hahaa
hehe fair
but im this far i really want to make it work
yeah of course
using these ideas you know
but we can make it more comprehensible if u want
linq is amazing, but if you get thrown right into it as a beginner its super confusing
fair enough
id recommend you write your own implementation. knowing that data is a list (or array) of QuestionChooser.
QuestionChooser has properties you can access using x.Param and x.Question
i think you're better off using for loops and writing it yourself, but ill help u if you need help ofc
i would love some help
when i tried to do this myself
i just end up hardcoding every possible outcome
because i cant think of another way to do it
alright, do you know how arrays and loops work?
yeah
oh and do you know what properties on a class or record do?
like have you used it before?
just to know how much i need to explain
i have used classes and records before yeah
in the code
bascially
perfect
alright, so data is a
List<QuestionChooser>
which is basically just a fancy QuestionChooser[]
.
so what we can do is foreach (var qs in data)
for starters and evaluate each to see if our input is usefulok
there are 2 parameters for each question btw
essentially 8x4 questions that can be asked
right, but lets try to stay focused on the scope we're in or even simple processes get confusing
ok my bad
well its fine, just trying to communicate my way of thinking
yh i gotchu all good
right now we have qs which is an instance of
QuestionChooser
theres a few ways we can do it, but lets go with this one:
we another foreach inside of there to iteratr the Params
on qs
so foreach (var p in qs.Params)
and then we can check if input.Contains(p)
normally i would put it in a function that we just return a bool out of at this pointok niec
and the code will automatically read every instance in params from the json file?
yep because we're looping over everything
how do i say not instance but like each element of the array
yeah
ok nice
so that saves me from having to do if inputcontains x or if inputcontainsy because this loop will see what the input contains exactly
once you get the results, it depends what you wanna do with it really
yeah
do note, you wanna break out of the loop in a situation with a lot of data after you found your match
thats why i would recommend creating a seperate method for it that returns whatever datatype you want as soon as the match is found
returning out of a method breaks the loop. otherwise you gotta break out of two loops manually which is slightly annoying
yeah that makes sense
my description isnt fully accurate either, but i think youll figure out where the bug is, and if not, feel free to ask
ok im gonna make something to eat then i will come and write the code with ur help
thanks
i probably will encoutner some problems tho so just letting u know lool
heh alright, i do gotta hop off for a bit in like an hour but ill be around most of the night
ok nice one thank you
but yeah thats fine bro, dont sweat it, we've all been there
and it is hard when you just get started
yea
ive made a new class in my main program in which im attempting to implement the functions that will cycle through the file and output the answers based on the users questions
do i make a function within this class that ill then implement the necessary loops into in order for it to successfully check the users input and then compare that to the data in the file
What's in the screenshot is quite literally all I have
The input in the terminal is my entire input
so u have to input:
it's raining cats and dogs
in order for it to return unga bunga
ohh
because the code says
the input has to fulfill all the parameters present in the file
so u have to input cats and dogs in some sort of way
to get unga bunga returned
yeep so if u input stuff that doesnt match, it wont work. if u put in both inputs it should
but i dont think it helpful to paste code you dont understand. linq is very magical, but if you cant implement it without it, youre missing essentials
yeah fair enough
so i was trying to do this without linq
do i approach it in a way that basically uses for loops and then put that insdie a method and then use that method to check user input?
because i have no clue how to do it
yeah sounds about right, or foreach loops for convinience
i can prototype it for you if its too hard
if that wouldnt be too much of an inconvenience i would seriously appreciate just like a draft or basic frame for it
just to clarify, when both params are in our string, we want to console.writeline .Question right?
im already writing it lel
yeah
haha
and so it would be like when paramx and paramy are in string, print question and its corresponding answer 1
and then if paramz and paramq are in string print qusetion and its corresponding answer 2
but i will store both the q and a in a json file
together
then output it as 1
or output it as 2 but output them both together
thats the general idea. again, this is not good code, ZZZ's solution is way more elegant but it's important that you know what's going on.
if you're confused about something, feel free to ask
thank you so much
so this is a way to solve it without using this linq thing
ill explain the linq solution im a bit too. its kinda chaos rn here lel
hahaha
Alright so lets first cover how LINQ kinda works.
For now, all you rly gotta know is that it uses some magic under the hood and it's extremely useful to write clean code.
Mind, if you need very high performance, it's not always the best, but for most usecases its ok to use.
Lets for use
.OrderBy()
as an example. it basically sorts an array.
Using it looks like this: QuestionChooser[] sortedArray = myArray.OrderBy(x => x.Question);
This would order your array alphabetically by Question.
the x => x
threw me off at first too. What it does is roughly the same as a foreach (QuestionChooser x in myArray) { /* sorting logic here */ }
loop
x is basically the instance we're currently doing stuff with in our loop. You could also call it something other than x like qs
then youd have myArray.OrderBy(qs => qs.Question)
it's basically just the variable name in the loop, with me so far?so is the code here saying something like foreach qs in question, and then using the OrderBy function for each qs
kinda, except you dont need to do the foreach yourself. OrderBy() takes care of pretty much everything.
You just gotta define what you wanna order by, in this case QuestionChooser.Question
and the way to do that is by using the arrow like
.OrderBy(x => x.Question)
ah ok
so u define what ur ordering inside the orderby by using x=> x.whatistobeordered
then you get back your array of QuestionChooser, but ordered alphabetically by the values in QuestionChooser.Question
yep
i see
So if we break down the original linq query to your problem
What happens here is data.First() will says we will grab the first thing that matches our conditions. defined inside First() and assign it to our var question
ok i get that
q => q.Params.All(...)
This says that we're looping over Params in the instance of QuestionChooser we're currently evaluating, and that all conditions must match.so its like a foreach loop and it cycles through every parameter?
yeah exactly
its just kind of a shortcut
and it does our little innerMatchCount thing internally
It checks if
.All()
elements from the collection satisfy the given conditionfinally we have
p => input.Contains(p)
which again takes the parameter provided by All()
which was iterating p.Params
and we check if input contains the specific param
under the hood, .All and .Contains returns a bool to the caller (which is the linq query wrapping it)
makes sense kinda?yep makes sense
awesome
although im not sure if im gonna use it over the non-linq version for this program
It shows up well with simple numbers
yeah thats fine, its a lot of compressed stuff and when you're new its hard enough to read code at all so
but its certainly good to add in your toolkit down the line
oh for sure
Angius
REPL Result: Success
Result: <>f__AnonymousType0#1<int, IEnumerable<int>, bool>
Compile: 597.827ms | Execution: 124.321ms | React with ❌ to remove this embed.
this example zzz provided me is so nice
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.