How to express "children" or "parent" relation with typescript?
Let's say I have a
country
and region
tables. A region belongs to a country, so on the region
table, there's a foreign key country_id
to the country
table.
The question is if it is possible to have a Country type generated like this:
The goal is to be able to query a region and join it with the country table as well. Thank you!9 Replies
Current schema attempt:
You could do that, but it's usually all the way around. I don't know what are you app requirements but this what people would typically do:
But you can make it however you want
Sure. But how can I do it, either way?
It is using the relations()?
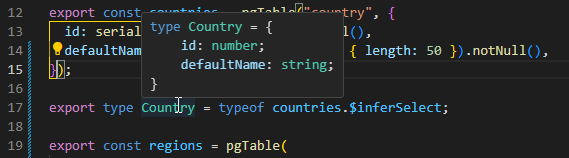
The types you are looking for are not autimatically exported for you
You either have to construct them manually or get them from the query you'd do
for example
Will be the type you're looking for
Or you can construct it like:
I tried the first approach without success. Looks like I got a
never
type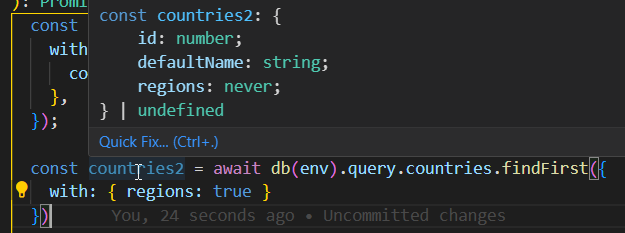
Is this expected?
Nope, are you defining your relations the drizzle way? https://orm.drizzle.team/docs/rqb#declaring-relations
Drizzle Queries - DrizzleORM
Drizzle ORM | %s
Checking 🔃
That was it, thank you!!