Conditional Form Input and Table Column
I want to create two types of data: 'image' or 'text.' That's why I've structured my code this way. Is there a more optimal approach? Additionally, I'd prefer to hide the 'Status' field on the Create form and reveal it in the Edit form. Please provide me with ideas on how to display input values in a table, where the value type can be either text or an image.
Solution:Jump to solution
This code solved my problem.
```php
<div>
@if ($getRecord()->value_type === 'text')...
26 Replies
Select::make(‘status’)->hiddenOn(‘create’)
The callbacks for the forms have injectable params for the $context or $operation that you can use for display purposes.
Specifically https://filamentphp.com/docs/3.x/forms/advanced#injecting-the-current-form-operation but read through the whole page.
Perhaps I didn't understand you correctly. I'm seeking suggestions regarding the conditional table column. In this table section, I aim to dynamically change between 'TextColumn' and 'ImageColumn' based on the value type.
I don’t think that’s possible.
It goes against the fundamental html spec for a table.
Yes! I know, it's not possible. But What should I change to done this type of condition with filament?
Form value can be image or text. And why it's not can be show in list of table?
Similar question asked earlier today. https://discord.com/channels/883083792112300104/1162755489436794931
Actually, I don't understand the custom 'Curator' column. Do you have any information about it? I found only custom column in filamentphp documentation.
It’s not an issue with the curator column.
But Curator is a plugin, it’s not part of core so it won’t be in the filament docs.
It’s covered in the Readme on the Curator GitHub repo.
Do you think? this plugin can solve my problem
But the plugin doesn’t have anything to do with trying to make conditional columns.
The curator column is just a stand column that uses the storage facade and the media table to render an image. But your trying to conditionally show a whole different column on a per row record. Tables just don’t work that way from a programmatic standpoint.
Should I try with filament custom column?
There’s not really anything to try. The same column can’t have different column types.
So you're saying that column types can't be selected based on the value type?
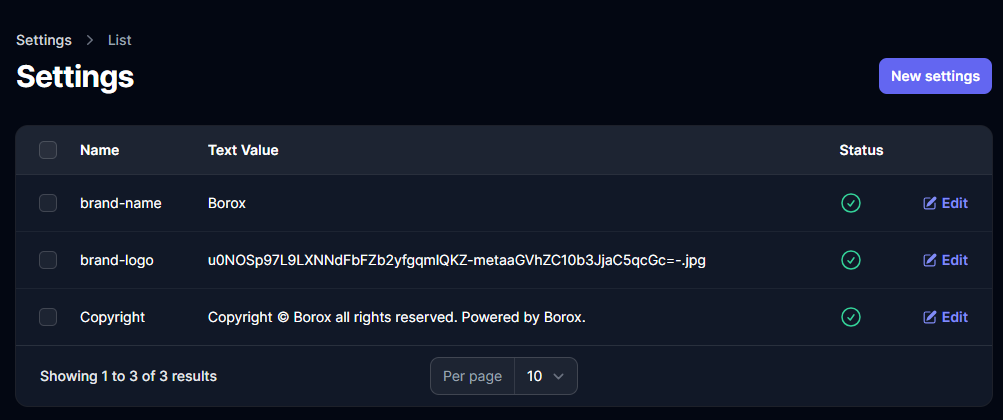
I’m saying that having an image, Boolean or text column, in the same column for a table is technically a violation of accessibility and why it’s not really supported.
You could potentially do a custom column that parses the state and returns a different html output.
The value of a file field is a string and the value of a title is a string, how could it possibly determine what type of field to show if they are both strings?
I found a way with custom column. But I can't call here the image tag with src attribute.
And that’s my point.
There’s no reliable way to determine from the state of the field which is a string if it should be a text or an image output which needs the storage facade.
Best you could do is check if a file exists with the storage facade and default to a text output. But that could fall apart pretty quick with curator which isn’t a string but a reference to another model on a different table.
Solution
This code solved my problem.
Ok
Feels brittle to me, but if it works for you. Great. 🙂
At least this is enough for me, I will definitely update if I get a better solution later.
Hi everyone, I'm experiencing an issue where the image thumbnail is not displaying when I view/edit it, but it appears on the front page (rows). Below is my code, please provide guidance.
FileUpload::make('image')
->enableOpen()
->enableDownload()
->label('Company Image')
->disk('cloudinary')
->visibility('private')
->columnSpanFull(),
What is the reason of this code "->visibility('private')"?
I was just experimenting with various methods, and when I removed 'visibility('private'),' the image still didn't appear.
First, you should take your code simple to take the view.
May be you forget to create symbolic link of laravel storage. You should run this command on your project.
I'm using Cloudinary, so I'm not using a storage link.
Is this a hosting server?
Rich Editor can also help solve the creation problem. I can upload images or text using the Rich Editor. But how can I display this data in a list or table?
I have a similar scenario but I needed to use Text and Select columns, so I did this that worked as expected. Hope it helps:
In your case: