Enabling HMAC for in-app with C#
Hey guy's im setting up HMAC for in-app and endpoint
https://api.novu.co/v1/widgets/session/initialize
is giving me a 400 Bad Request "Please provide a valid HMAC hash" and I was wondering if there's some obvious error im making when generating it
I'm basically using the React example from the docs:
21 Replies
The novu api key, app id, and the subscriber id seems to be correct
Unfortunately, I could help in nodejs 😅
Not sure if your above .net code is correct
So I fiddled around with it and turns out that it was happening because the Convert.ToHexString generates it in uppercase, converting it to lowercase fixes it
May be worth adding to the docs that it should be a lowercased hex hash @Pawan Jain
@Jelle, you just advanced to level 3!
@Jelle : Are your issues fixed? I am facing the same issue, I did the lowecased hex hash.
My last message got it working for me
Here is my implimentation, Do you see any problem?
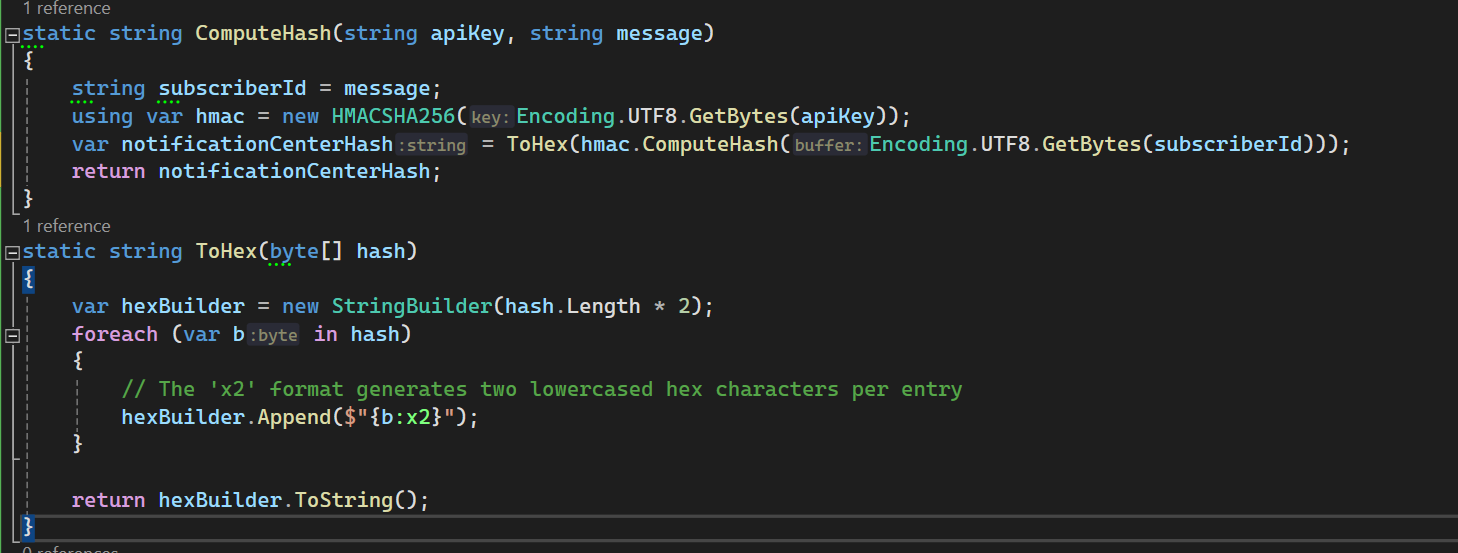
@dhruv.barot, you just advanced to level 1!
This is my implementation,
ComputeHash(string)
is called with the subscriberId
I don't really see anything wrong in your code though, looks the same
Let me check if it still works in our app lmaoThanks, Kindly check
Yep it does
Are you sure you're using the correct values everywhere?
And that it's sent correctly to the novu api
curl --location 'https://api.novu.co/v1/widgets/session/initialize' \
--header 'Authorization: ApiKey 17e6db6160cXXXXXX' \
--header 'Content-Type: application/json' \
--data '{
"applicationIdentifier": "F_5Gu0excnDH",
"subscriberId": "cbe4b020beed7774dcb298f87186e4e9bd440164b2ae5538c53543dee5c4ad1e"
}'
Do you think the curl is correct?
Yes, recheck all values and it's correct
Youre not sending the hmac hash though
Can you please send curl or any implimentation details for initializing the session endpoint?
Im pretty sure you just POST this
You're not supposed to send your api key over from the client
Great thanks: It's working
@Pawan Jain : I am happy to add documentation for session initialize API.
Sure @dhruv.barot feel free to ping us here in this thread or @unicodeveloper if you need any help 🙂
Hello! Could I check how do you all pass the generated hmac to the client in terms of single page application like react?
To pass the generated HMAC to a client in a single-page application like React, you can generate an HMAC encrypted subscriberId on your backend, and then pass it along to your React client-side application. This approach ensures that the subscriberId is securely encrypted using your secret API key, protecting against impersonation by malicious actors.
Here is an example of how the HMAC can be generated on the backend:
After creating the HMAC, you would pass the subscriberId and the generated subscriberHash to the client-side application and include them when initializing the <NovuProvider> component:
The subscription to notifications will not be operational if you have enabled HMAC encryption and then fail to provide both subscriberId and subscriberHash to the <NovuProvider> component.
Ensure that you enable HMAC encryption in the admin panel settings of your Novu account.
The actual HMAC generation should occur on a backend server where the API key can remain hidden. Only the resulting HMAC hash should be sent to the client.
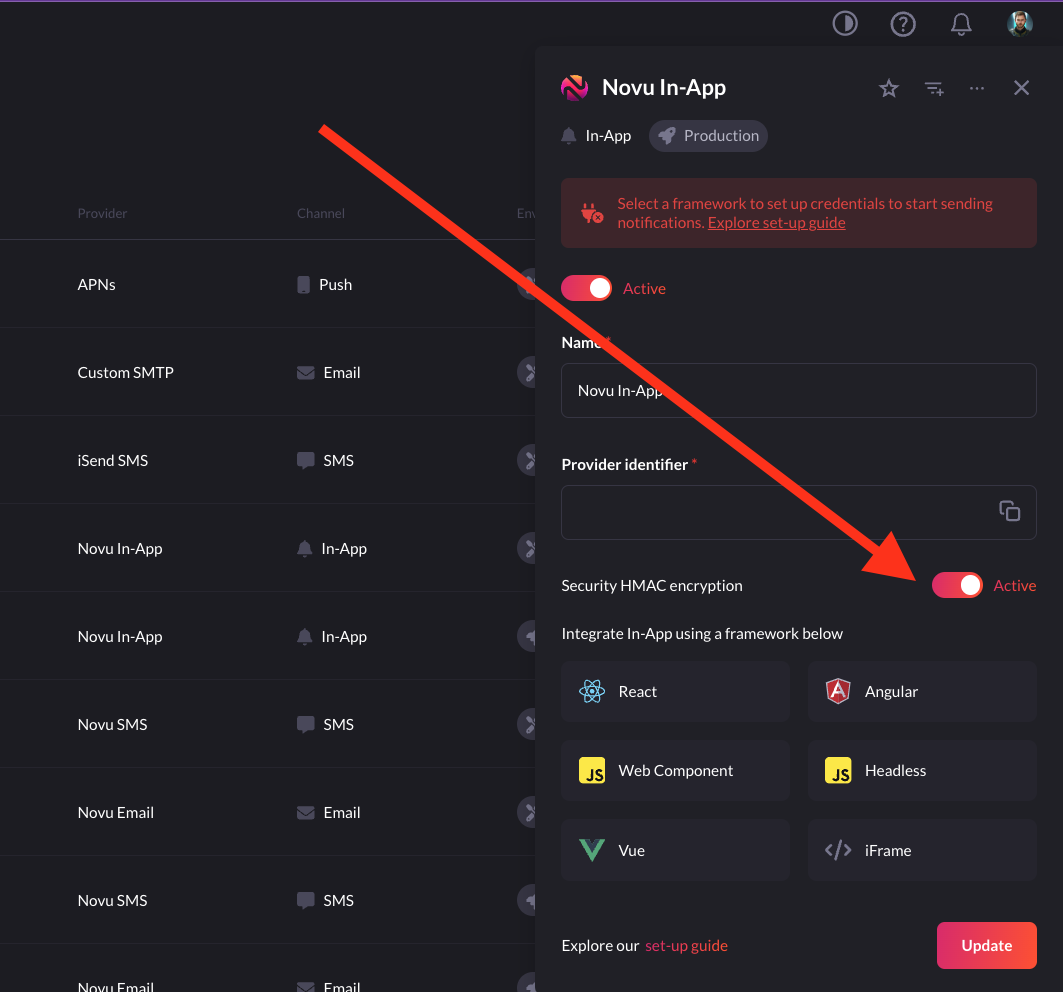
@Jelle Let me know if you need more help in this issue.
I fixed it with this