Database Notifications Slideover Custom Model
Hello,
I love the
@livewire('notifications')
panel, and have almost everything working correctly... but how do I use a Model (with the Notifiable
trait on it, of course) that isn't User
?
I tried doing an override of Filament\Livewire\DatabaseNotifications
like so:
And then putting it in the AppServiceProvider:
But no luck; the slideover panel is still showing for the logged-in User instead of my other Notifiable entity.28 Replies
I could be wrong, but I think DB notifications have to be scoped to the user. I'm sure that's a laravel thing.
They actually don't. They can be sent to any model with
use Notifiable;
on them, and the database is structured as such. Filament can also send Notifications to the proper model like so without any issue: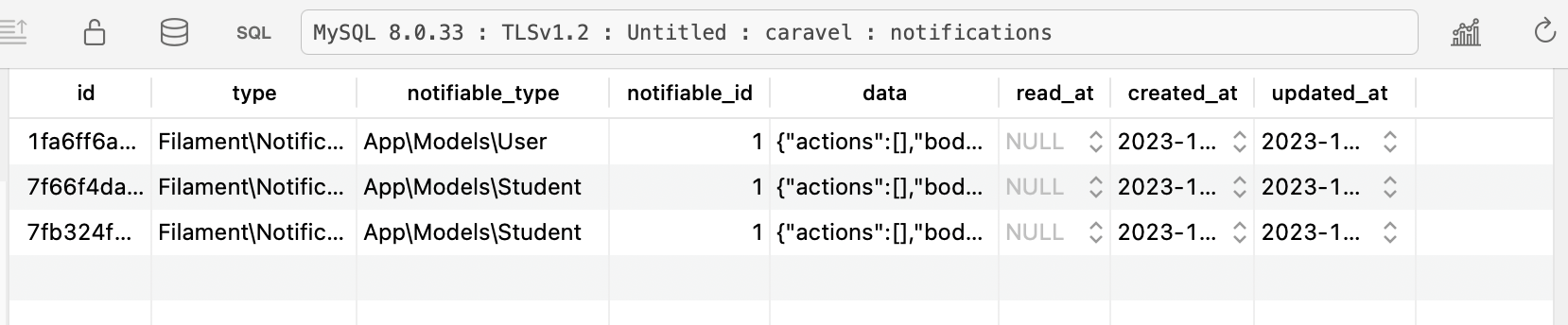
ok, so you're saying that when you are logged in as a student it's showing you the User notifications, instead of the Student notifications?
Exactly. It's a little tricky because Student is an active property underneath User in my case. So, a User might have logged in, but not have selected a Student. Think like Netflix - you can be logged in without necessarily being in a profile. So I want to feed the User model in if there is no Student, and a Student model if there is one. However, that doesn't even necessarily matter when I can't figure out how to feed anything other than a User in anyway.
If I can figure out where to set what model I want to display the notifications for, the rest is easy.
But a Student isn't the authenticated User, right? maybe i'm not following.
or you're trying to have the User issue a notification to a Student, so they see it when they log in?
Well, here's a quick sketch of what it's like.
It's a learning website, so the way it works is that the User creates an account and signs in. We have an authenticated User, we can see the User's notifications, no problem.
The User, on the home page, sees the option to either select a Student (and thus add a Student to their session), or browse for courses to purchase for a Student, or view grades for all their Students. It's not truly "logging in as" Student even though it very much looks like it, because all Students under that account have the same User. However, the Students should have seperate notifications than the User (because the User wants to get notified about "Payment Declined," that kind of thing, whereas Students should have seperate "Course starting in 10 minutes" warnings.)
The notification panel is in the header. Right now, any notifications sent to
App\Models\User
get displayed, no problem. I can send notifications to App\Models\Student
like you see in the table, but I can't tell @livewire('notifications')
to show that model instead. I wish I could do something like @livewire('notifications', ['model' => Student::currentStudent()]
or @livewire('notifications', ['model' => auth()->user()])
or similar.
@awcodes Does that help at all? Or at least the last paragraph?right, but why would User see notifications for Student? that's what isn't clicking in my head.
if the Student was the logged in user, they would see those notifications.
Here is some UI if this helps:
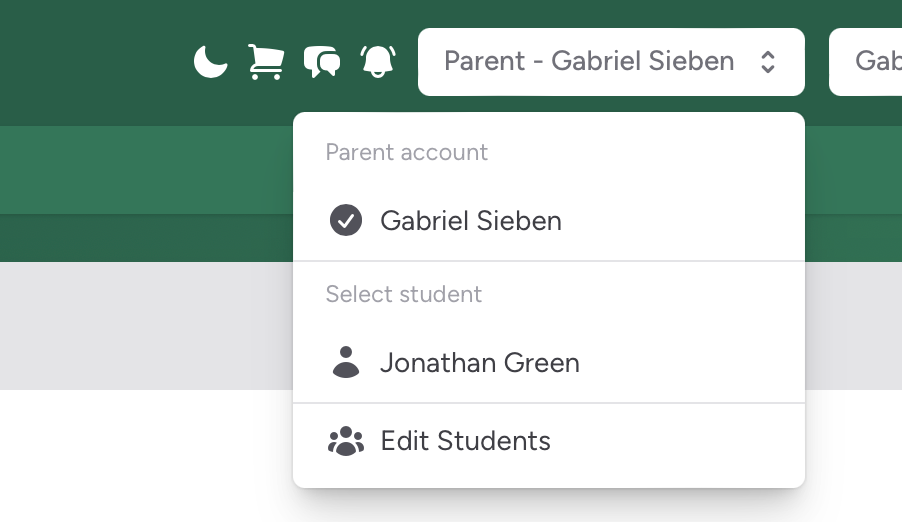
I am logged in as the same "User" model no matter the student I select. The "parent account" is just... not having a Student active.
yea, but Gabriel isn't going to get notifications for Jonathan
I don't want him to, in this case. I don't want Jonathan to see notifications for Gabriel.
other way around, is what i'm getting at
only Jonathan will see notifications if he is logged in
That's the goal. Right now Filament has no idea, because everyone is the same User account and has the same username/password, that anything has changed even though my
Student::currentStudent()
or whatever is changing. Every student, and parent, gets the same notification panel.
And my database notifications (in my prior screenshot) to App\Models\Student
never show up anywhere.Sorry, i'm just not following. Hopefully, someone else can assist further.
@awcodes Scrap the above then; here's another analogy that might make sense. Imagine if I have teams. I can be in multiple teams, and I can move between teams. There is such a thing as "team notifications" that I might want to send to a whole team. With the Filament panel, I the user could get notifications, but I couldn't display team notifications because the filament panel only shows User model notifications.
In that particular case you would get all the team members and send the notification to all of them. when the member logs in they will see it.
Perhaps... ugh. I wish...
OK, actually, here's probably the simplest way I can phrase my problem (sorry for any confusion): Imagine if I renamed the User model in my app to
NinjaWarrior
or something funny like that. How would I tell Filament to use my NinjaWarrior
model?
How can I invoke @livewire('notifications')
and have it refer to NinjaWarrior
instead of User? I've done all the authentication myself, it should be as easy as @livewire('notifications', ['model' => auth()->ninjawarrior()]
, ideally... but that doesn't work. What would I do?but NinjaWarrior is a user, so in the database there's a notification for NinjaWarrior model with an id of say 2. When that NinjaWarrior with that id of 2 logs in they will get that notification.
you're persisting the correct model to the table
laravel will just only show that notification to that logged in person.
Yes, it's in the notifications table. It would look almost like this:

But imagine if I completely ripped out auth()->user() because, I deleted the User model. Hypothetically.
How would I tell filament, reroute to use auth()->ninjawarrior(), for notifications?
i don't think you can or need to
is NinjaWarrior extending User or Authenticatable
Well, let's imagine it was Authenticatable
basically you have to be logged in to see notifications
or subscribed to a channel with broadcasting, but even that still requires a logged in person
i mean it's kind of a safety thing to keep notifications from leaking to the wrong people.
Would it work for you, later today, if I made a very simple demo app, using Filament, that demonstrated the situtation?
And open-sourced it on Github of course
sure, if you set up a reproduction repo i'm happy to look at it. it might be tomorrow before i can look at it though.
No problem; thank you for any help. 👍
no worries
please set it up with sqlite too please, so i don't have to worry about setting up a db. 🙂
Can’t you simply filter the notifications relation for each type on their model? That’s how I did it. So student has relationship with ->where(‘notifiable_type, ‘student’)