Compiler doesn't work properly
So i'm following the Microsoft course for beginners with C#.
The assignment is letting the console show this message
"Hello, Bob! You have 3 messages in your inbox. The temperature is 34.4 celsius."
This is my code, when I try to run it the online course keeps processing and doesn't proceed.
When I check my code in VS code it shows no errors, any help would be appreciated.
20 Replies
First, that's a very unusual style of variable initialisation. You don't need the parentheses there.
Second, those 4 Console.Write could and should be replaced with a single WriteLine with string interpolation
Sorry i’m not sure what you mean with String interpolation…
Bring up your favorite search engine and search "C# string interpolation"
Sure will do, sorry for that I could have thought of that myself 😅
Uhm...
Pobiega said it all.
There doesnt seem to be much actualy wrong with your code. it should execute.
most probable cause is, that the validator expects an new-line in the end
so in your last statement, just use WriteLine instead of Write
There will be no space between the temperature and word
celsius
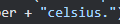
The message will be
Hello, Bob!You have3messages in your inboxThe temperature is 34.4celsius
That's why string interpolation is the wayThanks everyone for the feedback 🙂
String interpolation if I understood correctly 🙂
You still don't need the parentheses when declaring your variables 🙂
that, and also, consider using
WriteLine
instead, so there is a newline added at the end
and that blank space at the end triggers meHow is this ?, Removed the blank space at the end as mentioned by @Pobiega and the parentheses with the variable declaration @SinFluxx
perfect
Awesome
Thanks
In my variable declaration is the added m required since i'm already declaring a decimal type ?
yes
the right side is not aware of whats happening on the left. and
M
is the decimal suffix for a literalGood to know
So the declaration of the decimal type happens twice, left for the human readability and on the right for the compiler to process it correctly ?
its not different from how your other variables are declared
"Bob"
is a string literal.
3
is an int literal
34.4M
is a decimal literal
you could replace the types on the left with var
in this code
it would change nothingBecause var inherits the data type from which is assigned to ?
Infers
But yes
Infers , all right noted 🙂