✅ Any Frontenders? pagination
Hi, so i was training in building pagination, thats what i got - works well,
so, my problem is, i have no clue how to... can't even say what i need, like
in this case i have 5 buttons cuz -
so, what should i do for render only 3 pages, and like when on page 1 i click page 3, it render 2 - 3 - 4?
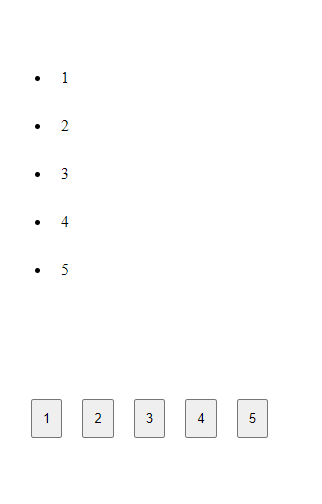
13 Replies
So, if its something super complicated for js without advanced frameworks, i prefer to keep it this way
or maybe i need to handle it in controller? like don't send page amount, just send specific pages i want to render?, like make start with 1-2-3 always, and on clicking for examle 3, send ((3-1) (3) (3+1) ?
and handle edge cases by if's?
are you asking if you want server-side pagination?
will it require to refresh my page all the time?
like, when i get to models and views, i thoght it will be super easy to do pagination
but its kida sucks to refresh page all the time
and a back to js options
unless you implement some kind of page cache in your client, yes
atm, i think im on the right way
if your intent is to load ALL records at once, and paginate them just for display, I woild propose that the goal you're trying to achieve is a better user experience for viewing a lot of records, and pagination is rather poor for that goal
if your intent is to minimize the amount of data being pulled from the server to client, pagination is a fine solution, and having repeat queries to the server to retrieve more data is actually what you WANT
@V.EINA Jaken
oh wtf
is there a question somewhere in there?
no, just solved and sharing i did it 🙂
so, will save code here
and close ticket 🙂
gg