❔ Property vs field?
Hi everyone,
I have a really basic but also really important question regarding C#. I have been coding for some time now, and I am using properties and fields as I have been instructed to in my classes, but still I don´t really grasp the difference. What is actually the difference? been reading online but these explanations don´t seem to do it for me :/
27 Replies
Rule of thumb: private fields, public properties
Properties allow for better encapsulation
Using them means the underlying field is never accessed directly, they create a layer of insulation and protect from unforseen results of API changes
yeah, but what does it actually mean? like I understand the basic concept of encapsulation, but I think I would need a practical example, if thats possible?
like, what could be a real-life consequence of not having proper encapsulation when needed?
For example, if you suddenly need to validate that a certain field is no less than 10, you would need to change all
object.Field = value
into object.SetField(value)
With properties it's not an issue, you just put the validation code in the setter
And object.Prop = value
remains object.Prop = value
, but now is validated
It doesn't break the code that calls it
Properties are a shortcut for getters and setters you might know from lesser languages like Java or C++
$getsetdevolvecan be shortened to
can be shortened to
can be shortened to
can be shortened to
Properties also allow for more granular access control
A
public
field will be public, in it's entirety
A property can be public but have a private setter
A property can also have an initializer instead of a setter, making it immutable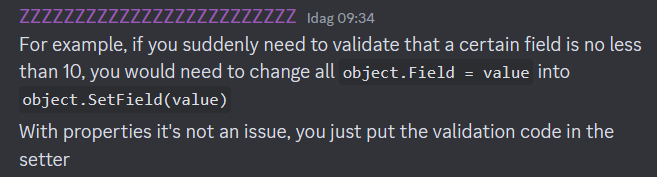
this part I dont fully understand
could you perhaps give me an example with the validation above? 🙂 🙌☺️
For example this
If you try to set age lower than 0 or higher than 150, it will throw an exception
Or this,
which will automatically truncate the value to 15 characters
private int _age;
public int Age {
get => _age;
set => _age = value is > 0 and < 150
? value
: throw new ArgumentException();
}
so this one.. the error would be prevented with a property?
The property allows validation, while a field does not
No, the error would be thrown with this property
Not prevented
Thing is, you cannot say "this field should be in range between 0 and 150, otherwise it's an error"
But you can say "this property should be in range between 0 and 150, otherwise it's an error"
soo a field should merely tell the programme which variables we want to use, and a property allows us to modify those variables?
Fields are the internal state of the object
Properties allow us to look at them (if allowed) and modify them (if allowed)
I think Im getting it
because fields should pretty much always be private?
yes
Yep
Angius
Rule of thumb: private fields, public properties
Quoted by
<@85903769203642368> from #Property vs field? (click here)
React with ❌ to remove this embed.
otherwise you are allowing unfiltered access to it from anywhere, and that access can do whatever it wants to the value.
setting it to 0, setting it to
int.MaxValue
etc
and this might break your domain rules for this object. thats why properties exist, so you can codify those rules
and be sureso the error thrown when we try to set the age to -5 is actually a thing we WANT to do? I think Im automatically thinking that errors is a bad thing
so properties are almost another way of saying "validation method"?
they are input validation methods to make sure that the objects values are reasonable
Yes, we want to throw the error
the error prevents -5 from being set. if you are writing it in code, you want the error so you can see "ah wait, im breaking my own rules here!"
thank you!! I finally understand it 🙂
and if you are getting the value from user input, you want the error (but catching it) so you can tell the user "you tried to set an invalid value!"
As a side note and an interesting piece of trivia, there are languages out there who have validation as a part of their type system. Can't remember their names or the specific syntax, but you can use them to do, say
F# allows this more or less
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.