uint value comparison for a 32 layer system (GameDev)
Hello. I'm looking for a way to see if these toggles are on or off by comparing the total value of the uint.
Description
How it works is that if the toggle is on, it returns the corresponding uint value and if it is off it returns zero. If we take top row for example, it goes from and index of 31 backwards to an index of 1. All these values that the toggles represents get added on top of each other and that results in the actual uint value. Taking the toggles on the top row as an example again, having them all toggled on it return
uint.MaxValue
and if they are all toggled off it returns 0
.
Example
Lets take 5 toggles each respresenting 1, 2, 4, 8 and 16 respectively.
if all toggles are on it should give back the total number of all the numbers the toggle represents. If all toggles are off it should return zero. If the first toggle is on and the rest are off it should return 1. If the first and the third toggle (1 and 4) are active it should return a total number of 5.
What I Did
What I did now was to create a switch and check every possible number, but I dont think it is a smart way to do this. it was the only way I knew that would work;
What I am hoping for
I was looking for a better/more elegant way to approach this problem. If anyone can point me in a direction, I'll be grateful. Thanks for reading.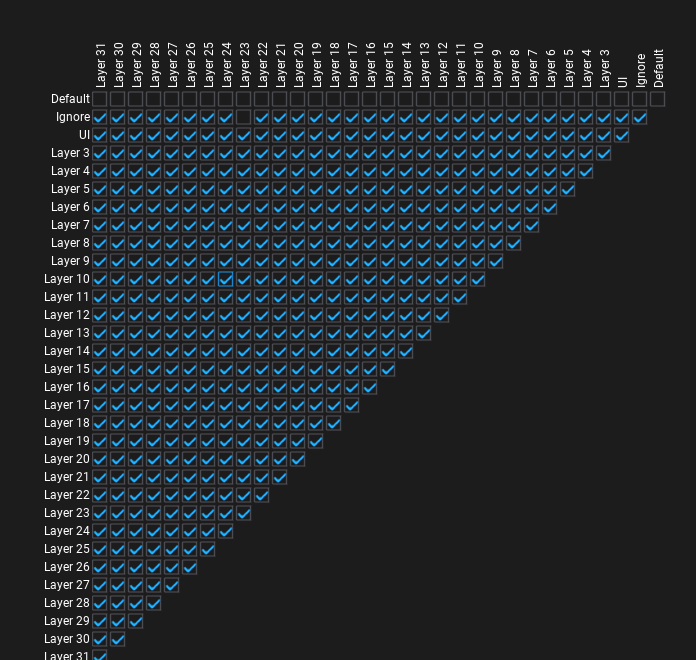
5 Replies
You're using a bitmask, basically
Convert the number to binary, and the positions of 1s will tell you which switches are turned on
I think I get it theoretically;
uint max being; 11111111 11111111 11111111 11111111
0 being; 00000000 00000000 00000000 00000000
1 being 00000000 00000000 00000000 00000001
but I'm still a little confused on how to do it practically. If you dont mind, could you give me a small example on how to do this?
bitwise and logical operators
its quite simple actually.
lets say u have ur toggles value as
1101
(binary) and u want to check the 3rd toggle, thats 0100
.
u simply need "and" both values and check if its not zero
1101 & 0100 == 0100
(3rd toggle)
1101 & 0010 == 0000
(2nd toggle)
to get the correct value from an index u need to bit shift 0001
by the index (remember that indices start at 0)
so its
1101 & (1 << 2) == 0100
(3rd toggle)
1101 & (1 << 1) == 0000
(2nd toggle)
so the method for checking a specific toggle is just
it can be done similar to check for any of a given set of indices to check at once.
for that u simply "or" the indices u want to check for:
uint toggle2and3 = (1 << 2) | (1 << 1);
which would be 0110
for any its the same comparison as above
1101 & 0110
results in 0100
which is not zero and thus there was any match
to check if exactly all given toggles are are set u would compare the "and" result with the togglesToCheck
(toggles & togglesToCheck) == togglesToCheck
if u want to check if all given (and maybe more) are set u would check if its greater or equal
(toggles & togglesToCheck) >= togglesToCheck
Got it figured out. The explanations made it crystal clear for me. Thank you everyone for taking the time helping me out! Super appreciated