Json object aggregate
In a multilingual app, I am using relational queries (but I could also just work with normal
select
s) to retrieve rows and their related translation strings. My schema has a bunch of tables like so:
I'm looking for a query that would return the data formatted as:
but I'm struggling to get anything else than:
10 Replies
Right now I'm using a reduce helper in to map the query data in js, but I'd rather use sql (
json_object_agg()?
) for the final aggregation formatting.I wrote an utility function for json aggregation
It may look horrible, but it works :D
Here's an example
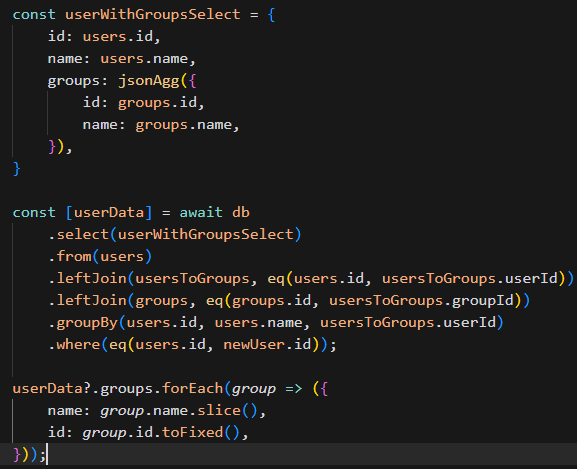
oh, sorry, I misunderstood the question. :D
No worries, it's still useful for me to just look at this code!
I can give u solution, but u need to use typescript instead of sql query ;d
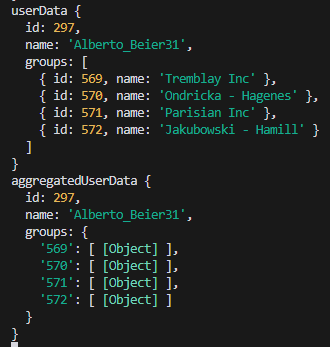
Yeah, I already have a ts solution but I'd rather have postgres run the aggregation :/
Here's a sample of
sql
query i'm essentially trying to translate to drizzle:
This appears to work:
I'll prolly make it into a more generic / reusable helper for my schemas.
Ended up being easier than i thought (hope i'm not forgetting something major). For posterity, here's the helpers I made basing myself on your code and a few other examples found across discord threads and gh issues:
That solves my need if used as:
Personally, I think official helpers that implement this in a better way should be part of drizzleAre u sure that if the translations are empty u won't get a null object?
I figure I'd get something like this. Unless I'm mistaken, thats what I want.
ah, sorry, yeap.
anyway gj :D