Custom getTableQuery() shows bad information after create record.
Hello, I have a custom query on ListPage to make groupBy and sum() instructions on the table.
After create a record shows bad information but after refresh page it shows correctly
Thanks in advance!
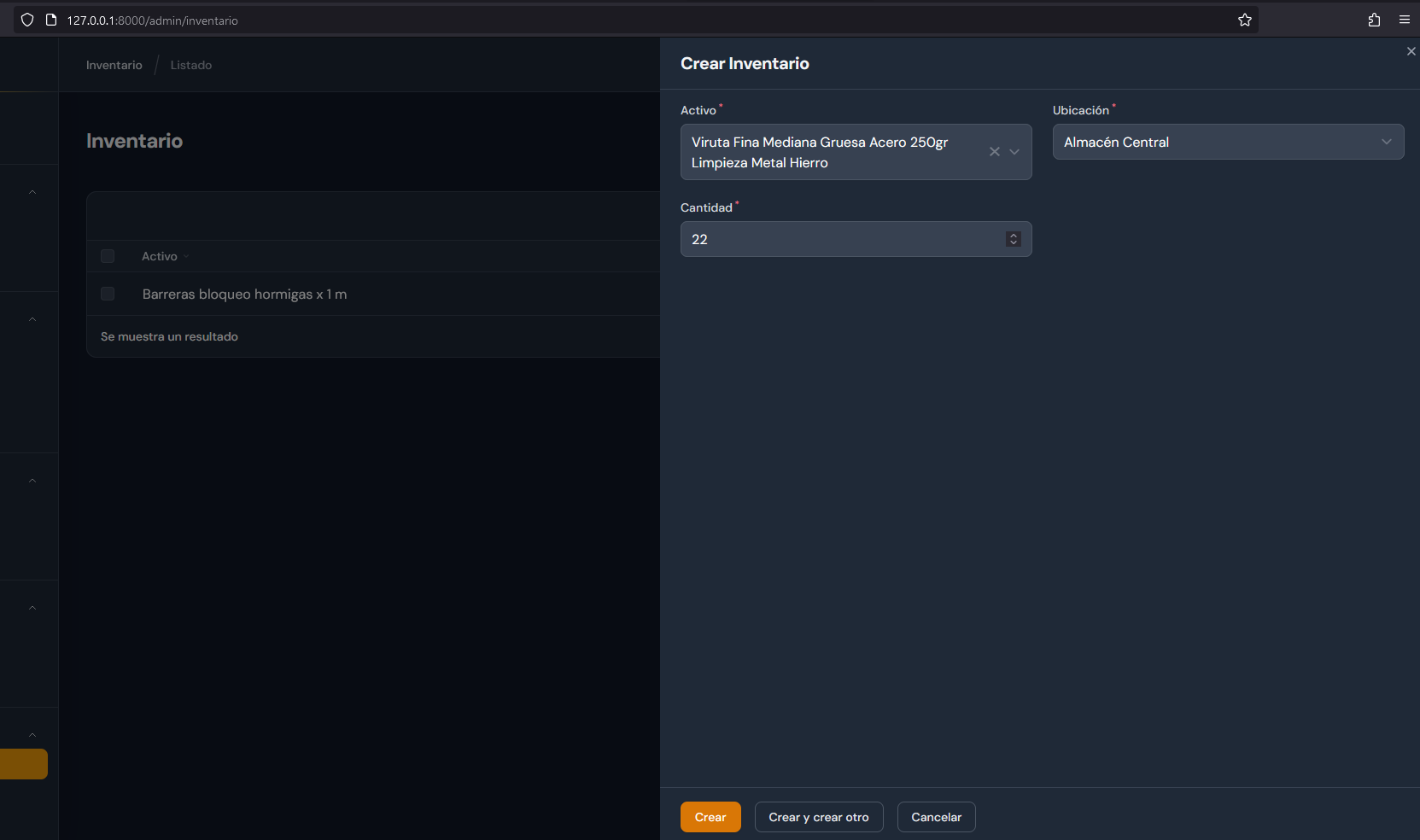
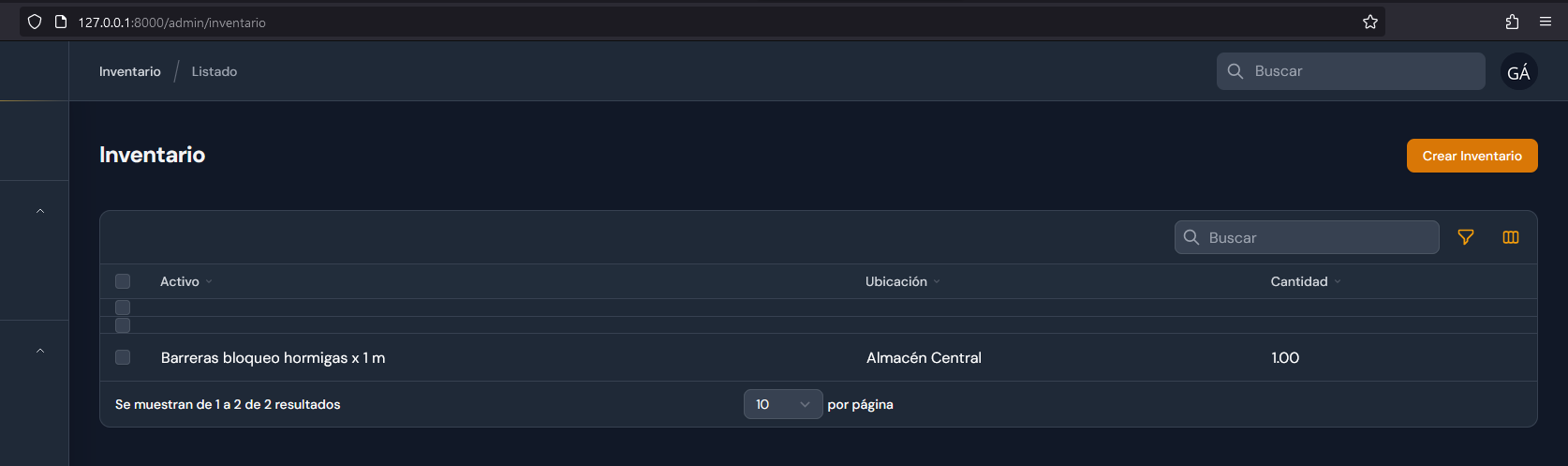
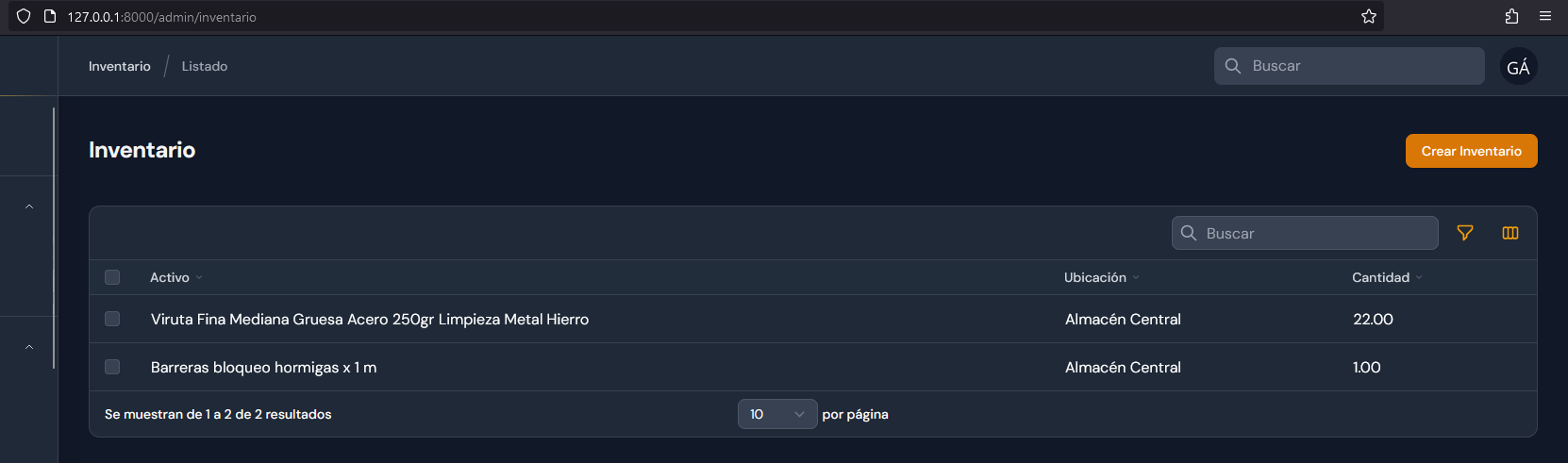
6 Replies
This just seems like the wrong approach. Feels like you should be using Eloquent's built in aggregate functionality.
I'm also suspicious of using a random() as the 'id'. And feels like you'll end up with multiple rows for each Inventory.
Thanks Hugh, I changed the query for the following and it works (until the moment):
Is there a specific reason you can use the built in aggregate functions for summing relations?
Hello @cheesegrits , no. How do you suggest I make the query? Thank you!
Well, I don't know exactly what you are trying to achieve, but Filament supports Laravel's relationship aggregation queries.
So in this example, I have a relationship called flightLegs, and I want to sum the total of the hobbs_flight_time field from that related table on each row of my main table.
As per the Filament docs, this just requires naming your aggregate field as <table_name>_sum_<field_name> (as per Laravel documentation), and then adding the sum(<relationship name>, <field_name>) method for FIlament. No additional query modifications required.
I just don't know if this provides what you need to do, as I can't really tell from your code exactly what it is you are trying to do.
https://filamentphp.com/docs/3.x/tables/columns/relationships#aggregating-relationships