❔ ✅ Why does this block the UI thread?
I have this function. Calling this seems to be blocking the thread that it's called from.
Did I do something wrong here, or should this not block and maybe something else is causing that?
56 Replies
What I want to accomplish is waiting for a window to exist, and then call the
handleWindowFind
callbackthere is lot of synchronous aka blocking code in this function. pretty much everything before your Task.Run()
and after
.. but that's not notable
right
how did you note that this blockgs the thread its called from?
I noticed the stutter the moment this gets called
Which is the exact time before for the window is created
what does the synchronous handleWindowFind() do?
It's a delegate parameter
yeah I know
but the function/-s stored in that delegate, what do they do?
they are blocking
It creates a new object that only assigns one value and does nothing more
mhm okay
how are you calling FindWindowHandle()?
can you show pls
playerManager.FindWindowHandle((IntPtr handle) => UnityHandleReady(new HandleRef(this, handle)));
just like a normal functionso its not an event handler, aight
why is it async void?
should be async Task
what's the diff
I don't want to await it cuz it's a callback
async void is bad and evil and you never do it, only exception event handlers
this is why its blocking tho
Ah okie
a fucntion dooesnt run asynchronouhslly just because of
async
. Its the await keyword that makes a func run async
so have it return task and await itbut uh
wait so that await won't block?
await is what makes code run asynchronously
you are running this function synchronously right now
hm allright
aka blocking
Coming from Java I had the idea that await would do the same as for example thread.join
there is no equivalent in Java
cuz it feels like await means 'wait until this is done'
most similar thing would be the Future lib in java
CompletableFuture is somewhat comparable to a Task in c#
yeah fair, but then this is without the endless lambda's xD
allright lemme test rq
So it doesn't let me await
cuz I'm calling the function from a UI thread function
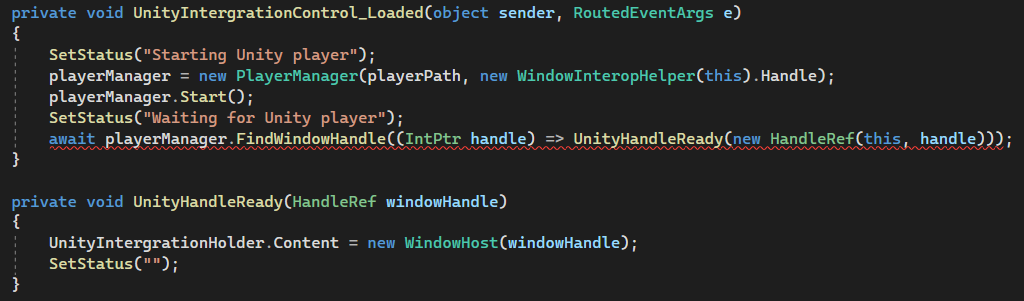
Calling the function when a WPF control is loaded
nop, it doesn't let you await because the method is not declared
async
oh wait so I don't give this the Task return?
cuz it's a event
but do give it async
correcct,
UnityIngetragtion....
should be async void because its an event handlerhmm
aight clear, that seems to work. Thank you!
glad I could help
no
I get it now ^-^
await means
come back when this task finishes
I suggest reading on TPL (Task-Parallel library), especially on TAP section (Task-based-asynchronous Pattern)
https://learn.microsoft.com/en-us/dotnet/standard/asynchronous-programming-patterns/task-based-asynchronous-pattern-tap
Task-based Asynchronous Pattern (TAP): Introduction and overview - ...
Learn about the Task-based Asynchronous Pattern (TAP), and compare it to the legacy patterns: Asynchronous Programming Model (APM) and Event-based Asynchronous Pattern (EAP).
yeah, it's weird cuz this makes the lines of code not ordered
they are ordered
thats the point of await
what cyb is saying
that you dont have to schedule continuation tasks
No because the UI thread is executing lines while it's waiting
like
task.then(...).then(...)
isnt neededthe ppoint of await is to run code asnychronously but in a "synchronous fashion" which means in order
but not blocking
wdym
I get that your own code is executed in order
I mean that you could have statement 1 2 3 of your code executed and then have an await
then a couple lines of UI thread code get executed
and the await finishes and the next lines of your code get executed
exactly
So it's not entirely 'line by line'
which feels odd if you don't know how it works
but yeah
yeah, but in the context of the method it is
yup
everything does :)
ty for the help I gotta catch a bus ^-^
have a nice day!
np you too :)
I will point this out again just to make sure it doesnt get forgotten
if your issue has been solved, dont forget to close 🙂
Will check out the links.
oh and if you're interested in how the treads get joined together when using asynchronous code, a Task stores a reference to the calling thread and continuations, which are the code that follows an
await someTask
call or code thats put in someTask.ContinueWith()
. when it reaches Completed state it enqeues the continuations on the calling threadWas this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.