✅ why are they considered int instead of string?
So I was working on this puzzle https://leetcode.com/problems/backspace-string-compare/submissions/ I would like some advice on the compiler error/tipo (not the semantic/logical error to resolve this puzzle). My code is as follows, I am getting error
CS1503: Argument 1: cannot convert from 'string' to 'int' (in Solution.cs)
for two string initialisations inside the foreach-loop at the bottom. I had tried ToString()/Convert.ToString(), but to no avail. Could anyone kindly point me in the right direction?
public class Solution {
public bool BackspaceCompare(string s, string t) {
var dic1 = new Dictionary<string, string>(){
{" ", "#"},
{" ", "##"},
{" ", "###"}
};
var dic2 = new Dictionary<string, string>(){
{" ", "#"},
{" ", "##"},
{" ", "###"}
};
for(int i = 0; i < s.Length; i++){
string sStr = s[i].ToString();
string tStr = t[i].ToString();
if(!dic1.ContainsKey(sStr)){
dic1.Add(sStr, " ");
}
if(!dic2.ContainsKey(tStr)){
dic2.Add(tStr, " ");
}
}
foreach(var k in dic1.Keys){
string sForEach = s[k].Convert.ToString(); //ERROR HERE
string tForEach = t[k].Convert.ToString(); //ALSO HERE
if(dic2.ContainsKey(k) && sForEach == tForEach){
return true;
}
}
return false;
}
}
LeetCode
LeetCode - The World's Leading Online Programming Learning Platform
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
8 Replies
In which line does this error happen?
Well, the keys of that dictionary are strings
So
k
is a string
And you can't index a string with a string
"hello"["unga"]
makes no sense after all
Also, I'm not sure a char
has a property .Convert
So, uh, did it help? Did it not? Did you write it while driving and had an accident and that's why you're silent?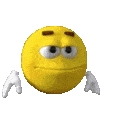
maybe he's thinking really hard 😒
Hi all, I was taking a 15min-break, not in front of computer. Ok I misunderstood the indexing and the working of the string
Yes, it helped me a lot ^^, let me try this - if I still have problems I let you all know here
A
string
is syntactic sugar over char[]
basicallyWas this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.