Automod System
I realized if I say 3 bad words fast enough it won't trigger the automod, why could this happend?
Code:
https://pastebin.com/cy6T1Re2
Pastebin
Pastebin.com - Locked Paste
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
33 Replies
Since event listeners are triggered concurrently, and you there is a lot of async functionality in the listener, you're in a situation where messages sent in quick succession might not be fully processed before the next one arrives. i.e.:
message 1 is detected
data 1 is fetched from the db
message 2 is detected
data 2 is fetched from the db
new data is written to the db from message 1 (not fast enough!)
new data is written to the db from message 2
The easiest solution, in my opinion, is to use 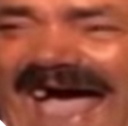
@sapphire/async-queue
, which will ensure that the messages are processed sequentially. Example:
One potential performance concern would be that this would process all messages sequentially when you really only care about processing an individual's messages sequentially (and doing this concurrently for all individuals). This could be solved by creating a different queue for each person (I'll leave that as an exercise to the reader 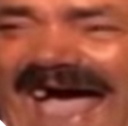
instead of
// ...
I put the rest of my code rightThe best way for that would probably be
Map<string, Queue>
, and just add a new entry for each user. As a form of cache invalidation, you can check before queue.shift()
if there are no other promises (queue.promises
), remove it from the map
Yes (although use your discretion)Got an error:
You didn't create the queue variable. As I said, use your discretion. Make sure you understand the library and how to use it (it's a small library, so reading the source code might be best).
GitHub
utilities/packages/async-queue/src/lib/AsyncQueue.ts at main · sapp...
Common JavaScript utilities for Sapphire Projects. Contribute to sapphiredev/utilities development by creating an account on GitHub.
It's more productive for you to learn how it works than for me to microedit your code
Added this, (not sure if it's correct), it doesn't log any error now, but it also still doesn't work
Please send the full code. Also,
automod
and automodMute
both return promises, so you have to await them if you want to use the queue effectively.
You also should await the message.channel.send
and message.delete
(Or, if you want to make it fast, use Promise.all
and resolve the promises all at once.)updated code:
https://pastebin.com/cy6T1Re2
Pastebin
Pastebin.com - Locked Paste
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
The queue should be defined outside of the listener so it can maintain state between function calls.
done
any way of making it delete the command instantly?
Delete the command?
message I meant
Order your promises consciously. If you want to delete the message before you check the DB, do that. If you want to do both at the same time, use Promise.all. if you want to register the infraction in the DB before sending the message, do that. If you want to do it at the same time, use Promise.all.
Order your promises considering your priorities
Maybe you want to delete the message while querying the DB while sending the confirmation message, then register the infraction in the DB, then unshift the queue
Often you want the visible things to happen first to give the illusion (whether true or false) of things being fast
In situations such as sending the confirmation message early, this is called an optimistic update.
Sorry that's a bunch of word jumble it's late lol
it's ok lol
if I do this I would also need to put my
if
to check if the message.content
contains a filtered word, which would not work in my command since I want it to check if the member has 3, 5, 7, 8 or 9 auto warnings firstThen no, you can't delete the message instantly 🤷
Maybe you could do some caching in the db?
Or, since the queue only needs to wait until you write to the db, try to write to the db and shift the queue as soon as possible
.
Like this?
I'm on my phone so I don't have the original code up, but probably
yeah just tried and it didn't work
Can you send the full code
Pastebin
Pastebin.com - Locked Paste
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
Should you be waiting until after automod() too?
wouldn't that just be the same as before
What do you mean
I meant my code was already like that
I don't remember your previous code well, but I think you sent a message before shifting that wasn't necessary for the queue to shift
i mean, the delete time is just like 1 second or less, so it doesn't really matter
however I just realized this:
If I member gets auto warned 3 times, gets auto timeouted, then I manually untimeout them, then that person say anything (anything, even if the word is not in the filter), it will timeout them like if they had 5 warnings
weird since
1. word wasn't on filter
2. the user would have 4 warnings, not 5
Is that because youre checking data.length + 1 instead of data.length?
earlier, when I was making this command, I was trying stuff like
if (data.length === 3) { do something }
before making await this.container.util.automodMute()
(and it didn't work), due to this I logged data.length and it said 0 so adding a + 1 fixed itYou should probably figure out what the original problem was, because your solution might've created another one
what really confuses me is that when I manually untimeout them, and then the user says anything it timeouts them for 4 hours, which is the 5 warns punishment
it doesn't add the warn to the db but it adds the timeout
it also doesn't send the
.send()
partanyone has any idea why could this happend?
code:
https://pastebin.com/cy6T1Re2
Pastebin Password: TL8PytN6tj
Pastebin
Pastebin.com - Locked Paste
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.