How can I run Alpine.js code from php or blade?
I want to create a music player, and even though the plain alpine code works, I need to intergrade it in blade or php.
23 Replies
I think the main way to interact between Livewire and Alpine would be dispatching events... can you give a bit more context on what you're trying to do?
Yes so basically I want to create my own music player plugin for filament. Atm I want to see how I can make a single audio file play. And afterwards I will make a UI for it.
For example:
So the Action in this case wont be delete, but lets say play
When you press play, the music starts
Then I need to make another Action that pauses the music etc
I see! Thanks. So a few thoughts:
The
<audio>
tag will have its own set of controls... as far as I know it's not very styleable in CSS but you can style the container.
There are entire JS libraries to implement custom video/audio players, it's not a small task. But totally possible.
This is inevitably linked to browser permissions, so if a user doesn't give permissions to play audio/video, you custom button won't be able to start/stop the audio player.I see, thank you. But how can I handle the play/pause dispatch events?
But to answer your question more directly, I think I would dispatch a browser event from the Filament action, and catch it with an Alpine listener on the
<audio>
tag.This is how I approached it in just alpine:
I assume there is filament documentation for that, correct?
JS code looks fine at a glance, here's a rough sketch of what I'm thinking about:
This is more in the Livewire/Alpine side of things... I would look in the docs for that instead of Filament
I see. Thank you! 🙂
I will keep you updated
if the alpine works, why can't you just put that code in the blade file. Alpine is already loaded
I tried but for some reason it doesnt play:
Im almost sure I havent configured blade properly though
change the 'body' tags to 'div' you can't nest body tags
Yeah, I just tried and it doesnt work. No audio
try this not sure why your control functions are outside the alpine component?
This didnt work either
I think im getting closer.
This works
At least it loads the song
However when trying to upload to the storage, I got errors. I had to manually copy paste it. Any idea why?
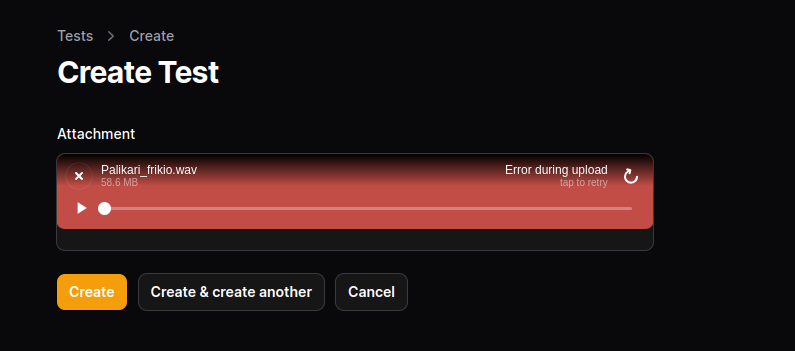
Possibly because of the temporary storage... you'll need to save the form (or at least trigger validation) for the file to be moved to the final storage location.
I doubt it. It works fine for other files like svg, png etc
Ok. Any errors in the browser console or
storage/logs/laravel.log
?
I exceeded the default limit?
Php upload limit probably
in
php.ini
Yes you were right. I just went to the ini file and edited the default values. Thanks:)